ios动画
2015-09-16 21:12
141 查看
一、动画的使用场景
iOS中的动画是指⼀些视图上的过渡效果,合理利⽤动画能提⾼⽤户体验。
动画的分类

二、UIView动画
2.1 属性:
frame:视图框架
center:视图位置
bounds:视图⼤⼩
backgroundColor:背景颜⾊
alpha:视图透明度
transform:视图转换
2.2 UIView动画的设置
⽅法名 作⽤
+ (void)setAnimationDuration:(NSTimeInterval)duration; 动画持续时间
+ (void)setAnimationDelay:(NSTimeInterval)delay; 动画开始前延时
+ (void)setAnimationCurve:(UIViewAnimationCurve)curve; 动画的速度曲线
+ (void)setAnimationRepeatAutoreverses:(BOOL)repeatAutoreverses; 动画反转
+ (void)setAnimationRepeatCount:(float)repeatCount; 动画反转的次数
+ (void)setAnimationDelegate:(id)delegate; 设置动画的代理
+ (void)setAnimationWillStartSelector:(SEL)selector; 动画开始的代理⽅法
+ (void)setAnimationDidStopSelector:(SEL)selector; 动画结束的代理⽅法
+ (void)setAnimationBeginsFromCurrentState:(BOOL)fromCurrentState; 动画从当前状态继续执⾏
三、CGAffineTransform2D仿射变换
CGAffineTransform是结构体,表⽰⼀个矩阵,⽤于映射视图变 换。 缩放、旋转、偏移是仿射变换⽀持的最常⽤的操作。 缩放、缩放、偏移区分“基于上⼀次”和“基于初始” 。
3.1 CGAffineTransfrom的API
CGAffineTransformMake(CGFloat a, CGFloat b, CGFloat c, CGFloat d,CGFloat tx, CGFloat ty) 通过设置矩阵值进⾏变换
CGAffineTransform CGAffineTransformMakeScale(CGFloat sx, CGFloat sy) 放⼤缩⼩(基于初始)
CGAffineTransform CGAffineTransformMakeRotation(CGFloat angle) 旋转(基于初始)
CGAffineTransform CGAffineTransformScale(CGAffineTransform t,CGFloat sx, CGFloat sy) 放⼤缩⼩(基于前⼀次变化)
CGAffineTransform CGAffineTransformRotate(CGAffineTransform t,CGFloat angle) 旋转(基于前⼀次变化)
3.2 Demo
#pragma mark -- 首尾动画 --
/*
语法形式:
[UIView beginAnimations:动画名 context:传给代理所执行的方法后面的参数];
[UIView commitAnimations];
*/
- (IBAction)didClickFirstBtn:(UIButton *)sender {
[UIView beginAnimations:@"第一个UIView动画" context:nil];
//动画和视图的属性设置
//1.设置动画的执行时间
[UIView setAnimationDuration:.1];
//2.设置动画重复次数
[UIView setAnimationRepeatCount:2];
[UIView setAnimationCurve:UIViewAnimationCurveLinear];
//从当前状态执行
[UIView setAnimationBeginsFromCurrentState:YES];
// [UIView setAnimationRepeatAutoreverses:YES];
//设置视图相关属性
// CGRect frame = self.view.frame;
// frame.size.width /= 50;
// frame.size.height /= 50;
// self.myView.frame = frame;
self.myView.transform = CGAffineTransformRotate(self.myView.transform, M_PI);
// self.myView.transform = CGAffineTransformMakeScale(0.5, 0.5);
self.myView.transform = CGAffineTransformScale(self.myView.transform, 2, 2);
[UIView commitAnimations];
}
#pragma mark -- BLOCK动画块 --
- (IBAction)didClickSecondBtn:(id)sender {
