读Pyqt4教程,带你入门Pyqt4 _009
2015-06-15 18:44
260 查看
QLineEdit
QLineEdit 窗口组件用来输入或者编辑单行纯文本,有撤销/重做,剪切/粘贴和拖放功能。
该例子现在一个单行编辑器和一个标签。在单行编辑器中键入的文字会立即显示在标签中。
创建 QLineEdit
如果单行编辑器中的文本发生变化,调用 onChanged() 方法。
在 onChanged() 方法中,我们把输入的文字设置到标签中。并调用 adjustSize() 方法调整标签的尺寸为文本的长度。
QSplitter
QSplitter 使得用户可以通过拖动子窗口组件的边界来控制子窗口组件的尺寸。在我们的例子中,我们显示由两个分离器组织的三个 QFrame 窗口组件。
例子中有三个框架窗口组件和两个分离器。
为了看到 QFrame 之间的边界,我们使用带样式的框架。
使用 Cleanlooks 样式。 在某些样式中,框架是不可见的。
QComboBox
QComboBox 窗口组件允许用户从列表清单中选择。
这个例子中显示一个 QComboBox 和一个 QLabel 。组合框有5个选项的列表,他们是Linux发行版的名称。标签显示从组合框选择的内容。
创建一个 QComboBox 窗口组件并增加5个选项。
当一个选项被选择,我们调用 onActivated() 方法。
在该方法中,我们把选择项设置到标签中,并调整标签的尺寸。
在PyQt4教程的这部分中,我们涵盖了其他4个PyQt4窗口组件。
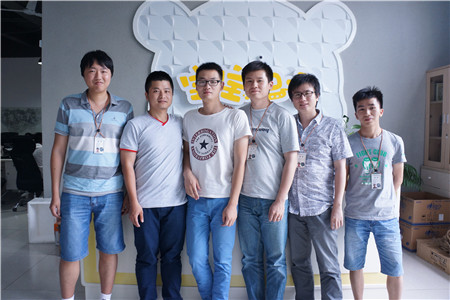
本站文章为 宝宝巴士 SD.Team 原创,转载务必在明显处注明:(作者官方网站: 宝宝巴士 )
转载自【宝宝巴士SuperDo团队】 原文链接: /article/6669079.html
QLineEdit 窗口组件用来输入或者编辑单行纯文本,有撤销/重做,剪切/粘贴和拖放功能。
#!/usr/bin/python # -*- coding: utf-8 -*- # ZetCode PyQt4 tutorial # # This example shows text which # is entered in a QLineEdit # in a QLabel widget. # # author: Jan Bodnar # website: zetcode.com # last edited: December 2010 from PyQt4 import QtGui from PyQt4 import QtCore class Example(QtGui.QWidget): def __init__(self, parent=None): QtGui.QWidget.__init__(self, parent) self.initUI() def initUI(self): self.label = QtGui.QLabel(self) edit = QtGui.QLineEdit(self) edit.move(60, 100) self.label.move(60, 40) self.connect(edit, QtCore.SIGNAL('textChanged(QString)'), self.onChanged) self.setWindowTitle('QLineEdit') self.setGeometry(250, 200, 350, 250) def onChanged(self, text): self.label.setText(text) self.label.adjustSize() def main(): app = QtGui.QApplication([]) exm = Example() exm.show() app.exec_() if __name__ == '__main__': main()
该例子现在一个单行编辑器和一个标签。在单行编辑器中键入的文字会立即显示在标签中。
edit = QtGui.QLineEdit(self)
创建 QLineEdit
self.connect(edit, QtCore.SIGNAL('textChanged(QString)'), self.onChanged)
如果单行编辑器中的文本发生变化,调用 onChanged() 方法。
def onChanged(self, text): self.label.setText(text) self.label.adjustSize()
在 onChanged() 方法中,我们把输入的文字设置到标签中。并调用 adjustSize() 方法调整标签的尺寸为文本的长度。
QSplitter
QSplitter 使得用户可以通过拖动子窗口组件的边界来控制子窗口组件的尺寸。在我们的例子中,我们显示由两个分离器组织的三个 QFrame 窗口组件。
#!/usr/bin/python # -*- coding: utf-8 -*- # ZetCode PyQt4 tutorial # # This example shows # how to use QSplitter widget. # # author: Jan Bodnar # website: zetcode.com # last edited: December 2010 from PyQt4 import QtGui, QtCore class Example(QtGui.QWidget): def __init__(self): super(Example, self).