12_Android中HttpClient的应用,doGet,doPost,doHttpClientGet,doHttpClient请求,另外借助第三方框架实现网络连接的应用,
2015-07-03 02:08
771 查看
准备条件,
编写一个web项目。编写一个servlet,若用户名为lisi,密码为123,则返回“登录成功”,否则”登录失败”。项目名为ServerItheima28。代码如下:
使用github上的android-async-http-master框架:
2 编写Android应用,应用截图如下:
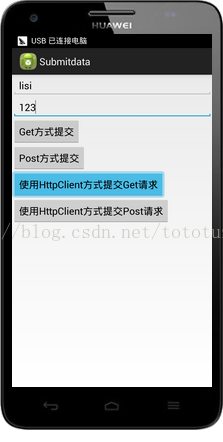
代码结构如下:
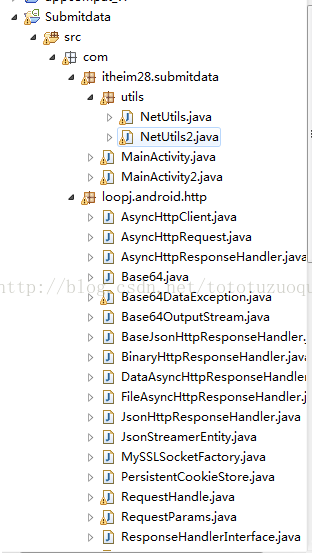
清单文件
3 编写布局文件activity_main.xml
4 NetUtils的内容如下:
5 NetUtils2代码如下:
6 MainActivity代码如下:
7 MainActivity2 的代码如下:
编写一个web项目。编写一个servlet,若用户名为lisi,密码为123,则返回“登录成功”,否则”登录失败”。项目名为ServerItheima28。代码如下:
package com.itheima28.servlet; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class LoginServlet extends HttpServlet { /** * The doGet method of the servlet. <br> * * This method is called when a form has its tag value method equals to get. * * @param request the request send by the client to the server * @param response the response send by the server to the client * @throws ServletException if an error occurred * @throws IOException if an error occurred */ public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String username = request.getParameter("username"); // 采用的编码是: iso-8859-1 String password = request.getParameter("password"); // 采用iso8859-1的编码对姓名进行逆转, 转换成字节数组, 再使用utf-8编码对数据进行转换, 字符串 username = new String(username.getBytes("iso8859-1"), "utf-8"); password = new String(password.getBytes("iso8859-1"), "utf-8"); System.out.println("姓名: " + username); System.out.println("密码: " + password); if("lisi".equals(username) && "123".equals(password)) { /* * getBytes 默认情况下, 使用的iso8859-1的编码, 但如果发现码表中没有当前字符, * 会使用当前系统下的默认编码: GBK */ response.getOutputStream().write("登录成功".getBytes("utf-8")); } else { response.getOutputStream().write("登录失败".getBytes("utf-8")); } } /** * The doPost method of the servlet. <br> * * This method is called when a form has its tag value method equals to post. * * @param request the request send by the client to the server * @param response the response send by the server to the client * @throws ServletException if an error occurred * @throws IOException if an error occurred */ public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("doPost"); doGet(request, response); } } |
2 编写Android应用,应用截图如下:
代码结构如下:
清单文件
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.itheim28.submitdata" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="19" /> <uses-permission android:name="android.permission.INTERNET"/> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.itheim28.submitdata.MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest> |
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity" > <EditText android:id="@+id/et_username" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="请输入姓名" /> <EditText android:id="@+id/et_password" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="请输入密码" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="doGet" android:text="Get方式提交" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="doPost" android:text="Post方式提交" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="doHttpClientOfGet" android:text="使用HttpClient方式提交Get请求" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:onClick="doHttpClientOfPost" android:text="使用HttpClient方式提交Post请求" /> </LinearLayout> |