__block ViewController *VC = self;
[UIView animateWithDuration:0.5 animations:^{
//对动画和视图属性进行设置
// [UIView setAnimationBeginsFromCurrentState:YES];
[UIView setAnimationRepeatCount:1.0];
//视图设置
VC.myView.transform = CGAffineTransformRotate(VC.myView.transform, M_PI_4);
VC.myView.backgroundColor = [UIColor colorWithRed:arc4random()%256/255.0 green:arc4random()%256/255.0 blue:arc4random()%256/255.0 alpha:1];
} completion:^(BOOL finished) {
//动画结束之后
[UIView animateWithDuration:0.5 animations:^{
[UIView setAnimationRepeatCount:1];
VC.myView.transform = CGAffineTransformScale(VC.myView.transform, 0.5, 0.5);
} completion:^(BOOL finished) {
}];
}];
}
#pragma mark -- UIView的转场动画 --
- (IBAction)transitionAnimation:(id)sender {
//1.view自身的转场动画
// [UIView transitionWithView:self.myView duration:1 options:UIViewAnimationOptionTransitionCurlUp animations:^{
// [UIView setAnimationRepeatCount:2];
// self.myView.backgroundColor = [UIColor redColor];
// } completion:^(BOOL finished) {
//
// }];
//2.两个view之间的转场动画
UIView *toView = [[UIView alloc] initWithFrame:CGRectMake(100, 100, 100, 100)];
toView.backgroundColor = [UIColor cyanColor];
[UIView transitionFromView:_myView toView:toView duration:1.0 options:UIViewAnimationOptionTransitionCrossDissolve completion:nil];
//将self.myView从父视图上移除(销毁),同时添加toView
}
四、CALayer
4.1 UIView和CALayer的区别和联系
CALayer负责绘制,提供UIView 需要展⽰的内容。不能交互。是UIView的⼀个readonly属性。
UIView负责交互,显⽰CALayer绘制的内容。
4.2 CALayer的常⽤属性
属性 作⽤
CornerRadius 圆⾓
ShadowColor 阴影颜⾊
ShadowOffset 阴影偏移距离
ShadowRadius 阴影模糊程度
ShadowOpacity 阴影透明度
BorderWidth 描边粗细
BorderColor 描边颜⾊
anchorPoint 锚点
position 位置信息
transfrom 使CALayer产⽣3D空间内的平移、缩放、旋转等变化
4.3 anchorPoint和position
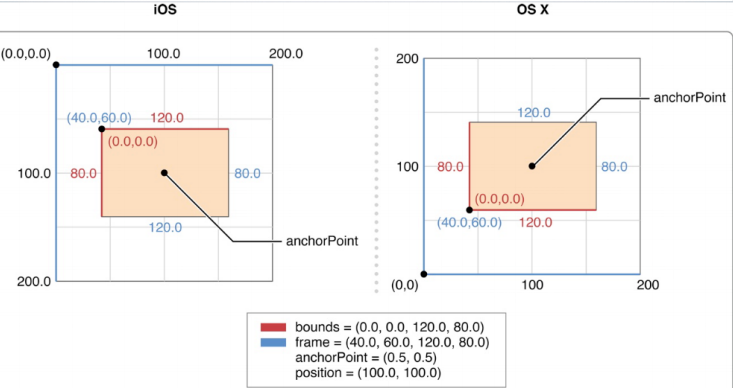
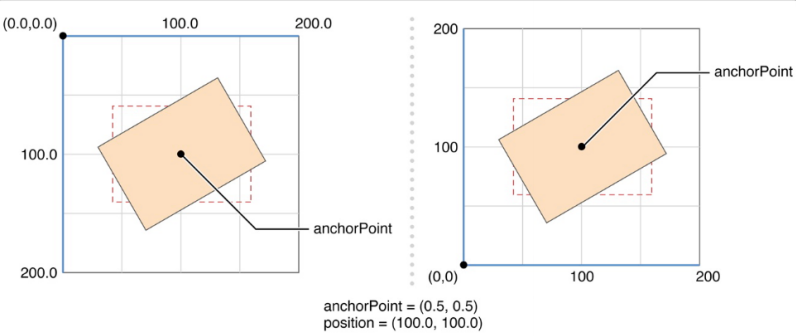
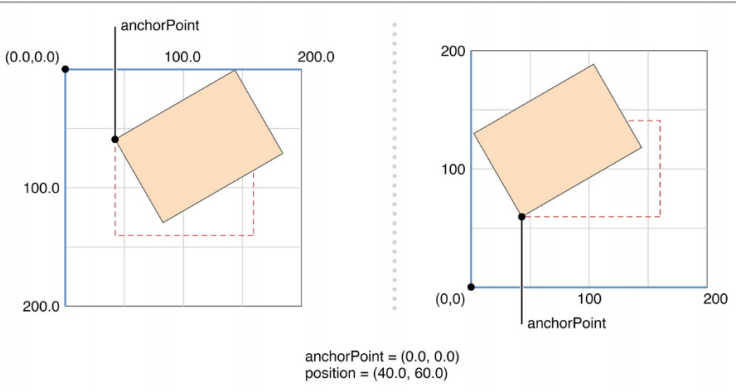
4.4 Demo
- (void)viewDidLoad {
[super viewDidLoad];
_imgView.backgroundColor = [UIColor redColor];
_imgView.layer.cornerRadius = 30;
// _imgView.layer.masksToBounds = YES; //如果设置masksToBounds=YES,设置阴影无效
_imgView.layer.anchorPoint = CGPointMake(0.5, 1);
_imgView.layer.shadowColor = [UIColor lightGrayColor].CGColor;
_imgView.layer.shadowOpacity = 1;
_imgView.layer.shadowOffset = CGSizeMake(10, 10);
_imgView.layer.shadowRadius = 1;
_myView.layer.cornerRadius = 50;
_myView.layer.shadowColor = [UIColor redColor].CGColor;
