C++中 auto自动变量,命名空间,using作用以及作用域
2014-08-13 23:58
260 查看
1.auto关键字的用途
A:自动变量,可以自动获取类型,输出,类似泛型
B:自动变量,可以实现自动循环一维数组
C:自动循环的时候,对应的必须是常量
2.auto自动变量,自动匹配类型的案例如下:
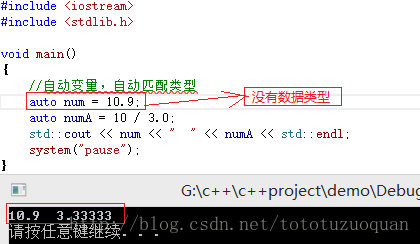
注意:如果是在QT下运行上面的的程序需要加上C++11的相关配置(CONFIG += C++11)
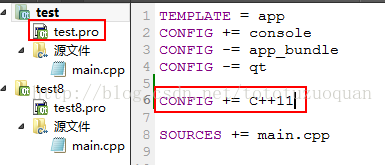
3.通过auto关键字自动循环一维数组的案例
#include<iostream>
#include<stdlib.h>
#include<iomanip>
usingnamespacestd;
voidmain()
{
//定义一维数组,下面的数组名是一个指针常量
intnum[10]
= { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
//通过循环遍历出数组
for
(autodata
:num)
{
cout
<<setw(5) <<data;
}
cout
<<endl;
//当变成二维数组之后,不能直接通过auto来输出
//auto自动循环begin endl;必须是一个数组的常量
doublenum2[2][5]
= { 1.0, 2.0, 3.0, 4.5, 5, 6, 7, 8, 9, 10 };
for
(autodata
:num2)//泛型C++语法,循环一维数组,是个常量
{
cout
<<data <<std::endl;
for
(inti
= 0;i < 5;i++)
{
std::cout<<setw(5)
<< *(data +i);
}
cout
<<endl;
}
system("pause");
}
运行结果如下:
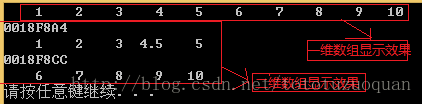
4.关于头文件
在C++中,为了区分C++,C++中不使用.h文件头
5.C++注重类型,它是一种强类型语言,严格检查类型。
6.C++中的输出函数,宽字符输出,赋初值的方式,输出符,::域控制符
#include<iostream> //在C++中,为了区分C++,C++中不使用.h文件头
#include<stdlib.h>
usingnamespacestd;
voidmain()
{
inta
= 5;
//C++中赋值:A变量名(数值) B:变量名=数值,通过这两种方式。
intb(5);
cout
<<a <<"
" <<b
<<endl;
//通过括号赋值
doublec(3.5);
cout
<<c <<endl;
//通过等号赋值
char
*pStr ="1234";
cout
<< *pStr <<"
" <<pStr
<<endl;
//通过括号赋值
char
*str("china");
cout
<< *str <<"
" <<str
<<endl;
//宽字符,汉子,棒子文
wchar_t
*str2(L"china");
wcout
<< *str2 <<"
" <<str2
<<endl;
system("pause");
}
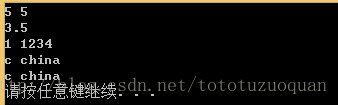
#include<iostream>
voidmain()
{
//输出符
<<
//std命名空间
//::域控制符
std::cout
<< "hello world";
system("pause");
}
7.一个文件中使用另外一个文件中声明的变量的时候,C++要求显示的加上extern关键字调用这个全局变量。
8.调用命名空间中的变量已经命名空间中的函数
A:有名命名空间
#include"iostream"
#include"stdlib.h"
namespacemyspace
{
inta(15);
voidprint()
{
std::cout
<< "锄禾日当午"
<< std::endl;
}
}
namespacemyspaceA
{
inta(25);
voidprint()
{
std::cout
<< "AAAAAAAAAAAA" <<std::endl;
}
}
voidmain()
{
inta(5);
//如果想不被上面的a变量影响,而直接访问命名空间
//中的变量,这里要加上命名空间名
std::cout
<< "myspace a = " <<myspace::a
<< std::endl;
//调用myspaceA中定义的变量a
std::cout
<< "myspaceA a = " <<myspaceA::a
<< std::endl;
std::cout
<< "main a = " <<a
<<std::endl;
std::cout
<< std::endl;
//调用命名空间中的函数