package com.itheim28.submitdata.utils; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import android.util.Log; public class NetUtils { private static final String TAG = "NetUtils"; /** * 使用post的方式登录 * * @param userName * @param password * @return */ public static String loginOfPost(String userName, String password) { HttpURLConnection conn = null; try { URL url = new URL( "http://114.215.142.191:8080/ServerItheima28/servlet/LoginServlet"); conn = (HttpURLConnection) url.openConnection(); conn.setRequestMethod("POST"); conn.setConnectTimeout(10000); // 连接的超时时间 conn.setReadTimeout(5000); // 读数据的超时时间 conn.setDoOutput(true); // 必须设置此方法, 允许输出 // conn.setRequestProperty("Content-Length", 234); // 设置请求头消息, // 可以设置多个 // post请求的参数 String data = "username=" + userName + "&password=" + password; // 获得一个输出流, 用于向服务器写数据, 默认情况下, 系统不允许向服务器输出内容 OutputStream out = conn.getOutputStream(); out.write(data.getBytes()); out.flush(); out.close(); int responseCode = conn.getResponseCode(); if (responseCode == 200) { InputStream is = conn.getInputStream(); String state = getStringFromInputStream(is); return state; } else { Log.i(TAG, "访问失败: " + responseCode); } } catch (Exception e) { e.printStackTrace(); } finally { if (conn != null) { conn.disconnect(); } } return null; } /** * 使用get的方式登录 * * @param userName * @param password * @return 登录的状态 */ public static String loginOfGet(String userName, String password) { HttpURLConnection conn = null; try { String data = "username=" + URLEncoder.encode(userName) + "&password=" + URLEncoder.encode(password); URL url = new URL( "http://114.215.142.191:8080/ServerItheima28/servlet/LoginServlet?" + data); conn = (HttpURLConnection) url.openConnection(); conn.setRequestMethod("GET"); // get或者post必须得全大写 conn.setConnectTimeout(10000); // 连接的超时时间 conn.setReadTimeout(5000); // 读数据的超时时间 int responseCode = conn.getResponseCode(); if (responseCode == 200) { InputStream is = conn.getInputStream(); String state = getStringFromInputStream(is); return state; } else { Log.i(TAG, "访问失败: " + responseCode); } } catch (Exception e) { e.printStackTrace(); } finally { if (conn != null) { conn.disconnect(); // 关闭连接 } } return null; } /** * 根据流返回一个字符串信息 * * @param is * @return * @throws IOException */ private static String getStringFromInputStream(InputStream is) throws IOException { ByteArrayOutputStream baos = new ByteArrayOutputStream(); byte[] buffer = new byte[1024]; int len = -1; while ((len = is.read(buffer)) != -1) { baos.write(buffer, 0, len); } is.close(); String html = baos.toString(); // 把流中的数据转换成字符串, 采用的编码是: utf-8 // String html = new String(baos.toByteArray(), "GBK"); baos.close(); return html; } } |
package com.itheim28.submitdata.utils; import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; import java.util.ArrayList; import java.util.List; import org.apache.http.HttpResponse; import org.apache.http.NameValuePair; import org.apache.http.client.HttpClient; import org.apache.http.client.entity.UrlEncodedFormEntity; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.impl.client.DefaultHttpClient; import org.apache.http.message.BasicNameValuePair; import android.util.Log; public class NetUtils2 { private static final String TAG = "NetUtils"; /** * 使用post的方式登录 * * @param userName * @param password * @return */ public static String loginOfPost(String userName, String password) { HttpClient client = null; try { // 定义一个客户端 client = new DefaultHttpClient(); // 定义post方法 HttpPost post = new HttpPost( "http://114.215.142.191:8080/ServerItheima28/servlet/LoginServlet"); // 定义post请求的参数 List<NameValuePair> parameters = new ArrayList<NameValuePair>(); parameters.add(new BasicNameValuePair("username", userName)); parameters.add(new BasicNameValuePair("password", password)); // 把post请求的参数包装了一层. // 不写编码名称服务器收数据时乱码. 