_myView.layer.shadowOpacity = 1;
_myView.layer.shadowOffset = CGSizeMake(10, 10);
#pragma mark -- 自定义CALayer --
[self customLayer];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)customLayer
{
CALayer *myLayer = [CALayer layer];
//设置其位置和大小
myLayer.bounds = CGRectMake(0, 0, 50, 50);
myLayer.backgroundColor = [UIColor yellowColor].CGColor;
//设置锚点
myLayer.anchorPoint = CGPointMake(0, 0);
// 设置position(定位点,默认是(0,0)).锚点和position始终重合
myLayer.position = CGPointMake(50, 50);
//添加到父图层
[self.view.layer addSublayer:myLayer];
}
- (IBAction)didClickBasic:(id)sender {
/*
//1.设置动画对象
CABasicAnimation *basicAnimation = [CABasicAnimation animation];
//2.告诉系统要执行的动画,影响的是哪个属性
basicAnimation.keyPath = @"position";
//3.设置通过动画,将layer从哪移动到哪
basicAnimation.fromValue = [NSValue valueWithCGPoint:CGPointMake(100, 100)];
basicAnimation.toValue = [NSValue valueWithCGPoint:CGPointMake(200, 500)];
//取消回到原位置
basicAnimation.removedOnCompletion = NO;
//设置保存动画的最新状态
basicAnimation.fillMode = kCAFillModeForwards;
basicAnimation.duration = 3.0;
[self.imgView.layer addAnimation:basicAnimation forKey:@"basic"];
*/
//实现旋转
CABasicAnimation *basic = [CABasicAnimation animationWithKeyPath:@"transform"];
basic.duration = 2.0;
basic.toValue = [NSValue valueWithCATransform3D:CATransform3DMakeRotation(M_PI_4*4, 1, 1, 1)];
basic.removedOnCompletion = NO;
basic.fillMode = kCAFillModeForwards;
[self.imgView.layer addAnimation:basic forKey:@"basic"];
//移除动画
// [self.imgView.layer removeAnimationForKey:@"basic"];
}
#pragma mark -- IBActions -------
#pragma mark -- 帧动画 --
- (IBAction)didClickKeyFrame:(id)sender {
CAKeyframeAnimation *keyFrame = [CAKeyframeAnimation animationWithKeyPath:@"transform.rotation"];
//设置动画时间
keyFrame.duration = 0.1;
//设置imageView的抖动角度
//角度转换为弧度: 度数/180*M_PI
keyFrame.values = @[@(-4.0/180*M_PI),@(4.0/180*M_PI)];
keyFrame.repeatCount = 100;
[self.imgView.layer addAnimation:keyFrame forKey:@"keyFrame"];
}
#pragma mark -- CATransition转场动画 --
- (IBAction)didClickTransition:(id)sender {
//属性:
//type:设置动画过渡类型
//subType:动画过度的方向
//startProgress:动画起点
//endProgress:动画终点
self.index++;
self.index = _index%19 +1;
self.imgView.image = [UIImage imageNamed:[NSString stringWithFormat:@"image%d.jpg",self.index]];
CATransition *transition = [CATransition animation];
transition.type = @"cube";
