二维码之qrencode生成(带logo)
2015-06-09 15:28
417 查看
从github下载的qrencode没有QRCodeGenerator文件,需要引入
在需要的文件中加入#import "QRCodeGenerator.h"即可
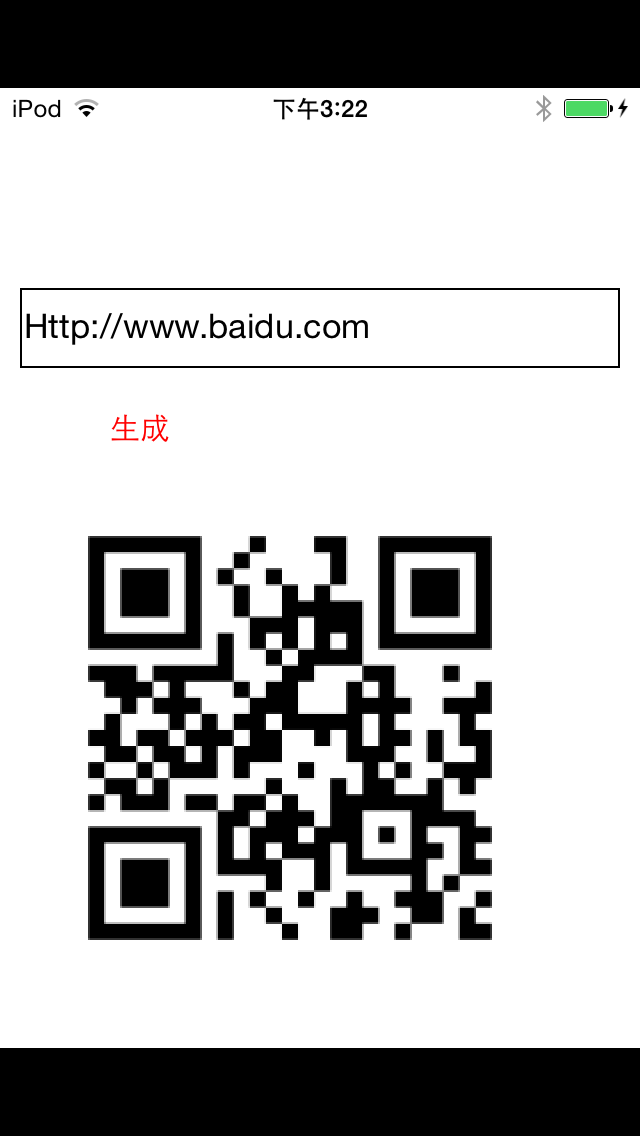
有时候想在二维码中间带个Logo,犹豫二维码有容错率,所以可以直接将图片添加上去
// // QR Code Generator - generates UIImage from NSString // // Copyright (C) 2012 http://moqod.com Andrew Kopanev <andrew@moqod.com> // // Permission is hereby granted, free of charge, to any person obtaining a copy // of this software and associated documentation files (the "Software"), to deal // in the Software without restriction, including without limitation the rights // to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies // of the Software, and to permit persons to whom the Software is furnished to do so, // subject to the following conditions: // // The above copyright notice and this permission notice shall be included in all // copies or substantial portions of the Software. // // THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, // INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR // PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE // FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR // OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER // DEALINGS IN THE SOFTWARE. // #import <Foundation/Foundation.h> #import <UIKit/UIKit.h> @interface QRCodeGenerator : NSObject + (UIImage *)qrImageForString:(NSString *)string imageSize:(CGFloat)size; @end
// // QR Code Generator - generates UIImage from NSString // // Copyright (C) 2012 http://moqod.com Andrew Kopanev <andrew@moqod.com> // // Permission is hereby granted, free of charge, to any person obtaining a copy // of this software and associated documentation files (the "Software"), to deal // in the Software without restriction, including without limitation the rights // to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies // of the Software, and to permit persons to whom the Software is furnished to do so, // subject to the following conditions: // // The above copyright notice and this permission notice shall be included in all // copies or substantial portions of the Software. // // THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, // INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR // PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE // FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR // OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER // DEALINGS IN THE SOFTWARE. // #import "QRCodeGenerator.h" #import "qrencode.h" enum { qr_margin = 3 }; @implementation QRCodeGenerator + (void)drawQRCode:(QRcode *)code context:(CGContextRef)ctx size:(CGFloat)size { unsigned char *data = 0; int width; data = code->data; width = code->width; float zoom = (double)size / (code->width + 2.0 * qr_margin); CGRect rectDraw = CGRectMake(0, 0, zoom, zoom); // draw CGContextSetFillColor(ctx, CGColorGetComponents([UIColor blackColor].CGColor)); for(int i = 0; i < width; ++i) { for(int j = 0; j < width; ++j) { if(*data & 1) { rectDraw.origin = CGPointMake((j + qr_margin) * zoom,(i + qr_margin) * zoom); CGContextAddRect(ctx, rectDraw); } ++data; } } CGContextFillPath(ctx); } + (UIImage *)qrImageForString:(NSString *)string imageSize:(CGFloat)size { if (![string length]) { return nil; } QRcode *code = QRcode_encodeString([string UTF8String], 0, QR_ECLEVEL_L, QR_MODE_8, 1); if (!code) { return nil; } // create context CGColorSpaceRef colorSpace = CGColorSpaceCreateDeviceRGB(); CGContextRef ctx = CGBitmapContextCreate(0, size, size, 8, size * 4, colorSpace, kCGImageAlphaPremultipliedLast); CGAffineTransform translateTransform = CGAffineTransformMakeTranslation(0, -size); CGAffineTransform scaleTransform = CGAffineTransformMakeScale(1, -1); CGContextConcatCTM(ctx, CGAffineTransformConcat(translateTransform, scaleTransform)); // draw QR on this context [QRCodeGenerator drawQRCode:code context:ctx size:size]; // get image CGImageRef qrCGImage = CGBitmapContextCreateImage(ctx); UIImage * qrImage = [UIImage imageWithCGImage:qrCGImage]; // some releases CGContextRelease(ctx); CGImageRelease(qrCGImage); CGColorSpaceRelease(colorSpace); QRcode_free(code); return qrImage; } @end
在需要的文件中加入#import "QRCodeGenerator.h"即可