需要指定字符集为utf-8 UrlEncodedFormEntity entity = new UrlEncodedFormEntity(parameters, "utf-8"); // 设置参数. post.setEntity(entity); // 设置请求头消息 // post.addHeader("Content-Length", "20"); // 使用客户端执行post方法 HttpResponse response = client.execute(post); // 开始执行post请求, // 会返回给我们一个HttpResponse对象 // 使用响应对象, 获得状态码, 处理内容 int statusCode = response.getStatusLine().getStatusCode(); // 获得状态码 if (statusCode == 200) { // 使用响应对象获得实体, 获得输入流 InputStream is = response.getEntity().getContent(); String text = getStringFromInputStream(is); return text; } else { Log.i(TAG, "请求失败: " + statusCode); } } catch (Exception e) { e.printStackTrace(); } finally { if (client != null) { client.getConnectionManager().shutdown(); // 关闭连接和释放资源 } } return null; } /** * 使用get的方式登录 * * @param userName * @param password * @return 登录的状态 */ public static String loginOfGet(String userName, String password) { HttpClient client = null; try { // 定义一个客户端 client = new DefaultHttpClient(); // 定义一个get请求方法 String data = "username=" + userName + "&password=" + password; HttpGet get = new HttpGet( "http://114.215.142.191:8080/ServerItheima28/servlet/LoginServlet?" + data); // response 服务器相应对象, 其中包含了状态信息和服务器返回的数据 // 开始执行get方法, 请求网络 HttpResponse response = client.execute(get); // 获得响应码 int statusCode = response.getStatusLine().getStatusCode(); if (statusCode == 200) { InputStream is = response.getEntity().getContent(); String text = getStringFromInputStream(is); return text; } else { Log.i(TAG, "请求失败: " + statusCode); } } catch (Exception e) { e.printStackTrace(); } finally { if (client != null) { client.getConnectionManager().shutdown(); // 关闭连接, 和释放资源 } } return null; } /** * 根据流返回一个字符串信息 * * @param is * @return * @throws IOException */ private static String getStringFromInputStream(InputStream is) throws IOException { ByteArrayOutputStream baos = new ByteArrayOutputStream(); byte[] buffer = new byte[1024]; int len = -1; while ((len = is.read(buffer)) != -1) { baos.write(buffer, 0, len); } is.close(); String html = baos.toString(); // 把流中的数据转换成字符串, 采用的编码是: utf-8 // String html = new String(baos.toByteArray(), "GBK"); baos.close(); return html; } } |
package com.itheim28.submitdata; import android.app.Activity; import android.os.Bundle; import android.text.TextUtils; import android.util.Log; import android.view.View; import android.widget.EditText; import android.widget.Toast; import com.itheim28.submitdata.utils.NetUtils; import com.itheim28.submitdata.utils.NetUtils2; public class MainActivity extends Activity { private static final String TAG = "MainActivity"; private EditText etUserName; private EditText etPassword; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); etUserName = (EditText) findViewById(R.id.et_username); etPassword = (EditText) findViewById(R.id.et_password); } /** * 使用httpClient方式提交get请求 * * @param v */ public void doHttpClientOfGet(View v) { Log.i(TAG, "doHttpClientOfGet"); final String userName = etUserName.getText().toString(); final String password = etPassword.getText().toString(); new Thread(new Runnable() { @Override public void run() { // 请求网络 final String state = NetUtils2.loginOfGet(userName, password); // 执行任务在主线程中 runOnUiThread(new Runnable() { @Override public void run() { // 就是在主线程中操作 Toast.makeText(MainActivity.this, state, 0).show(); } }); } }).start(); } /** * 使用httpClient方式提交post请求 * * @param v */ public void doHttpClientOfPost(View v) { Log.i(TAG, "doHttpClientOfPost"); final String userName = etUserName.getText().toString(); final String password = etPassword.getText().toString(); new Thread(new Runnable() { @Override public void run() { final String state = NetUtils2.loginOfPost(userName, password); // 