transition.subtype = kCATransitionFromLeft;
transition.duration = 2;
transition.repeatCount = 10;
// transition.startProgress = 0;
// transition.endProgress = 1;
[self.imgView.layer addAnimation:transition forKey:@"transition"];
}
五、CAAnimation动画
CAAnimation是抽象类,通常使⽤它的⼦类实现动画效果。所有CAAnimation及其⼦类的对象都添加在View的layer上,例如: [view.layer addAnimation:animation forKey:nil];
5.1 给layer添加/移除CALayer动画
- (void)addAnimation:(CAAnimation *)anim forKey:(NSString *)key;
- (void)removeAnimationForKey:(NSString *)key;
- (void)removeAllAnimations;
5.2 CAAnimation相关⼦类
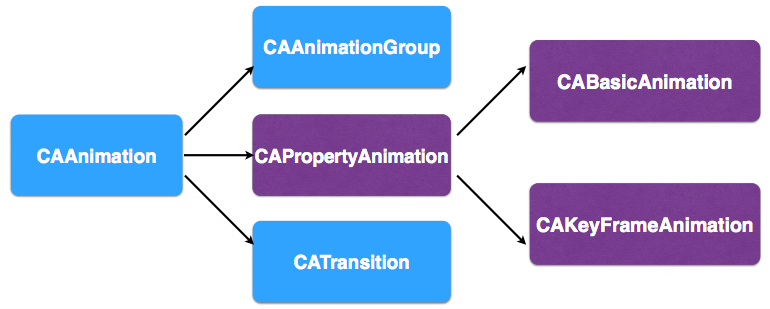
CAPropertyAnimation也是⼀个抽象类 通常我们都使⽤它的两个⼦类:CABasicAnimation和 CAKeyFrameAnimation
5.2.1 CABasicAnimation
作⽤:基本layer动画,通过设定初始和结束值执⾏动画
+ (id)animationWithKeyPath:(NSString *)path; 系统提供的构造器⽅法
@property(copy) NSString *keyPath; 只能填写CALayer中能够做
动画的属性名
@property(retain) id fromValue; 起始值
@property(retain) id toValue; 结束值
@property(retain) id byValue; 相对值
5.2.2 CAKeyFrameAnimation
作⽤:关键帧动画,可以让你的view的layer按照预定轨迹做动画
⽅法名/属性名 作⽤
+ (id)animationWithKeyPath:(NSString *)path; 系统提供的构造器⽅法
@property CGPathRef path; 通过制定⼀个⾃⼰定义的path来让某⼀个物体按照
这个路径进⾏动画
@property(copy) NSArray *values; ⼀个数组,提供了⼀组关键帧的值, 当使⽤path的
时候 values的值⾃动被忽略。
@property(copy) NSArray *keyTimes; ⼀个数组,设置每⼀帧的时间,其成员必须是
NSNumber。设置详情查看API。
@property(copy) NSString *rotationMode; 设定关键帧中间的值是如何计算
5.3 CAAnimaitionGroup
CAAnimationGroup只有⼀个数组属性,可以添加多个CAAnimation⼀起执⾏
5.3.1 CATrasition
作⽤:layer的过渡动画
有两个主要属性:type(设置过渡动画的效果)和subType(设置过渡动画的⽅向)
5.4 DEMO
- (IBAction)didGroupAnimation:(id)sender {
// 平移动画
CABasicAnimation *a1 = [CABasicAnimation animation];
a1.keyPath = @"transform.translation.y";
a1.toValue = @(100);
// 缩放动画
CABasicAnimation *a2 = [CABasicAnimation animation];
a2.keyPath = @"transform.scale";
a2.toValue = @(0.0);
// 旋转动画
CABasicAnimation *a3 = [CABasicAnimation animation];
a3.keyPath = @"transform.rotation";
a3.toValue = @(M_PI_2);
CAAnimationGroup *group = [CAAnimationGroup animation];
group.animations = @[a1, a2, a3];
group.fillMode = kCAFillModeForwards;
// group.removedOnCompletion = NO;
[self.imgView.layer addAnimation:group forKey:@"group"];
}
iOS中的动画是指⼀些视图上的过渡效果,合理利⽤动画能提⾼⽤户体验。
动画的分类

二、UIView动画
2.1 属性:
frame:视图框架
center:视图位置
bounds:视图⼤⼩
backgroundColor:背景颜⾊
alpha:视图透明度
transform:视图转换
2.2 UIView动画的设置
⽅法名 作⽤
