基于数组实现Java 自定义Stack栈类及应用
2015-06-09 00:02
501 查看
栈是存放对象的一种特殊容器,在插入与删除对象时,这种结构遵循后进先出( Last-in-first-out,LIFO)的原则。java本身是有自带Stack类包,为了达到学习目的已经更好深入了解stack栈,自己动手自建java stack类是个很好的学习开始:
自定义ExceptionStackEmpty异常类(关于如何自定义异常类可以看相关博客)
自定义ExceptionStackFull异常类
测试类:
测试结果:
假设算术表达式为 X = “x0x1x2…xn-1”,其中 xi 可以是括号、常数、变量名或者算术运算符。我们依次检查 X 中的各个符号,非括号的符号都可以忽略。若遇到左括号,则将其压入栈 S 中;若遇到右括号,则将栈顶符号弹出并与该右括号对比。如果发现某对括号不匹配,或者遇到右括号时栈为空,或者整个表达式扫描过后栈非空,都可以断定括号不匹配。
在按照以上规则扫描完所有字符后,若栈为空,则说明括号是匹配的。如果按照前面对栈的实现,每一 push()和 pop()操作都只需常数时间,因此对于长度为 n 的算术表达式,上述算法需要运行 O(n)的时间。
该算法的伪代码描述如 算法二.1 所示:
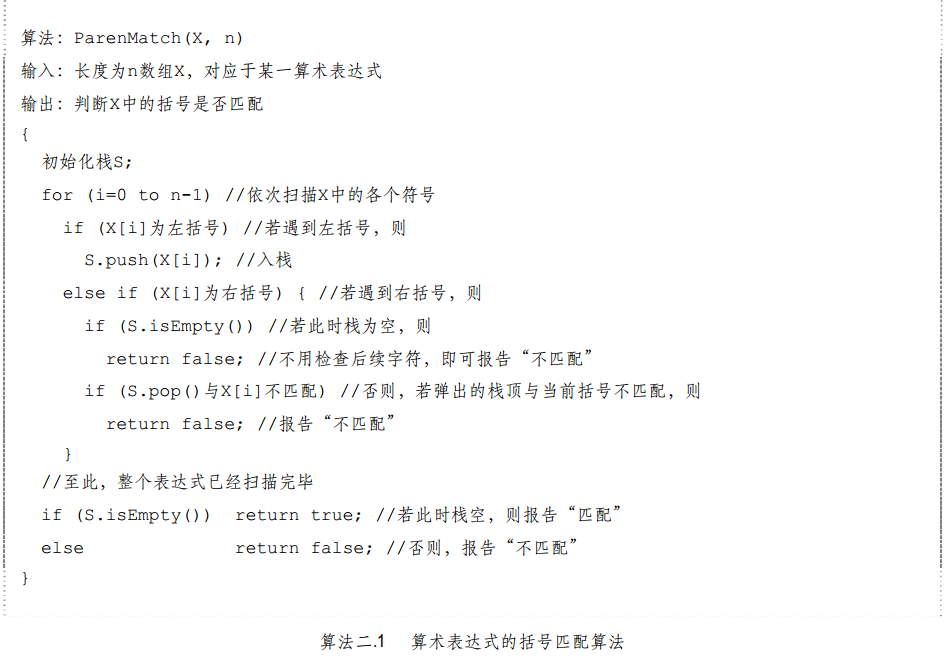
测试结果:
文章参考:数据结构与算法( Java 描述)邓俊辉 著
转载请注明出处,谢谢!
