C++ 引用
2015-07-10 09:55
309 查看
本文主要记录了C++中的引用特性,总结了一下代码示例和错误。
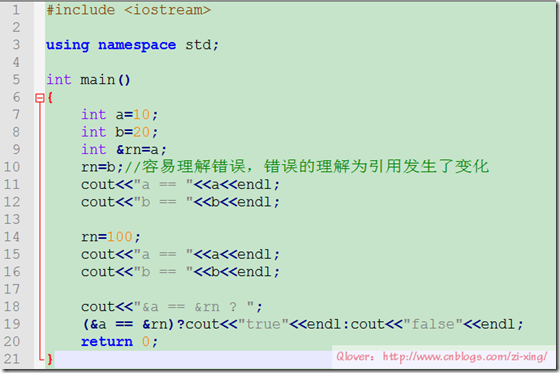
引用是C++的特性,指针是C语言的特性。上面代码结果如下:
20
20
100
20
true
引用和被引用的对象是占用同一个内存单元的,具有同一的地址。
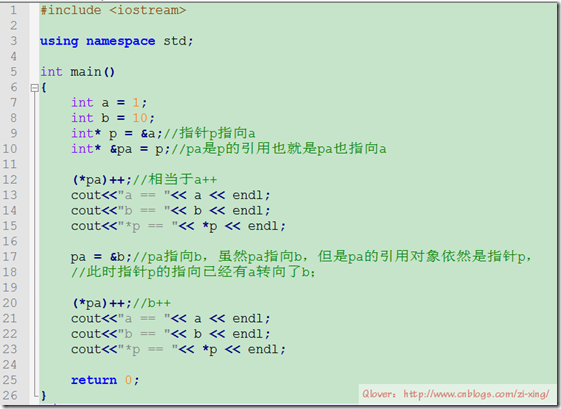
对于引用,创建的时候就要说明引用的对象是哪一个,而且以后都不会修改这个引用的对象。引用就相当于一个变量的别名。

简单的引用
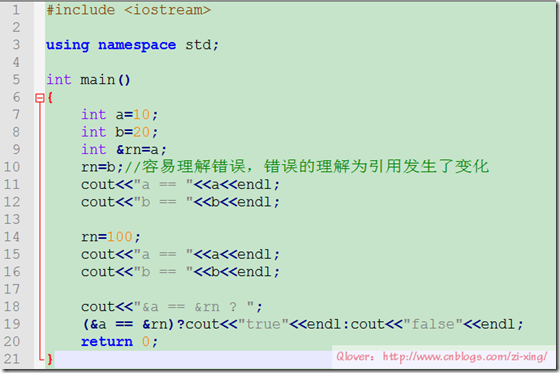
引用是C++的特性,指针是C语言的特性。上面代码结果如下:
20
20
100
20
true
引用和被引用的对象是占用同一个内存单元的,具有同一的地址。
指针变量引用
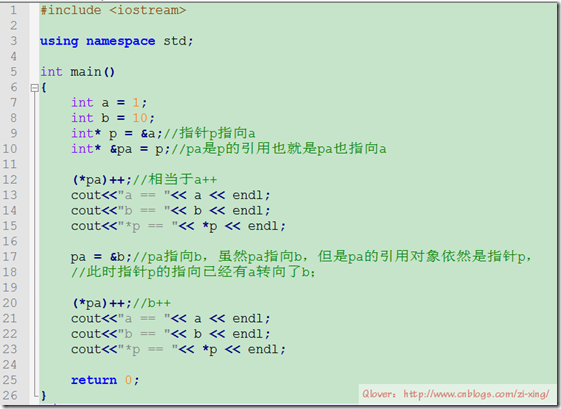
对于引用,创建的时候就要说明引用的对象是哪一个,而且以后都不会修改这个引用的对象。引用就相当于一个变量的别名。
引用错误示例

交换2个字符串
#include <iostream> #include <string.h> using namespace std; void swapOne(char *&x,char *&y)//指针的引用 { char *temp; temp = x; x = y; y = temp; } void swapTwo(char **x,char **y)//指针的指针 { char *temp; temp = *x; *x = *y; *y = temp; } void swapThree(char *x,char *y)//指针的传值(这种方法是不行的,相当于值传递) {//有人会误解这个,因为可能使用过 利用指针交换2个整数。这还是有差别的。 char *temp; temp = x; x = y; y = temp; } int main() { char *ap = "Hello";//*ap的内容是H,*bp的内容是H char *bp = "How are you?"; cout<<"ap: "<< ap << endl; cout<<"bp: "<< bp << endl; //swapOne(ap,bp); //swapTwo(&ap,&bp); swapThree(ap,bp); cout<<"swap ap,bp"<<endl; cout<<"ap: "<< ap << endl; cout<<"bp: "<< bp << endl; return 0; }
引用错误示例2
#include <iostream> using namespace std; const float pi=3.14f;//常量 float f;//全局变量 float f1(float r) { f = r*r*pi; return f; } float& f2(float r)//返回引用 { f = r*r*pi; return f; //局部变量的引用返回(注意有效期),系统运行正常,但是结果会出错。 /*float ff = f; return ff;*/ } int main() { float f1(float=5);//默认参数5,可以修改全局变量f=78.5 float& f2(float=5);//同上 float a=f1(); //float& b=f1();//f1()函数中,全局变量f的值78.1赋给一个临时变量temp(由编译器隐式建立),然后建立这个temp的引用b,对一个临时变量temp引用会发生错误 float c=f2(); float& d=f2();//主函数中不使用定义变量,而是直接使用全局变量的引用。这种方式在全部4中方式中,最节省内存空间。但须注意它所引用的有效期。 //此处的全局变量f的有效期肯定长于引用d,所以安全。例如,将一个局部变量的引用返回。 d += 1.0f; cout<<"a = "<< a <<endl; //cout<<"b = "<< b <<endl; cout<<"c = "<< c <<endl; cout<<"d = "<< d <<endl; cout<<"f = "<< f <<endl; return 0; }
引用常见错误
#include <iostream> using namespace std; class Test { public: void f(const int& arg); private: int value; }; int main() { int a = 7; const int b = 10; //int &c = b;//错误:b为常量,但是C不是常量引用,正确的:const int &c=b; const int &d = a; a++; //d++;//错误,d为常量引用,所以不能修改 Test test; test.f(a); cout << "a = "<< a <<endl; return 0; } void Test::f(const int &arg) { //arg = 10;//常量不能修改 cout <<"arg = "<< arg <<endl; value = 20; } /* * 对于常量类型的变量,其引用也必须是常量类型的。 * 对于非常量类型的变量,其可以是非常量的。 * 但是,注意:无论什么情况下,都不能使用常量引用修改引用的变量的值。 */
相关文章推荐
- 浅谈C语言中的联合体
- c语言基本数据类型所占的位数
- Jni中C++和Java的参数传递
- Windows下使用Sublime Text 3调试/运行 C/C++ 程序
- C++ 之cin与cout常见问题详解
- sift算法c语言实现
- C++运算符重载(成员函数方式)
- C语言中unsigned char* 与char*的区别?
- C++指针学习
- Java通过JNI调用C++程序
- hdu 1159, LCS, dynamic programming, recursive backtrack vs iterative backtrack vs incremental, C++
- 关于Eclipse C++出现Launch failed,Binary not found问题的解决方案(Win7环境下)
- Longest Substring Without Repeating Characters 7 lines with c++ in 12ms
- C++默认构造函数
- C++类的底层机理
- Regular Expression Matching
- C++堆和栈区别
- c++内存分配方式
- 1985年公布的C++语言1.0版的内容中又添加了一些重要特征
- C++这个名字