Linux网络编程--select()和pselect()函数
2015-09-10 17:14
316 查看
函数select()和pselect()用于IO复用,它们监视多个文件描述符的集合,判断是否有符合条件的时间发生。
1.select()函数
函数select()与之前的recv()和send()直接操作文件描述符不同。使用select()函数可以先对需要操作的文件描述符进行查询,查看是否目标文件描述符可以进行读、写或者错误操作,然后当文件描述符满足操作的条件的时候才进行真正的IO操作。
select()函数原型以及解释说明如下:
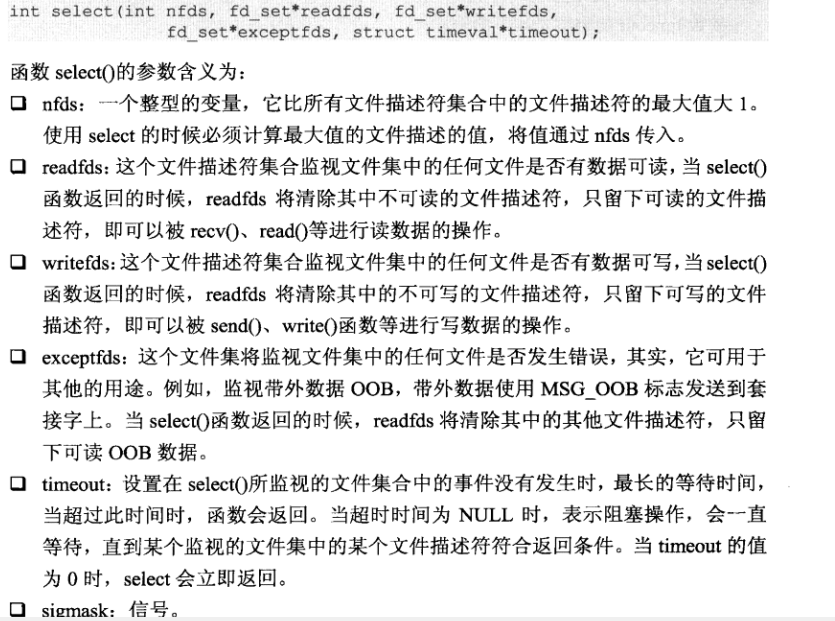
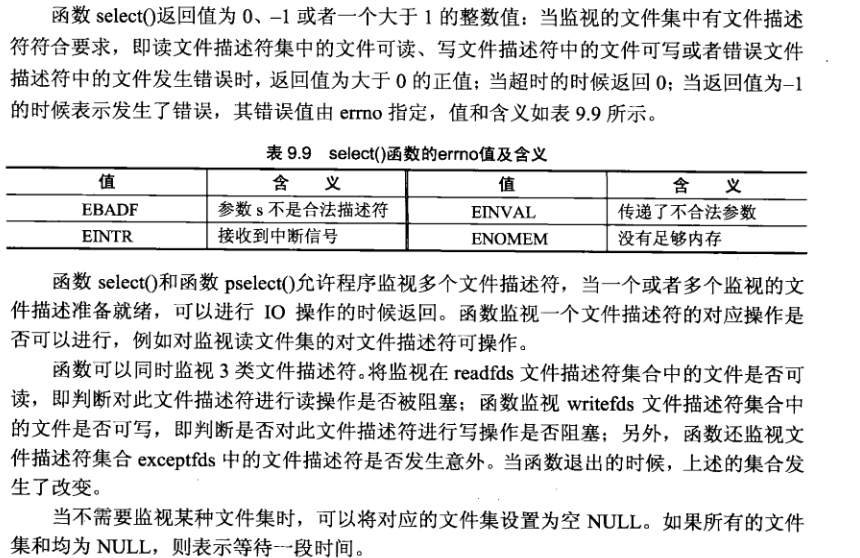
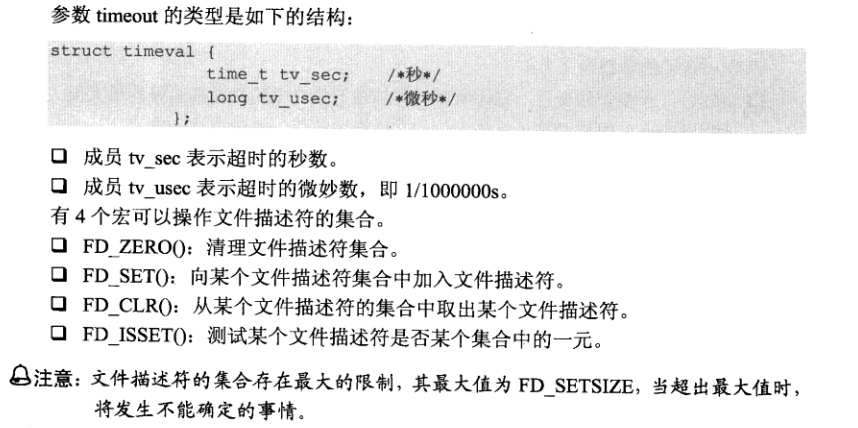
select()函数例子:
使用select函数监视标准输入是否有数据处理,设置超时时间为5s。
2.pselect()函数
函数select()是用一种超时轮循的方式来查看文件的读写错误可操作性。在Linux下,还有一个相似的函数pselect()。
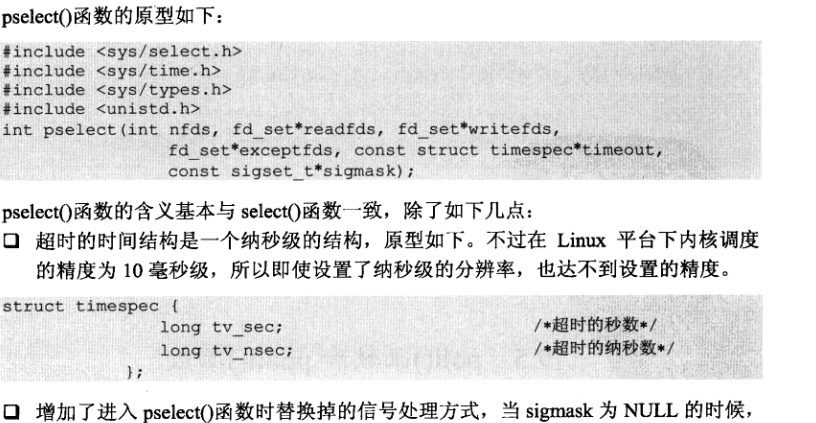
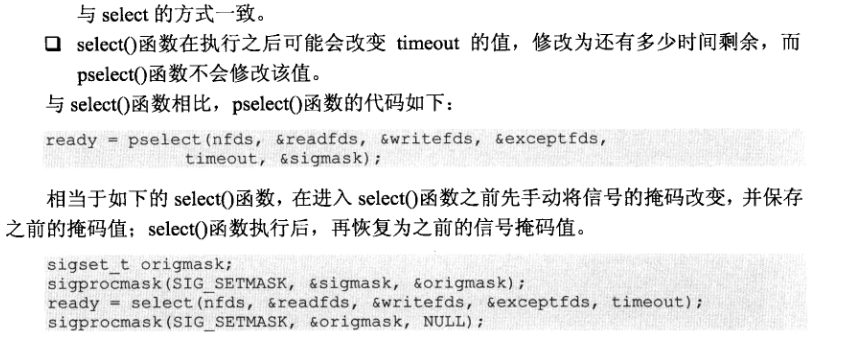
pselect()函数例子:
最后介绍重量级select()函数例子:
1.select()函数
函数select()与之前的recv()和send()直接操作文件描述符不同。使用select()函数可以先对需要操作的文件描述符进行查询,查看是否目标文件描述符可以进行读、写或者错误操作,然后当文件描述符满足操作的条件的时候才进行真正的IO操作。
select()函数原型以及解释说明如下:
select()函数例子:
使用select函数监视标准输入是否有数据处理,设置超时时间为5s。
#include<unistd.h> #include<sys/time.h> #include<sys/types.h> int main(){ fd_set rd;//定义fd_set类型的读描述符集合 struct timeval tv;//timeval 结构 int retval;//select返回值 FD_ZERO(&rd);//清空读描述符集合 FD_SET(0,&rd);//将描述符标准输入0放入读描述符集合中 tv.tv_sec=5; tv.tv_usec=0;//设置超时间为5S,select函数调用返回的时候会修改timeval的值为剩余的时间。 retval=select(2,&rd,NULL,NULL,&tv);//调用select函数监听标准输入,fd最大描述符个数加1(这里只监听标准输入一个描述符,那么这个值就是1+1,也就是2) // printf("[tv.tv_sec:%d]\n",tv.tv_sec); if(retval==-1){ perror("select"); } else if(retval){ printf("Data is avaialble now\n"); } else{ printf("NO Data within five seconds\n"); } return 0; }
2.pselect()函数
函数select()是用一种超时轮循的方式来查看文件的读写错误可操作性。在Linux下,还有一个相似的函数pselect()。
pselect()函数例子:
#include<unistd.h> #include<sys/time.h> #include<sys/types.h> #include <signal.h> int sigint_event=0; void sigint_sig_handler(int s){//设置信号处理函数 sigint_event++; signal(SIGINT,sigint_sig_handler); } int main(){ int r; fd_set rd; sigset_t sigmask,orignal_sigmask;//设置新掩码和保存原始掩码 FD_ZERO(&rd);//清空读描述符集合 FD_SET(0,&rd);//将标准输入放入读描述符集合 sigemptyset(&sigmask);//清空信号 sigaddset(&sigmask,SIGINT);//将SIGINT信号加入sigmask中 //设置信号SIG_BLOCK的掩码sigmask,并将原始的掩码保存在orignal_sigmask中 sigprocmask(SIG_BLOCK,&sigmask,&orignal_sigmask); signal(SIGINT,sigint_sig_handler);//挂接信号处理函数 for(;;){ for(;sigint_event>0;sigint_event--){ printf("sigint_event[%d]\n",sigint_event); } r=pselect(1,&rd,NULL,NULL,NULL,&orignal_sigmask);//pselect函数IO复用 if(r==-1){ perror("pselect"); } else if(r){ printf("Data is avaialble now\n"); } else{ printf("NO Data within five seconds\n"); } sleep(1); } return 0; }
最后介绍重量级select()函数例子:
/* select使用的例子 */ #include <stdlib.h> #include <stdio.h> #include <unistd.h> #include <sys/time.h> #include <sys/types.h> #include <string.h> #include <signal.h> #include <sys/socket.h> #include <netinet/in.h> #include <arpa/inet.h> #include <errno.h> static int forward_port; #undef max #define max(x,y) ((x) > (y) ? (x) : (y)) static int listen_socket (int listen_port) { struct sockaddr_in a; int s; int yes; if ((s = socket (AF_INET, SOCK_STREAM, 0)) < 0) { perror ("socket"); return -1; } yes = 1; if (setsockopt (s, SOL_SOCKET, SO_REUSEADDR, (char *) &yes, sizeof (yes)) < 0) { perror ("setsockopt"); close (s); return -1; } memset (&a, 0, sizeof (a)); a.sin_port = htons (listen_port); a.sin_family = AF_INET; if (bind (s, (struct sockaddr *) &a, sizeof (a)) < 0) { perror ("bind"); close (s); return -1; } printf ("accepting connections on port %d\n", (int) listen_port); listen (s, 10); return s; } static int connect_socket (int connect_port, char *address) { struct sockaddr_in a; int s; if ((s = socket (AF_INET, SOCK_STREAM, 0)) < 0) { perror ("socket"); close (s); return -1; } memset (&a, 0, sizeof (a)); a.sin_port = htons (connect_port); a.sin_family = AF_INET; if (!inet_aton (address, (struct in_addr *) &a.sin_addr.s_addr)) { perror ("bad IP address format"); close (s); return -1; } if (connect (s, (struct sockaddr *) &a, sizeof (a)) < 0) { perror ("connect()"); shutdown (s, SHUT_RDWR); close (s); return -1; } return s; } #define SHUT_FD1 { \ if (fd1 >= 0) { \ shutdown (fd1, SHUT_RDWR); \ close (fd1); \ fd1 = -1; \ } \ } #define SHUT_FD2 { \ if (fd2 >= 0) { \ shutdown (fd2, SHUT_RDWR); \ close (fd2); \ fd2 = -1; \ } \ } /* 缓冲区的大小为1024 */ #define BUF_SIZE 1024 int main (int argc, char **argv) { int h; int fd1 = -1, fd2 = -1; char buf1[BUF_SIZE], buf2[BUF_SIZE]; int buf1_avail, buf1_written; int buf2_avail, buf2_written; if (argc != 4) { fprintf (stderr, "Usage\n\tfwd <listen-port> \ <forward-to-port> <forward-to-ip-address>\n"); exit (1); } signal (SIGPIPE, SIG_IGN); forward_port = atoi (argv[2]); h = listen_socket (atoi (argv[1])); if (h < 0) exit (1); /* 主循环 */ for (;;) { int r, nfds = 0; fd_set rd, wr, er; /*定义用于读、写和错误的文件集*/ FD_ZERO (&rd); /*清空读文件集描述符和*/ FD_ZERO (&wr); /*清空写文件描述符集和*/ FD_ZERO (&er); /*清空错误文件描述符集和*/ FD_SET (h, &rd); /*将服务器的侦听套接字放入可读文件描述符集合*/ nfds = max (nfds, h); if (fd1 > 0 && buf1_avail < BUF_SIZE) { FD_SET (fd1, &rd); nfds = max (nfds, fd1); } if (fd2 > 0 && buf2_avail < BUF_SIZE) { FD_SET (fd2, &rd); nfds = max (nfds, fd2); } if (fd1 > 0 && buf2_avail - buf2_written > 0) { FD_SET (fd1, &wr); nfds = max (nfds, fd1); } if (fd2 > 0 && buf1_avail - buf1_written > 0) { FD_SET (fd2, &wr); nfds = max (nfds, fd2); } if (fd1 > 0) { FD_SET (fd1, &er); nfds = max (nfds, fd1); } if (fd2 > 0) { FD_SET (fd2, &er); nfds = max (nfds, fd2); } r = select (nfds + 1, &rd, &wr, &er, NULL); if (r == -1 && errno == EINTR) continue; if (r < 0) { perror ("select()"); exit (1); } if (FD_ISSET (h, &rd)) { unsigned int l; struct sockaddr_in client_address; memset (&client_address, 0, l = sizeof (client_address)); r = accept (h, (struct sockaddr *) &client_address, &l); if (r < 0) { perror ("accept()"); } else { SHUT_FD1; SHUT_FD2; buf1_avail = buf1_written = 0; buf2_avail = buf2_written = 0; fd1 = r; fd2 = connect_socket (forward_port, argv[3]); if (fd2 < 0) { SHUT_FD1; } else printf ("connect from %s\n", inet_ntoa (client_address.sin_addr)); } } /* NB: read oob data before normal reads */ if (fd1 > 0) if (FD_ISSET (fd1, &er)) { char c; errno = 0; r = recv (fd1, &c, 1, MSG_OOB); if (r < 1) { SHUT_FD1; } else send (fd2, &c, 1, MSG_OOB); } if (fd2 > 0) if (FD_ISSET (fd2, &er)) { char c; errno = 0; r = recv (fd2, &c, 1, MSG_OOB); if (r < 1) { SHUT_FD1; } else send (fd1, &c, 1, MSG_OOB); } if (fd1 > 0) if (FD_ISSET (fd1, &rd)) { r = read (fd1, buf1 + buf1_avail, BUF_SIZE - buf1_avail); if (r < 1) { SHUT_FD1; } else buf1_avail += r; } if (fd2 > 0) if (FD_ISSET (fd2, &rd)) { r = read (fd2, buf2 + buf2_avail, BUF_SIZE - buf2_avail); if (r < 1) { SHUT_FD2; } else buf2_avail += r; } if (fd1 > 0) if (FD_ISSET (fd1, &wr)) { r = write (fd1, buf2 + buf2_written, buf2_avail - buf2_written); if (r < 1) { SHUT_FD1; } else buf2_written += r; } if (fd2 > 0) if (FD_ISSET (fd2, &wr)) { r = write (fd2, buf1 + buf1_written, buf1_avail - buf1_written); if (r < 1) { SHUT_FD2; } else buf1_written += r; } /* check if write data has caught read data */ if (buf1_written == buf1_avail) buf1_written = buf1_avail = 0; if (buf2_written == buf2_avail) buf2_written = buf2_avail = 0; /* one side has closed the connection, keep writing to the other side until empty */ if (fd1 < 0 && buf1_avail - buf1_written == 0) { SHUT_FD2; } if (fd2 < 0 && buf2_avail - buf2_written == 0) { SHUT_FD1; } } return 0; }
相关文章推荐
- android6.0SDK中删除HttpClient的相关类的解决方法
- ZOJ 3229 Shoot the Bullet 有源汇上下界网络流 最大流
- HTTP请求详解
- 从贝叶斯方法谈到贝叶斯网络
- ZOJ 2314 Reactor Cooling 无源汇上下界网络流 可行流
- 构造HTTP请求Header实现“伪造来源IP”
- MCC(移动国家码)和 MNC(移动网络码)
- HTTP/2 常见问题回答
- 【Http】HTTP报文结构及请求数据大小
- U3D 网络库实现通信 基于Warensoft Unity3d
- HTTP协议详解
- 异构网络
- [置顶] Linux网络编程--IO模型基础
- Android HttpClient post MultipartEntity - Android 上传文件
- TCP的数据流——滑动窗口,拥塞窗口,慢启动,Nagle算法,经受时延的确认等
- http-关于application/x-www-form-urlencoded等字符编码的解释说明
- tcp学习系列
- HTTP 协议详解
- https和http有何区别
- Linux Shell下的后台运行及其前台的转换 http://mobile.51cto.com/others-446925.htm