__init__() self.initUI() def initUI(self): hbox = QtGui.QHBoxLayout(self) topleft = QtGui.QFrame(self) topleft.setFrameShape(QtGui.QFrame.StyledPanel) topright = QtGui.QFrame(self) topright.setFrameShape(QtGui.QFrame.StyledPanel) bottom = QtGui.QFrame(self) bottom.setFrameShape(QtGui.QFrame.StyledPanel) splitter1 = QtGui.QSplitter(QtCore.Qt.Horizontal) splitter1.addWidget(topleft) splitter1.addWidget(topright) splitter2 = QtGui.QSplitter(QtCore.Qt.Vertical) splitter2.addWidget(splitter1) splitter2.addWidget(bottom) hbox.addWidget(splitter2) self.setLayout(hbox) self.setWindowTitle('QSplitter') QtGui.QApplication.setStyle(QtGui.QStyleFactory.create('Cleanlooks')) self.setGeometry(250, 200, 350, 250) def main(): app = QtGui.QApplication([]) ex = Example() ex.show() app.exec_() if __name__ == '__main__': main()
例子中有三个框架窗口组件和两个分离器。
topleft = QtGui.QFrame(self) topleft.setFrameShape(QtGui.QFrame.StyledPanel)
为了看到 QFrame 之间的边界,我们使用带样式的框架。
QtGui.QApplication.setStyle(QtGui.QStyleFactory.create('Cleanlooks'))
使用 Cleanlooks 样式。 在某些样式中,框架是不可见的。
QComboBox
QComboBox 窗口组件允许用户从列表清单中选择。
#!/usr/bin/python # -*- coding: utf-8 -*- # ZetCode PyQt4 tutorial # # In this example, we show how to # use the QComboBox widget. # # author: Jan Bodnar # website: zetcode.com # last edited: December 2010 from PyQt4 import QtGui, QtCore class Example(QtGui.QWidget): def __init__(self): super(Example, self).__init__() self.initUI() def initUI(self): self.label = QtGui.QLabel("Ubuntu", self) combo = QtGui.QComboBox(self) combo.addItem("Ubuntu") combo.addItem("Mandriva") combo.addItem("Fedora") combo.addItem("Red Hat") combo.addItem("Gentoo") combo.move(50, 50) self.label.move(50, 150) self.connect(combo, QtCore.SIGNAL('activated(QString)'), self.onActivated) self.setGeometry(250, 200, 350, 250) self.setWindowTitle('QComboBox') def onActivated(self, text): self.label.setText(text) self.label.adjustSize() def main(): app = QtGui.QApplication([]) ex = Example() ex.show() app.exec_() if __name__ == '__main__': main()
这个例子中显示一个 QComboBox 和一个 QLabel 。组合框有5个选项的列表,他们是Linux发行版的名称。标签显示从组合框选择的内容。
combo = QtGui.QComboBox(self) combo.addItem("Ubuntu") combo.addItem("Mandriva") combo.addItem("Fedora") combo.addItem("Red Hat") combo.addItem("Gentoo")
创建一个 QComboBox 窗口组件并增加5个选项。
self.connect(combo, QtCore.SIGNAL('activated(QString)'), self.onActivated)
当一个选项被选择,我们调用 onActivated() 方法。
def onActivated(self, text): self.label.setText(text) self.label.adjustSize()
在该方法中,我们把选择项设置到标签中,并调整标签的尺寸。
在PyQt4教程的这部分中,我们涵盖了其他4个PyQt4窗口组件。
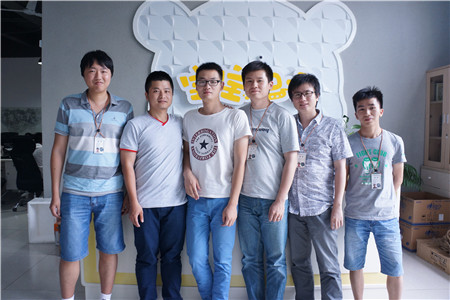
本站文章为 宝宝巴士 SD.Team 原创,转载务必在明显处注明:(作者官方网站: 宝宝巴士 )
转载自【宝宝巴士SuperDo团队】 原文链接: /article/6669079.html
相关文章推荐
- qml 嵌套到 qt的对话框上
- qt5 QString转char *
- 设置QTP脚本中每个步骤之间的延时时间
- Qt在windowsXp环境的安装和配置
- qt学习笔记001 2015/6/15
- vs2013下qt工程设置exe图标
- tiny4412 tslib ts_test显示界面,但触摸无反应,运行qt4命令触摸有反应
- QT QTableWidget不可编辑
- windows下 qt5&vs2010 在qtCreator下中文乱码
- win下使用PyQt的Phonon播放失败
- Mac Windows Qt5.4.x加载QMYSQL出错的原因
- QtPropertyBrowser 下载地址
- 在vs2013+qt中添加控制台
- Qt多线程
- QT相对路径显示图片
- Qt +vs发布
- Qt5.2连接SQLServer2008
- QT全局热键
- QT中PRO文件写法的详细介绍
- QT使用的积累(2015年7月3日更新)