+ (void)setAnimationDuration:(NSTimeInterval)duration; 动画持续时间
+ (void)setAnimationDelay:(NSTimeInterval)delay; 动画开始前延时
+ (void)setAnimationCurve:(UIViewAnimationCurve)curve; 动画的速度曲线
+ (void)setAnimationRepeatAutoreverses:(BOOL)repeatAutoreverses; 动画反转
+ (void)setAnimationRepeatCount:(float)repeatCount; 动画反转的次数
+ (void)setAnimationDelegate:(id)delegate; 设置动画的代理
+ (void)setAnimationWillStartSelector:(SEL)selector; 动画开始的代理⽅法
+ (void)setAnimationDidStopSelector:(SEL)selector; 动画结束的代理⽅法
+ (void)setAnimationBeginsFromCurrentState:(BOOL)fromCurrentState; 动画从当前状态继续执⾏
三、CGAffineTransform2D仿射变换
CGAffineTransform是结构体,表⽰⼀个矩阵,⽤于映射视图变 换。 缩放、旋转、偏移是仿射变换⽀持的最常⽤的操作。 缩放、缩放、偏移区分“基于上⼀次”和“基于初始” 。
3.1 CGAffineTransfrom的API
CGAffineTransformMake(CGFloat a, CGFloat b, CGFloat c, CGFloat d,CGFloat tx, CGFloat ty) 通过设置矩阵值进⾏变换
CGAffineTransform CGAffineTransformMakeScale(CGFloat sx, CGFloat sy) 放⼤缩⼩(基于初始)
CGAffineTransform CGAffineTransformMakeRotation(CGFloat angle) 旋转(基于初始)
CGAffineTransform CGAffineTransformScale(CGAffineTransform t,CGFloat sx, CGFloat sy) 放⼤缩⼩(基于前⼀次变化)
CGAffineTransform CGAffineTransformRotate(CGAffineTransform t,CGFloat angle) 旋转(基于前⼀次变化)
3.2 Demo
#pragma mark -- 首尾动画 --
/*
语法形式:
[UIView beginAnimations:动画名 context:传给代理所执行的方法后面的参数];
[UIView commitAnimations];
*/
- (IBAction)didClickFirstBtn:(UIButton *)sender {
[UIView beginAnimations:@"第一个UIView动画" context:nil];
//动画和视图的属性设置
//1.设置动画的执行时间
[UIView setAnimationDuration:.1];
//2.设置动画重复次数
[UIView setAnimationRepeatCount:2];
[UIView setAnimationCurve:UIViewAnimationCurveLinear];
//从当前状态执行
[UIView setAnimationBeginsFromCurrentState:YES];
// [UIView setAnimationRepeatAutoreverses:YES];
//设置视图相关属性
// CGRect frame = self.view.frame;
// frame.size.width /= 50;
// frame.size.height /= 50;
// self.myView.frame = frame;
self.myView.transform = CGAffineTransformRotate(self.myView.transform, M_PI);
// self.myView.transform = CGAffineTransformMakeScale(0.5, 0.5);
self.myView.transform = CGAffineTransformScale(self.myView.transform, 2, 2);
[UIView commitAnimations];
}
#pragma mark -- BLOCK动画块 --
- (IBAction)didClickSecondBtn:(id)sender {
__block ViewController *VC = self;
[UIView animateWithDuration:0.5 animations:^{
//对动画和视图属性进行设置
// [UIView setAnimationBeginsFromCurrentState:YES];
[UIView setAnimationRepeatCount:1.0];
//视图设置
