《数据结构》实验二: 线性表实验
2014-12-29 17:01
323 查看
一..实验目的
巩固线性表的数据结构,学会线性表的应用。
1.回顾线性表的逻辑结构,线性表的物理存储结构和常见操作。
2.学习运用线性表的知识来解决实际问题。
3.进一步巩固程序调试方法。
4.进一步巩固模板程序设计。
二.实验时间
准备时间为第2周到第4周,具体集中实验时间为第4周第2次课。2个学时。
三..实验内容
1.建立一个N个学生成绩的顺序表,对表进行插入、删除、查找等操作。分别输出结果。
要求如下:
1)用顺序表来实现。
#include<iostream>
using namespace std;
const int Maxsize=100;
class Score
{
public:
Score(){length=0;}
Score(int a[],int n);
~Score(){ }
int Locate(int x);
void Insert(int i,int x);
int Delete(int i);
void Printlist();
private:
int data[Maxsize];
int length;
};
Score::Score(int a[],int n)
{
if(n>Maxsize) throw"参数非法";
for(int i=0;i<n;i++)
data[i]=a[i];
length=n;
}
void Score::Insert(int i,int x)
{
if(length>=Maxsize) throw"上溢";
if(i<1||i>length+1) throw"位置非法";
for(int j=length;j>=i;j--)
data[j]=data[j-1];
data[i-1]=x;
length++;
}
int Score::Delete(int i)
{
if(length==0) throw"下溢";
if(i<1||i>length) throw"位置非法";
int x=data[i-1];
for(int j=i;j<length;j++)
dat
4000
a[j-1]=data[j];
length--;
return x;
}
int Score::Locate(int x)
{
for(int i=0;i<length;i++)
if(data[i]==x) return i+1;
return 0;
}
void Score::Printlist()
{
for(int i=0;i<length;i++)
cout<<data[i]<<" ";
cout<<endl;
}
void main()
{
int r[10]={83,85,74,71,67,73,66,84,100,99};
Score L(r,10);
cout<<"执行插入操作的前的数据为:"<<endl;
L.Printlist();
try
{
L.Insert(4,55);
}
catch(char *s)
{
cout<<s<<endl;
}
int d;
cout<<"执行插入操作后的数据为:"<<endl;
L.Printlist();
cout<<"请输入所查询的位置"<<endl;
cin>>d;
cout<<"值为"<<d<<"的元素位置为:";
cout<<L.Locate(d)<<endl;
cout<<"执行删除第一个元素操作,删除前数据为:"<<endl;
L.Printlist();
try
{
L.Delete(1);
}
catch(char *s)
{
cout<<s<<endl;
}
cout<<"删除后的数据为:"<<endl;
L.Printlist();
}
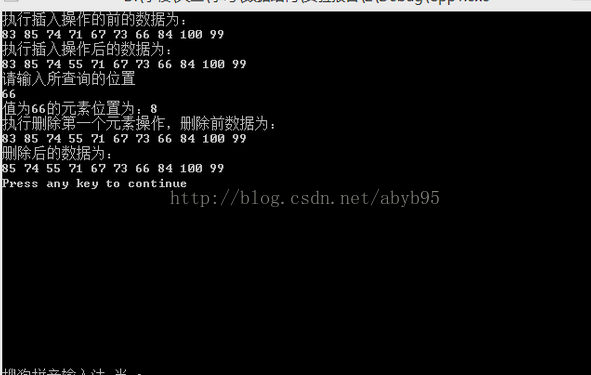
2)用单链表来实现。
#include<iostream>
using namespace std;
template<class Score>
struct Node
{
Score data;
Node<Score> * next;
};
template<class Score>
class Linklist
{
public:
Linklist();
Linklist(Score a[],int n);
~Linklist();
int Locate(Score x);
void Insert(int i,Score x);
Score Delete(int i);
void Printlist();
private:
Node<Score> * first;
};
template<class Score>
Linklist<Score>::Linklist()
{
first=new Node<Score>;
first->next=NULL;
}
template<class Score>
Linklist<Score>::Linklist(Score a[],int n)
{
Node<Score> *r,*s;
first=new Node<Score>;
r=first;
for(int i=0;i<n;i++)
{
s=new Node<Score>;
s->data=a[i];
r->next=s;r=s;
}
r->next=NULL;
}
template<class Score>
Linklist<Score>::~Linklist()
{
Node<Score>*q=NULL;
while(first!=NULL)
{
q=first;
first=first->next;
delete q;
}
}
template<class Score>
void Linklist<Score>::Insert(int i,Score x)
{
Node<Score>*p=first,*s=NULL;
int count=0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL)throw"位置";