// // ViewController.m // qRenCode // // Created by City--Online on 15/6/9. // Copyright (c) 2015年 CYW. All rights reserved. // #import "ViewController.h" #import "QRCodeGenerator.h" @interface ViewController () @property(nonatomic,strong) UITextField *textField; @property(nonatomic,strong) UIButton *btn; @property(nonatomic,strong) UIImageView *imgView; @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; _textField=[[UITextField alloc]initWithFrame:CGRectMake(10, 100, 300, 40)]; _textField.borderStyle=UITextBorderStyleLine; [self.view addSubview:_textField]; _btn=[UIButton buttonWithType:UIButtonTypeSystem]; [_btn setTitle:@"生成" forState:UIControlStateNormal]; [_btn setTitleColor:[UIColor redColor] forState:UIControlStateNormal]; _btn.frame=CGRectMake(20, 150, 100, 40); [_btn addTarget:self action:@selector(btnClick:) forControlEvents:UIControlEventTouchUpInside]; [self.view addSubview:_btn]; _imgView=[[UIImageView alloc]initWithFrame:CGRectMake(20, 200, 250, 250)]; // _imgView.backgroundColor=[UIColor blackColor]; [self.view addSubview:_imgView]; } -(void)btnClick:(id)sender { _imgView.image = [QRCodeGenerator qrImageForString:_textField.text imageSize:_imgView.bounds.size.width]; } -(void)touchesBegan:(NSSet *)touches withEvent:(UIEvent *)event { [_textField resignFirstResponder]; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } @end
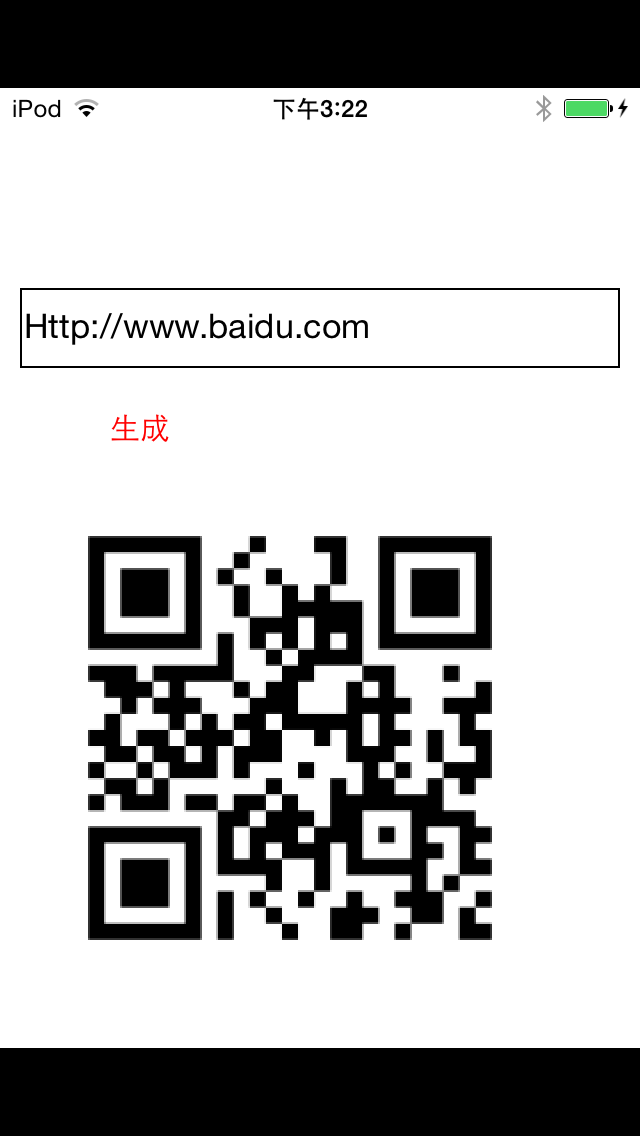
有时候想在二维码中间带个Logo,犹豫二维码有容错率,所以可以直接将图片添加上去
-(void)btnClick:(id)sender { _imgView.image = [QRCodeGenerator qrImageForString:_textField.text imageSize:_imgView.bounds.size.width]; UIImageView *img=[[UIImageView alloc]initWithImage:[UIImage imageNamed:@"1.jpg"]]; img.frame=CGRectMake(_imgView.bounds.size.width*3/7, _imgView.bounds.size.height*3/7, _imgView.bounds.size.width/7, _imgView.bounds.size.height/7); img.backgroundColor=[UIColor redColor]; [_imgView addSubview:img]; }

相关文章推荐
- mac修改host文件,让你的mac轻松上google
- golang语法学习(一):变量,常量以及数据类型
- golang vim ide 环境搭建
- STL学习笔记之算法--algorithmn
- django学习笔记
- Goroutine + Channel 实践
- 关于Go语言共享内存操作的小实例
- 算法导论习题-1.2-2
- POJ3623:Best Cow Line, Gold(后缀数组)
- Web开发与设计之Google兵器谱-Web开发与设计利器
- Ubuntu下的Juju现在支持systemd和Google Cloud Platform了
- LightOJ 1030 Discovering Gold【概率】
- Django笔记
- go语言实现的目录共享程序
- Django学习(七) 创建第一个Django项目
- 2、Django中模板的使用
- BestCoder Round #43 (hdu5264 - 5266)(数学,LCA)good
- django 中 manage.py通常使用的各种命令大全(包含django 安装指导及测试)
- django使用celery实现异步操作
- Kolmogorov复杂度(转)