VC.myView.transform = CGAffineTransformRotate(VC.myView.transform, M_PI_4);
VC.myView.backgroundColor = [UIColor colorWithRed:arc4random()%256/255.0 green:arc4random()%256/255.0 blue:arc4random()%256/255.0 alpha:1];
} completion:^(BOOL finished) {
//动画结束之后
[UIView animateWithDuration:0.5 animations:^{
[UIView setAnimationRepeatCount:1];
VC.myView.transform = CGAffineTransformScale(VC.myView.transform, 0.5, 0.5);
} completion:^(BOOL finished) {
}];
}];
}
#pragma mark -- UIView的转场动画 --
- (IBAction)transitionAnimation:(id)sender {
//1.view自身的转场动画
// [UIView transitionWithView:self.myView duration:1 options:UIViewAnimationOptionTransitionCurlUp animations:^{
// [UIView setAnimationRepeatCount:2];
// self.myView.backgroundColor = [UIColor redColor];
// } completion:^(BOOL finished) {
//
// }];
//2.两个view之间的转场动画
UIView *toView = [[UIView alloc] initWithFrame:CGRectMake(100, 100, 100, 100)];
toView.backgroundColor = [UIColor cyanColor];
[UIView transitionFromView:_myView toView:toView duration:1.0 options:UIViewAnimationOptionTransitionCrossDissolve completion:nil];
//将self.myView从父视图上移除(销毁),同时添加toView
}
四、CALayer
4.1 UIView和CALayer的区别和联系
CALayer负责绘制,提供UIView 需要展⽰的内容。不能交互。是UIView的⼀个readonly属性。
UIView负责交互,显⽰CALayer绘制的内容。
4.2 CALayer的常⽤属性
属性 作⽤
CornerRadius 圆⾓
ShadowColor 阴影颜⾊
ShadowOffset 阴影偏移距离
ShadowRadius 阴影模糊程度
ShadowOpacity 阴影透明度
BorderWidth 描边粗细
BorderColor 描边颜⾊
anchorPoint 锚点
position 位置信息
transfrom 使CALayer产⽣3D空间内的平移、缩放、旋转等变化
4.3 anchorPoint和position
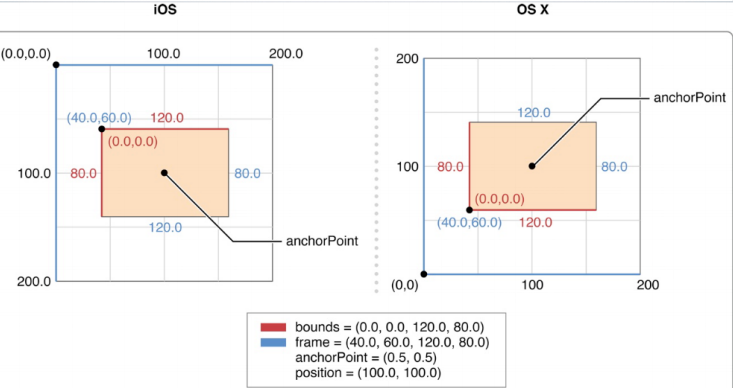
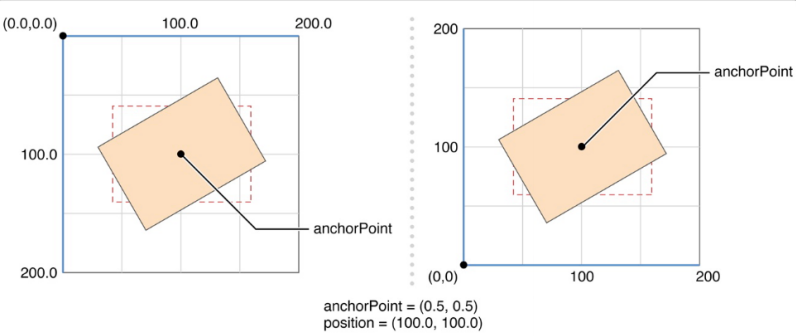
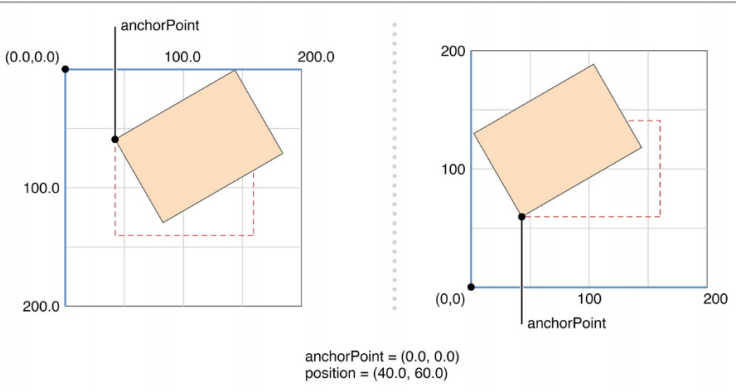
4.4 Demo
- (void)viewDidLoad {
[super viewDidLoad];
_imgView.backgroundColor = [UIColor redColor];
_imgView.layer.cornerRadius = 30;
// _imgView.layer.masksToBounds = YES; //如果设置masksToBounds=YES,设置阴影无效
_imgView.layer.anchorPoint = CGPointMake(0.5, 1);
_imgView.layer.shadowColor = [UIColor lightGrayColor].CGColor;
_imgView.layer.shadowOpacity = 1;
_imgView.layer.shadowOffset = CGSizeMake(10, 10);
_imgView.layer.shadowRadius = 1;
_myView.layer.cornerRadius = 50;
_myView.layer.shadowColor = [UIColor redColor].CGColor;
_myView.layer.shadowOpacity = 1;
_myView.layer.shadowOffset = CGSizeMake(10, 10);
#pragma mark -- 自定义CALayer --
[self customLayer];
// Do any additional setup after loading the view, typically from a nib.