执行任务在主线程中 runOnUiThread(new Runnable() { @Override public void run() { // 就是在主线程中操作 Toast.makeText(MainActivity.this, state, 0).show(); } }); } }).start(); } public void doGet(View v) { final String userName = etUserName.getText().toString(); final String password = etPassword.getText().toString(); new Thread(new Runnable() { @Override public void run() { // 使用get方式抓去数据 final String state = NetUtils.loginOfGet(userName, password); // 执行任务在主线程中 runOnUiThread(new Runnable() { @Override public void run() { // 就是在主线程中操作 Toast.makeText(MainActivity.this, state, 0).show(); } }); } }).start(); } public void doPost(View v) { final String userName = etUserName.getText().toString(); final String password = etPassword.getText().toString(); new Thread(new Runnable() { @Override public void run() { final String state = NetUtils.loginOfPost(userName, password); // 执行任务在主线程中 runOnUiThread(new Runnable() { @Override public void run() { // 就是在主线程中操作 Toast.makeText(MainActivity.this, state, 0).show(); } }); } }).start(); } } |
package com.itheim28.submitdata; import java.net.URLEncoder; import org.apache.http.Header; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.Toast; import com.loopj.android.http.AsyncHttpClient; import com.loopj.android.http.AsyncHttpResponseHandler; import com.loopj.android.http.RequestParams; public class MainActivity2 extends Activity { protected static final String TAG = "MainActivity2"; private EditText etUserName; private EditText etPassword; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); etUserName = (EditText) findViewById(R.id.et_username); etPassword = (EditText) findViewById(R.id.et_password); } public void doGet(View v) { final String userName = etUserName.getText().toString(); final String password = etPassword.getText().toString(); AsyncHttpClient client = new AsyncHttpClient(); String data = "username=" + URLEncoder.encode(userName) + "&password=" + URLEncoder.encode(password); client.get("http://114.215.142.191:8080/ServerItheima28/servlet/LoginServlet?" + data, new MyResponseHandler()); } public void doPost(View v) { final String userName = etUserName.getText().toString(); final String password = etPassword.getText().toString(); AsyncHttpClient client = new AsyncHttpClient(); RequestParams params = new RequestParams(); params.put("username", userName); params.put("password", password); client.post( "http://114.215.142.191:8080/ServerItheima28/servlet/LoginServlet", params, new MyResponseHandler()); } class MyResponseHandler extends AsyncHttpResponseHandler { @Override public void onSuccess(int statusCode, Header[] headers, byte[] responseBody) { // Log.i(TAG, "statusCode: " + statusCode); Toast.makeText( MainActivity2.this, "成功: statusCode: " + statusCode + ", body: " + new String(responseBody), 0).show(); } @Override public void onFailure(int statusCode, Header[] headers, byte[] responseBody, Throwable error) { Toast.makeText(MainActivity2.this, "失败: statusCode: " + statusCode, 0).show(); } } } |
相关文章推荐
- 12_Android中HttpClient的应用,doGet,doPost,doHttpClientGet,doHttpClient请求,另外借助第三方框架实现网络连接的应用,
- TCP/IP:链路层
- 美国政府网站全网HTTPS加密 国内CA呼吁借鉴
- tcpdump命令格式
- Http的请求方式 详解
- java网络编程(上)
- 查看网络图片功能的应用程序
- android之ConnectivityManager简介,网络连接状态
- tcpdump一些选项的使用
- BP神经网络PYthon实现(带有”增加充量项“)
- ajax之“解读原生态ajax”
- CocoaAsyncSocket网络通信使用之tcp连接(一)
- SessionA和pplication网上聊天室的网络范例
- 网络最大流问题之Ford-Fulkerson算法原理详解
- 10大人艰不拆的网络真相,你中了几枪?
- DSAPI多功能组件编程应用-HTTP监听服务端与客户端_指令版
- TCP/IP传输层,你懂多少?
- STM32W108无线ZigBee射频模块复位模块原理
- TCP/IP详解学习笔记——数据链路层(1)
- STM32W108无线ZigBee射频芯片引脚说明(下篇)