else{
s=new Node<Score>;s->data=x;
s->next=p->next;p->next=s;
}
}
template<class Score>
Score Linklist<Score>::Delete(int i)
{
Node<Score>*p=first,*q=NULL;
Score x;
int count =0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL||p->next==NULL)
throw"位置";
else{
q=p->next;
x=q->data;
p->next=q->next;
delete q;
return x;
}
}
template<class Score>
int Linklist<Score>::Locate(Score x)
{
Node<Score>*p=first->next;
int count=1;
while(p!=NULL)
{
if(p->data==x) return count;
p=p->next;
count++;
}
return 0;
}
template<class Score>
void Linklist<Score>::Printlist()
{
Node<Score>*p=first->next;
while(p!=NULL)
{
cout<<p->data<<" ";
p=p->next;
}
cout<<endl;
}
void main()
{
int r[10]={72,49,82,61,83,100,98,70,73,84};
Linklist<int>L(r,10);
cout<<"执行插入操作前的数据为:"<<endl;
L.Printlist();
try
{
L.Insert(3,89);
}
catch(char *s)
{
cout<<s<<endl;
}
cout<<"执行插入操作后的数据为:"<<endl;
L.Printlist();
cout<<"输入要查找的元素"<<endl;
int d;
cin>>d;
cout<<"值为"<<d<<"的元素的位置为:";
cout<<L.Locate(d)<<endl;
cout<<"执行删除操作前的数据为:"<<endl;
L.Printlist();
try
{
L.Delete(1);
}
catch(char *s)
{
cout<<s<<endl;
}
cout<<"执行删除操作后的数据为:"<<endl;
L.Printlist();
}
巩固线性表的数据结构,学会线性表的应用。
1.回顾线性表的逻辑结构,线性表的物理存储结构和常见操作。
2.学习运用线性表的知识来解决实际问题。
3.进一步巩固程序调试方法。
4.进一步巩固模板程序设计。
二.实验时间
准备时间为第2周到第4周,具体集中实验时间为第4周第2次课。2个学时。
三..实验内容
1.建立一个N个学生成绩的顺序表,对表进行插入、删除、查找等操作。分别输出结果。
要求如下:
1)用顺序表来实现。
#include<iostream>
using namespace std;
const int Maxsize=100;
class Score
{
public:
Score(){length=0;}
Score(int a[],int n);
~Score(){ }
int Locate(int x);
void Insert(int i,int x);
int Delete(int i);
void Printlist();
private:
int data[Maxsize];
int length;
};
Score::Score(int a[],int n)
{
if(n>Maxsize) throw"参数非法";
for(int i=0;i<n;i++)
data[i]=a[i];
length=n;
}
void Score::Insert(int i,int x)
{
if(length>=Maxsize) throw"上溢";
if(i<1||i>length+1) throw"位置非法";
for(int j=length;j>=i;j--)
data[j]=data[j-1];
data[i-1]=x;
length++;
}
int Score::Delete(int i)
{
if(length==0) throw"下溢";
if(i<1||i>length) throw"位置非法";
int x=data[i-1];
for(int j=i;j<length;j++)
dat
4000
a[j-1]=data[j];
length--;
return x;
}
int Score::Locate(int x)
{
for(int i=0;i<length;i++)
if(data[i]==x) return i+1;
return 0;
}
void Score::Printlist()
{
for(int i=0;i<length;i++)
cout<<data[i]<<" ";
cout<<endl;
}
void main()
{
int r[10]={83,85,74,71,67,73,66,84,100,99};
Score L(r,10);
cout<<"执行插入操作的前的数据为:"<<endl;
L.Printlist();
try
{
L.Insert(4,55);
}
catch(char *s)
{
cout<<s<<endl;
}
int d;
cout<<"执行插入操作后的数据为:"<<endl;
L.Printlist();
cout<<"请输入所查询的位置"<<endl;
cin>>d;
cout<<"值为"<<d<<"的元素位置为:";
cout<<L.Locate(d)<<endl;
cout<<"执行删除第一个元素操作,删除前数据为:"<<endl;
L.Printlist();
try
{
L.Delete(1);
}