}
- (void)customLayer
{
CALayer *myLayer = [CALayer layer];
//设置其位置和大小
myLayer.bounds = CGRectMake(0, 0, 50, 50);
myLayer.backgroundColor = [UIColor yellowColor].CGColor;
//设置锚点
myLayer.anchorPoint = CGPointMake(0, 0);
// 设置position(定位点,默认是(0,0)).锚点和position始终重合
myLayer.position = CGPointMake(50, 50);
//添加到父图层
[self.view.layer addSublayer:myLayer];
}
- (IBAction)didClickBasic:(id)sender {
/*
//1.设置动画对象
CABasicAnimation *basicAnimation = [CABasicAnimation animation];
//2.告诉系统要执行的动画,影响的是哪个属性
basicAnimation.keyPath = @"position";
//3.设置通过动画,将layer从哪移动到哪
basicAnimation.fromValue = [NSValue valueWithCGPoint:CGPointMake(100, 100)];
basicAnimation.toValue = [NSValue valueWithCGPoint:CGPointMake(200, 500)];
//取消回到原位置
basicAnimation.removedOnCompletion = NO;
//设置保存动画的最新状态
basicAnimation.fillMode = kCAFillModeForwards;
basicAnimation.duration = 3.0;
[self.imgView.layer addAnimation:basicAnimation forKey:@"basic"];
*/
//实现旋转
CABasicAnimation *basic = [CABasicAnimation animationWithKeyPath:@"transform"];
basic.duration = 2.0;
basic.toValue = [NSValue valueWithCATransform3D:CATransform3DMakeRotation(M_PI_4*4, 1, 1, 1)];
basic.removedOnCompletion = NO;
basic.fillMode = kCAFillModeForwards;
[self.imgView.layer addAnimation:basic forKey:@"basic"];
//移除动画
// [self.imgView.layer removeAnimationForKey:@"basic"];
}
#pragma mark -- IBActions -------
#pragma mark -- 帧动画 --
- (IBAction)didClickKeyFrame:(id)sender {
CAKeyframeAnimation *keyFrame = [CAKeyframeAnimation animationWithKeyPath:@"transform.rotation"];
//设置动画时间
keyFrame.duration = 0.1;
//设置imageView的抖动角度
//角度转换为弧度: 度数/180*M_PI
keyFrame.values = @[@(-4.0/180*M_PI),@(4.0/180*M_PI)];
keyFrame.repeatCount = 100;
[self.imgView.layer addAnimation:keyFrame forKey:@"keyFrame"];
}
#pragma mark -- CATransition转场动画 --
- (IBAction)didClickTransition:(id)sender {
//属性:
//type:设置动画过渡类型
//subType:动画过度的方向
//startProgress:动画起点
//endProgress:动画终点
self.index++;
self.index = _index%19 +1;
self.imgView.image = [UIImage imageNamed:[NSString stringWithFormat:@"image%d.jpg",self.index]];
CATransition *transition = [CATransition animation];
transition.type = @"cube";
transition.subtype = kCATransitionFromLeft;
transition.duration = 2;
transition.repeatCount = 10;
// transition.startProgress = 0;
// transition.endProgress = 1;
[self.imgView.layer addAnimation:transition forKey:@"transition"];
}