http://blog.csdn.net/github_27609763/article/details/46420149
自建Java Stack 类
Stack 类:package com.stack; import java.util.ArrayList; import java.util.Arrays; /** * Stack Class * @author ganyee * */ public class Stack { //Define capacity constant:CAPACITY private static final int CAPACITY = 1024; //Define capacity private static int capacity; //Define the top position of stack //top = -1 meaning that the stack empty private static int top = -1; //Basic Object class array Object[] array; //Initialize the capacity of stack public Stack() { this.capacity = CAPACITY; array = new Object[capacity]; } //Get the size of stack public int getSize(){ if(isEmpty()){ return 0; }else{ return top + 1; } } //Get whether stack is empty public boolean isEmpty(){ return (top < 0); } //Get the top element of stack public Object top() throws ExceptionStackEmpty{ if(isEmpty()){ throw new ExceptionStackEmpty("Stack is empty"); } return array[top]; } //Push element to stack public void push(Object element) throws ExceptionStackFull{ if(getSize()== CAPACITY){ throw new ExceptionStackFull("Stack is full"); } array[++ top] = element; } //Pop element from stack public Object pop() throws ExceptionStackEmpty{ if(isEmpty()){ throw new ExceptionStackEmpty("Stack is empty"); } return array[top --]; } //Get the all elements of stack public String getAllElements() throws ExceptionStackEmpty{ String[] arr = new String[top + 1]; if(!isEmpty()){ for(int i = 0;i < getSize();i ++){ arr[i] = (String)array[i]; } } return Arrays.toString(arr); } }
自定义ExceptionStackEmpty异常类(关于如何自定义异常类可以看相关博客)
package com.stack; public class ExceptionStackEmpty extends Exception { //Constructor public ExceptionStackEmpty(){ } //Define myself exception construct with parameters public ExceptionStackEmpty(String string){ super(string); } }
自定义ExceptionStackFull异常类
package com.stack; public class ExceptionStackFull extends Exception { //Constructor public ExceptionStackFull(){ } //Define myself exception construct with parameters public ExceptionStackFull(String string){ super(string); } }
测试类:
package com.stack; public class StackTest { public static void main(String[] args) { // TODO Auto-generated method stub Stack stack= new Stack(); System.out.println(stack.getSize()); System.out.println(stack.isEmpty()); try { stack.push(8); stack.push(3); stack.push(4); stack.push(7); stack.push(1); stack.push(8); stack.push(3); stack.push(4); stack.push(7); stack.push(1); System.out.println(stack.getSize()); System.out.println(stack.top()); System.out.println(stack.getAllElements()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); System.out.println(stack.pop()); } catch (ExceptionStackFull e) { // TODO Auto-generated catch block e.printStackTrace(); }catch (ExceptionStackEmpty e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
测试结果:
0 true 10 1 [8, 3, 4, 7, 1, 8, 3, 4, 7, 1] 1 7 4 3 8 1 7 4 3 8
栈的应用:符号匹配
下面,我们将借助一个栈结构 S,通过对算术表达式自左向右的一遍扫描,检查其中的括号是否匹配。假设算术表达式为 X = “x0x1x2…xn-1”,其中 xi 可以是括号、常数、变量名或者算术运算符。我们依次检查 X 中的各个符号,非括号的符号都可以忽略。若遇到左括号,则将其压入栈 S 中;若遇到右括号,则将栈顶符号弹出并与该右括号对比。如果发现某对括号不匹配,或者遇到右括号时栈为空,或者整个表达式扫描过后栈非空,都可以断定括号不匹配。
在按照以上规则扫描完所有字符后,若栈为空,则说明括号是匹配的。如果按照前面对栈的实现,每一 push()和 pop()操作都只需常数时间,因此对于长度为 n 的算术表达式,上述算法需要运行 O(n)的时间。
该算法的伪代码描述如 算法二.1 所示:
package com.stack; public class MatchClass { public static boolean Match(String str) throws ExceptionStackFull, ExceptionStackEmpty{ Stack stack = new Stack(); str = str.replaceAll(" ",""); char s; for(int i = 0;i < str.length();i ++){ if(str.charAt(i) == '(' || str.charAt(i) == '{' || str.charAt(i) == '[') stack.push(str.charAt(i)); else{ if(stack.isEmpty()) return false; else{ s = str.charAt(i); switch(s){ case ')': if((Character)stack.pop() != '(') return false; break; case '}': if((Character)stack.pop() != '{') return false; break; case ']': if((Character)stack.pop() != '[') return false; break; } } } } if(stack.isEmpty()){ return true; }else{ return false; } } }
package com.stack; public class ParentMatch { public static void main(String[] args) { MatchClass match = new MatchClass(); //String str = "()({})"; //Match //String str = "()({}) {([()[]])}";//Match //String str = "([]{)";//Not match //String str = ")([()] {}";//Not match String str = "([())]{}";//Not match try { if(!match.Match(str)){ System.out.println(str + ": Not Macth"); }else{ System.out.println(str + ": Macth"); } } catch (ExceptionStackFull e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (ExceptionStackEmpty e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
测试结果:
()({}): Macth ()({}) {([()[]])}: Macth ([]{): Not Macth )([()] {}: Not Macth ([())]{}: Not Macth
文章参考:数据结构与算法( Java 描述)邓俊辉 著
转载请注明出处,谢谢!
http://blog.csdn.net/github_27609763/article/details/46420149
相关文章推荐
- java对世界各个时区(TimeZone)的通用转换处理方法(转载)
- java-注解annotation
- java-模拟tomcat服务器
- java-用HttpURLConnection发送Http请求.
- java-WEB中的监听器Lisener
- Android IPC进程间通讯机制
- Android Native 绘图方法
- Android java 与 javascript互访(相互调用)的方法例子
- 介绍一款信息管理系统的开源框架---jeecg
- 聚类算法之kmeans算法java版本
- java实现 PageRank算法
- PropertyChangeListener简单理解
- 插入排序
- 冒泡排序
- 堆排序
- 快速排序
- 二叉查找树
- [原创]java局域网聊天系统