《Linux程序设计》第四版第二章 我的第一个shell程序:CD唱片应用程序
2014-10-15 22:43
253 查看
1. 书籍《Linux程序设计》第四版 Neil Mattew,Richard Stones著,第二章中书中用shell写了一个CD 唱片程序,仿照它,我自己大概实现了其功能,代码如下:
文件:manageCd.sh
2. 运行:
shell文件:manageCd.sh,保存唱片的文件:title_file,保存每个唱片对应歌曲的文件:songs_file
示例如下:
title_file: 各字段分别为:唱片名,标题,曲目类型,作曲家
CDname3,cool sax,type3,Bix
CDname2,classsic violin,type2,bach
songs_file:各字段分别为:唱片名,曲目编号,曲名
CDname2,1,good
CDname3,1,gsdf
CDname3,2,sff
截图:
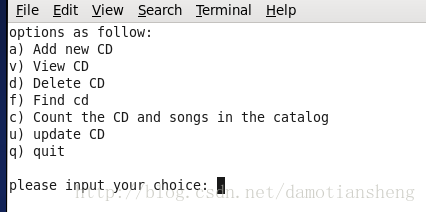
功能:添加唱片,查看唱片,删除唱片,查找唱片,统计唱片和更新唱片
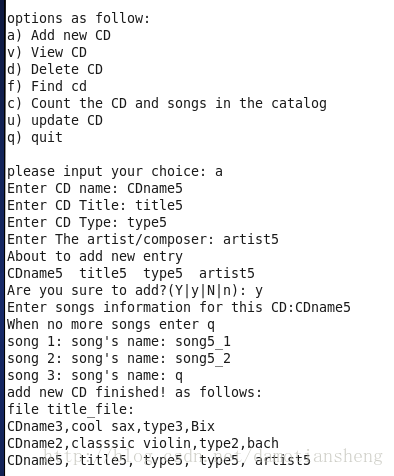
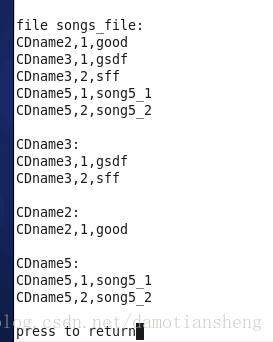
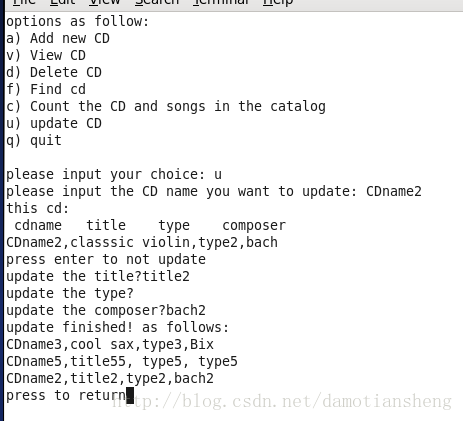
文件:manageCd.sh
#!/bin/bash # this program aims to manage CD and songs contained in the CD # history: # 2014-10-14 first release # manageCd.sh mainBoard() { choice=1 until [ $choice == "q" ] do clear echo "options as follow:" echo "a) Add new CD" echo "v) View CD" echo "d) Delete CD" echo "f) Find cd" echo "c) Count the CD and songs in the catalog" echo "u) update CD" echo "q) quit" echo "" read -p "please input your choice: " choice while [ "$choice" != "a" -a "$choice" != "v" -a "$choice" != "d" -a "$choice" != "f" -a "$choice" != "c" -a "$choice" != "u" -a "$choice" != "q" ] do read -p "input error,please input again([a,v,d,f,c,u]):" choice done case $choice in "a") addNewCD ;; "v") viewCD ;; "d") deleteCD ;; "f") findCD ;; "c") countCDAndSongs ;; "u") updateCD ;; "*") echo "error" ;; esac echo -n "press to return" read x done } addNewCD() { title_file="title_file" songs_file="songs_file" read -p "Enter CD name: " name while true do flag=0 for x in `awk -F , '{print $1}' $title_file` do if [ "$name" == "$x" ]; then echo -n "CD name:$name has existed,please input CD name again: " read name flag=1 break; fi done if [ "$flag" == 0 ]; then break; fi done read -p "Enter CD Title: " title read -p "Enter CD Type: " type read -p "Enter The artist/composer: " composer echo "About to add new entry" echo "$name $title $type $composer" read -p "Are you sure to add?(Y|y|N|n): " choice while [ $choice != "y" -a $choice != "Y" -a $choice != "N" -a $choice != "n" ] do read -p "input error! please input again(Y,y,N,n): " choice done if [ $choice == "n" ]; then echo "add cancelled" return 1 fi if [ -f $title_file ]; then flag=1 else flag=0 fi if [ $flag == "1" ]; then #file exists echo "$name, $title, $type, $type, $composer" >> $title_file else touch $title_file #create file echo "$name, $title, $type, $type, $composer" > $title_file fi num=1 echo "Enter songs information for this CD:$name" echo "When no more songs enter q" while true do echo -n "song $num: song's name: " while true do read songsname if [ "$songsname" == "" ]; then read -p "empty name,please input song's name again: " songsname else break; fi done if [ "$songsname" == "q" ]; then echo "add new CD finished! as follows: " viewCD return 0 fi [ ! -f "$songs_file" ] && echo > "$songs_file" #to create empty file echo "$name,$num,$songsname" >> "$songs_file" num=`expr $num + 1` # add 1 done } viewCD() { songs_file="songs_file" title_file="title_file" echo "file $title_file: " if test -f "$title_file"; then cat "$title_file" | more else echo "empty" return 1 fi echo "" echo "file $songs_file: " [ -f "$songs_file" ] && cat "$songs_file" | more [ ! -f "$songs_file" ] && echo "empty" && return 1 echo "" #awk -F , '{print $1}' "$title_file" #get the first row seperated by , for x in `awk -F , '{print $1}' "$title_file"` do echo "$x: " && grep "$x" "$songs_file" echo "" done # read -p "input to return" abc return 0 } deleteCD() { title_file="title_file" songs_file="songs_file" read -p "please input CD name: " name while [ "$name" == "" ] do read -p "no input,please input again: " name done echo "will delete CD $name..." getConfirm && { if grep "$name" $title_file; then grep -v "$name" $title_file > tmpfile mv tmpfile $title_file grep -v "$name" $songs_file > tmpfile mv tmpfile $songs_file echo "delete CD name:$name finished!" echo "CD contents:" viewCD #viewSongs fi } return 0 } findCD() { title_file="title_file" songs_file="songs_file" echo -n "please input CD name which you want to find: " read cdname while true do if [ "$cdname" == "" ]; then echo -n "your input is empty,please input cd name again: " read cdname else break fi done [ ! -f "$title_file" ] && echo "no this cd: $cdname" && return 1 flag=0 for x in `awk -F , '{print $1}' $title_file` do if [ "$cdname" == "$x" ]; then flag=1 grep "$cdname" $title_file echo "songs in $cdname: " [ -f "$songs_file" ] && grep "$cdname" $songs_file && flag=2 [ ! -f "$songs_file" ] && echo "this cd has no songs" && flag=3 # [ ! grep "$cdname" $songs_file ] && echo "this cd has no songs" && flag=3 break; fi done if [ "$flag" == "0" ]; then echo "no this cd: $cdname" fi } getConfirm() { echo -n "Are you sure?" while true do read x case "$x" in y|Y|yes|YES|Yes) return 0;; n|N|no|NO|No) echo "cancelled" return 1;; *) echo "please input yes or no";; esac done } countCDAndSongs() { title_file="title_file" songs_file="songs_file" cdnum=0 songnum=0 for x in `awk -F , '{print $1}' $title_file` do cdnum=`expr $cdnum + 1` # add 1 done for x in `awk -F , '{print $1}' $songs_file` do songnum=`expr $songnum + 1` # add 1 done echo "find $cdnum CDs,with a total of $songnum songs" return 0 } updateCD() { title_file="title_file" songs_file="songs_file" read -p "please input the CD name you want to update: " cdname while true do if [ "$cdname" == "" ]; then echo -n "empty input, please input again: " read cdname else break fi done flag=0 for x in `awk -F , '{print $1}' $title_file` do if [ "$cdname" == "$x" ]; then flag=1 echo "this cd: " echo " cdname title type composer" grep "$cdname" $title_file echo "press enter to not update" read -p "update the title?" cdtitle read -p "update the type?" cdtype read -p "update the composer?" cdartist if [ "$cdtitle" == "" -o "$cdtype" == "" -o "$cdartist" == "" ]; then if [ "$cdtitle" == "" ]; then cdtitle=`grep "$cdname" $title_file | awk -F , '{print $2}'` fi if [ "$cdtype" == "" ]; then cdtype=`grep "$cdname" $title_file | awk -F , '{print $3}'` fi if [ "$cdartist" == "" ]; then cdartist=`grep "$cdname" $title_file | awk -F , '{print $4}'` fi fi grep -v "$cdname" $title_file > tmpfile echo "$cdname,$cdtitle,$cdtype,$cdartist" >> tmpfile mv tmpfile $title_file echo "update finished! as follows: " cat $title_file | more break fi done if [ "$flag" == "0" ]; then echo "no this cd: $cdname" fi } mainBoard
2. 运行:
shell文件:manageCd.sh,保存唱片的文件:title_file,保存每个唱片对应歌曲的文件:songs_file
示例如下:
title_file: 各字段分别为:唱片名,标题,曲目类型,作曲家
CDname3,cool sax,type3,Bix
CDname2,classsic violin,type2,bach
songs_file:各字段分别为:唱片名,曲目编号,曲名
CDname2,1,good
CDname3,1,gsdf
CDname3,2,sff
截图:
功能:添加唱片,查看唱片,删除唱片,查找唱片,统计唱片和更新唱片
相关文章推荐
- shell CD唱片应用程序
- 第二章 编写第一个JAVA程序
- 我的第一个shell程序
- 第二章 Shell程序设计
- 第一个shell程序:hello world
- SHELL 编程入门与提高(一)第一个shell程序
- 我的第一个shell程序
- 第一个shell程序
- 创建第一个 Word 应用程序级外接程序
- Spring flex 搭建服务 之 编程环境配置和我的第一个程序(第二章,第二节)
- 第一个shell程序----压缩android源码
- (第四版中文版)[十六]读和写文档-SDI应用程序:序列化,双击运行程序,拖放运行,快捷方式的实现
- 第二章 第二节 Linux设备驱动程序之--我的第一个程序 Hello World
- Linux程序设计-学习笔记-第二章shell程序设计
- Linux程序设计读书笔记:第二章 shell程序设计
- 从零开始Android游戏编程(第二版) 第二章 创建第一个程序Hello Tank
- 我的第一个shell程序
- 创建您的第一个 Word 应用程序级外接程序
- 第一个shell程序
- 第二章 shell程序设计