五、CAAnimation动画
CAAnimation是抽象类,通常使⽤它的⼦类实现动画效果。所有CAAnimation及其⼦类的对象都添加在View的layer上,例如: [view.layer addAnimation:animation forKey:nil];
5.1 给layer添加/移除CALayer动画
- (void)addAnimation:(CAAnimation *)anim forKey:(NSString *)key;
- (void)removeAnimationForKey:(NSString *)key;
- (void)removeAllAnimations;
5.2 CAAnimation相关⼦类
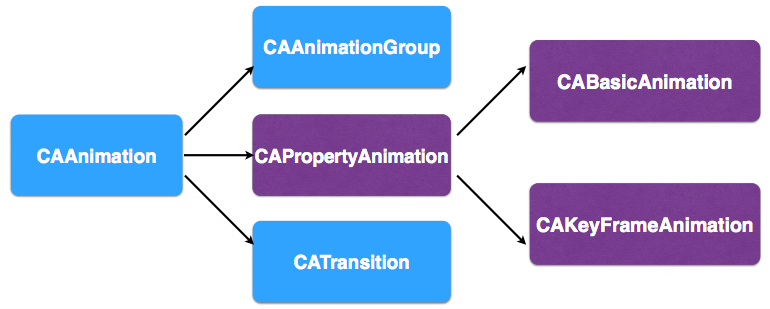
CAPropertyAnimation也是⼀个抽象类 通常我们都使⽤它的两个⼦类:CABasicAnimation和 CAKeyFrameAnimation
5.2.1 CABasicAnimation
作⽤:基本layer动画,通过设定初始和结束值执⾏动画
+ (id)animationWithKeyPath:(NSString *)path; 系统提供的构造器⽅法
@property(copy) NSString *keyPath; 只能填写CALayer中能够做
动画的属性名
@property(retain) id fromValue; 起始值
@property(retain) id toValue; 结束值
@property(retain) id byValue; 相对值
5.2.2 CAKeyFrameAnimation
作⽤:关键帧动画,可以让你的view的layer按照预定轨迹做动画
⽅法名/属性名 作⽤
+ (id)animationWithKeyPath:(NSString *)path; 系统提供的构造器⽅法
@property CGPathRef path; 通过制定⼀个⾃⼰定义的path来让某⼀个物体按照
这个路径进⾏动画
@property(copy) NSArray *values; ⼀个数组,提供了⼀组关键帧的值, 当使⽤path的
时候 values的值⾃动被忽略。
@property(copy) NSArray *keyTimes; ⼀个数组,设置每⼀帧的时间,其成员必须是
NSNumber。设置详情查看API。
@property(copy) NSString *rotationMode; 设定关键帧中间的值是如何计算
5.3 CAAnimaitionGroup
CAAnimationGroup只有⼀个数组属性,可以添加多个CAAnimation⼀起执⾏
5.3.1 CATrasition
作⽤:layer的过渡动画
有两个主要属性:type(设置过渡动画的效果)和subType(设置过渡动画的⽅向)
5.4 DEMO
- (IBAction)didGroupAnimation:(id)sender {
// 平移动画
CABasicAnimation *a1 = [CABasicAnimation animation];
a1.keyPath = @"transform.translation.y";
a1.toValue = @(100);
// 缩放动画
CABasicAnimation *a2 = [CABasicAnimation animation];
a2.keyPath = @"transform.scale";
a2.toValue = @(0.0);
// 旋转动画
CABasicAnimation *a3 = [CABasicAnimation animation];
a3.keyPath = @"transform.rotation";
a3.toValue = @(M_PI_2);
CAAnimationGroup *group = [CAAnimationGroup animation];
group.animations = @[a1, a2, a3];
group.fillMode = kCAFillModeForwards;
// group.removedOnCompletion = NO;
[self.imgView.layer addAnimation:group forKey:@"group"];
}
相关文章推荐
- ios开发-新浪微博10-(下拉菜单的二次封装 完整版)
- ios开发-新浪微博-09(下拉菜单封装一)
- wxhl iOS bj 49 张浩 学习心得体会连载 第10章
- ios开发-新浪微博08-下拉菜单的基本实现
- ios-新浪微博开发07-自定义搜索框
- 猫猫学iOS 之微博项目实战(10)微博cell中图片的显示以及各种填充模式简介
- iOS现成的引导页面的实现:纯代码
- ios7.1以后,iphone上隐藏应用图标的方法
- IOS afn三方上传图片
- IOS rumen
- iOS开发 -- WebSocket 通信(一)
- iOS中FMDB简介
- IOS-六种手势的简单使用
- IOS开发学习的思维导图
- iOS6 创建全局的pch文件
- iOS中几种数据永久存储方式
- iOS 9: Getting Started With SFSafariViewController
- iOS 9适配
- iOS 7的手势滑动返回功能
- iOS开发小技巧总汇(不定时增添)