myspace::print();
myspaceA::print();
system("pause");
}
运行结果如下:
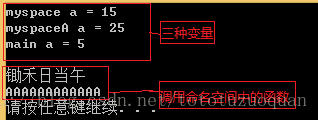
B:无名命名空间
#include"iostream"
//没有命名的命名空间中的参数可以直接调用
namespace
{
inta(3);
voidprint()
{
std::cout
<< "gogogo";
}
}
voidmain()
{
//说明没有命名的命名空间可以直接调用a
std::cout
<< a <<std::endl;
print();
getchar();
}
输出结果是:
3
gogogo
C:命名空间中所有的数据,函数,类,对象都是共有的,结构体内部的默认是共有的。
案例如下:
#include<iostream>
//namespace所有数据,函数,类,对象都是共有
namespacerunmove
{
inty(5);
int(*padd)(int,int);//函数指针接口
inty1(5);
classmyclass
{
//类中的变量等默认是私有的
public:
inta;
};
}
//对应上面的函数指针
intadd(inta,intb)
{
returna
+b;
}
intaddp(inta,intb)
{
std::cout
<< a <<"
" <<b
<<std::endl;
returna
+b;
}
//结构体默认是透明的(public的)
structMyStruct
{
intb;
private:
inta;//是私有
};
voidmain()
{
//namespace所有数据,函数,类,对象都是共有
MyStructstruct1;//结构体内部默认公有
struct1.b
= 20;
std::cout
<< struct1.b
<< std::endl;
std::cout
<< runmove::y
<< " " <<runmove::y1
<< std::endl;
runmove::padd
= addp;
std::cout
<< runmove::padd(5,
6) << std::endl;
getchar();
}
9.使用using关键字的时候,必须在命名空间的后面
关于作用域:
A:在函数体内使用using的时候,using的作用域是从using这行代码开始到函数体结束。
B:函数体外使用using的时候,作用域是从using这行代码开始到文件本文件结尾。
案例说明:
#include<iostream>
#include<stdlib.h>
namespacemydata
{
inta(6);
}
namespaceyourdata
{
inta(8);
}
//如果想使用mydata这个明明空间,并且使用using关键字,这时候要把using放
//在mydata命名空间后面
usingnamespacemydata;
usingnamespaceyourdata;
//using如果变量重名,会出现不明确错误,加上命名空间修饰符
voidgo()
{
//命名空间如果在块语句内部,作用域是定义开始到语句结束
std::cout
<< mydata::a
<< std::endl;
}
//using namespacemydata;//作用域为从代码开始到结束
voidmain()
{
std::cout
<< mydata::a
<< std::endl;
std::cout
<< yourdata::a
<< std::endl;
system("pause");
}
10.命名空间的嵌套,为命名空间起别名,命名空间的拓展
#include<iostream>
namespacerunrunrunrun
{
inta(10);
char
*str("gogogo");
namespacerun //命名空间的嵌套
{
inta(9);
}
}
//命名空间的拓展
namespacerunrunrunrun
{
int y(5);
//int a(15);重定义错误
}
//注意这里的r就是别名,而不是后者
namespacer
=runrunrunrun;//给命名空间起一个别名
voidmain()
{
//命名空间可以嵌套
std::cout
<< r::run::a
<< std::endl;
std::cout
<< r::y
<< std::endl;
std::cout
<< r::a
<< std::endl;
system("pause");
}

11.关于默认参数问题
A:默认参数必须放在右边
B:默认参数中间不允许出现不默认的
#include<stdio.h>
#include<stdlib.h>
#include<iostream>
//默认参数必须放在右边
//默认参数中间不允许出现不默认的
voidprint(intc,inta
= 1,intd
= 2,intb
= 3)
{
std::cout
<< a <<b