catch(char *s)
{
cout<<s<<endl;
}
cout<<"删除后的数据为:"<<endl;
L.Printlist();
}
2)用单链表来实现。
#include<iostream>
using namespace std;
template<class Score>
struct Node
{
Score data;
Node<Score> * next;
};
template<class Score>
class Linklist
{
public:
Linklist();
Linklist(Score a[],int n);
~Linklist();
int Locate(Score x);
void Insert(int i,Score x);
Score Delete(int i);
void Printlist();
private:
Node<Score> * first;
};
template<class Score>
Linklist<Score>::Linklist()
{
first=new Node<Score>;
first->next=NULL;
}
template<class Score>
Linklist<Score>::Linklist(Score a[],int n)
{
Node<Score> *r,*s;
first=new Node<Score>;
r=first;
for(int i=0;i<n;i++)
{
s=new Node<Score>;
s->data=a[i];
r->next=s;r=s;
}
r->next=NULL;
}
template<class Score>
Linklist<Score>::~Linklist()
{
Node<Score>*q=NULL;
while(first!=NULL)
{
q=first;
first=first->next;
delete q;
}
}
template<class Score>
void Linklist<Score>::Insert(int i,Score x)
{
Node<Score>*p=first,*s=NULL;
int count=0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL)throw"位置";
else{
s=new Node<Score>;s->data=x;
s->next=p->next;p->next=s;
}
}
template<class Score>
Score Linklist<Score>::Delete(int i)
{
Node<Score>*p=first,*q=NULL;
Score x;
int count =0;
while(p!=NULL&&count<i-1)
{
p=p->next;
count++;
}
if(p==NULL||p->next==NULL)
throw"位置";
else{
q=p->next;
x=q->data;
p->next=q->next;
delete q;
return x;
}
}
template<class Score>
int Linklist<Score>::Locate(Score x)
{
Node<Score>*p=first->next;
int count=1;
while(p!=NULL)
{
if(p->data==x) return count;
p=p->next;
count++;
}
return 0;
}
template<class Score>
void Linklist<Score>::Printlist()
{
Node<Score>*p=first->next;
while(p!=NULL)
{
cout<<p->data<<" ";
p=p->next;
}
cout<<endl;
}
void main()
{
int r[10]={72,49,82,61,83,100,98,70,73,84};
Linklist<int>L(r,10);
cout<<"执行插入操作前的数据为:"<<endl;
L.Printlist();
try
{
L.Insert(3,89);
}
catch(char *s)
{
cout<<s<<endl;
}
cout<<"执行插入操作后的数据为:"<<endl;
L.Printlist();
cout<<"输入要查找的元素"<<endl;
int d;
cin>>d;
cout<<"值为"<<d<<"的元素的位置为:";
cout<<L.Locate(d)<<endl;
cout<<"执行删除操作前的数据为:"<<endl;
L.Printlist();
try
{
L.Delete(1);
}
catch(char *s)
{
cout<<s<<endl;
}
cout<<"执行删除操作后的数据为:"<<endl;
L.Printlist();
}
相关文章推荐
- 数据结构第三次实验——线性表专题在线评测
- 《数据结构》实验二: 线性表实验
- 《数据结构》实验二: 线性表实验
- 《数据结构》实验二: 线性表综合实验——总结线性表的几种主要存储结果
- 数据结构线性表的综合实验——单链表
- 《数据结构》实验二: 线性表实验
- 《数据结构》实验二: 线性表实验--------------第一题
- 《数据结构》实验二: 线性表综合实验——总结线性表的几种主要存储结果
- 《数据结构》实验二: 线性表实验(下)
- 数据结构线性表的综合实验——顺序表
- 《数据结构》实验一: VC编程工具的灵活使用
- 数据结构(22)循环队列--线性表实现
- 数据结构上机实验之顺序查找
- [考研系列之数据结构]线性表之字符串
- 数据结构-线性表
- javascript数据结构系列(一)-线性表
- Java数据结构--线性表
- [数据结构]第二章--线性表(读书笔记4)
- 数据结构--顺序线性表
- 数据结构 【实验9 图的基本操作】