<<c <<std::endl;
}
voidprintf(doublec)
{
std::cout
<< "hello,world" <<std::endl;
}
voidmain()
{
//print(1,2,3);
//函数指针没有默认参数,必须全部输入数据
void(*pt1)(intc,inta,intd,intb)
=print;
pt1(100,1,2,3);//函数指针调用,没有用默认的
print(100);
system("pause");
}
1.auto关键字的用途
A:自动变量,可以自动获取类型,输出,类似泛型
B:自动变量,可以实现自动循环一维数组
C:自动循环的时候,对应的必须是常量
2.auto自动变量,自动匹配类型的案例如下:
注意:如果是在QT下运行上面的的程序需要加上C++11的相关配置(CONFIG += C++11)
3.通过auto关键字自动循环一维数组的案例
#include<iostream>
#include<stdlib.h>
#include<iomanip>
usingnamespacestd;
voidmain()
{
//定义一维数组,下面的数组名是一个指针常量
intnum[10]
= { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
//通过循环遍历出数组
for
(autodata
:num)
{
cout
<<setw(5) <<data;
}
cout
<<endl;
//当变成二维数组之后,不能直接通过auto来输出
//auto自动循环begin endl;必须是一个数组的常量
doublenum2[2][5]
= { 1.0, 2.0, 3.0, 4.5, 5, 6, 7, 8, 9, 10 };
for
(autodata
:num2)//泛型C++语法,循环一维数组,是个常量
{
cout
<<data <<std::endl;
for
(inti
= 0;i < 5;i++)
{
std::cout<<setw(5)
<< *(data +i);
}
cout
<<endl;
}
system("pause");
}
运行结果如下:
4.关于头文件
在C++中,为了区分C++,C++中不使用.h文件头
5.C++注重类型,它是一种强类型语言,严格检查类型。
6.C++中的输出函数,宽字符输出,赋初值的方式,输出符,::域控制符
#include<iostream> //在C++中,为了区分C++,C++中不使用.h文件头
#include<stdlib.h>
usingnamespacestd;
voidmain()
{
inta
= 5;
//C++中赋值:A变量名(数值) B:变量名=数值,通过这两种方式。
intb(5);
cout
<<a <<"
" <<b
<<endl;
//通过括号赋值
doublec(3.5);
cout
<<c <<endl;
//通过等号赋值
char
*pStr ="1234";
cout
<< *pStr <<"
" <<pStr
<<endl;
//通过括号赋值
char
*str("china");
cout
<< *str <<"
" <<str
<<endl;
//宽字符,汉子,棒子文
wchar_t
*str2(L"china");
wcout
<< *str2 <<"
" <<str2
<<endl;
system("pause");
}
#include<iostream>
voidmain()
{
//输出符
<<
//std命名空间
//::域控制符
std::cout
<< "hello world";
system("pause");
}
7.一个文件中使用另外一个文件中声明的变量的时候,C++要求显示的加上extern关键字调用这个全局变量。
8.调用命名空间中的变量已经命名空间中的函数
A:有名命名空间
#include"iostream"
#include"stdlib.h"
namespacemyspace
{
inta(15);
voidprint()
{
std::cout
<< "锄禾日当午"
<< std::endl;
}
}
namespacemyspaceA
{
inta(25);
voidprint()
{
std::cout
<< "AAAAAAAAAAAA" <<std::endl;
}
}
voidmain()
{
inta(5);
//如果想不被上面的a变量影响,而直接访问命名空间
//中的变量,这里要加上命名空间名
std::cout
<< "myspace a = " <<myspace::a
<< std::endl;
//调用myspaceA中定义的变量a
std::cout
<< "myspaceA a = " <<myspaceA::a
<< std::endl;
std::cout
<< "main a = " <<a
<<std::endl;
std::cout
<< std::endl;
//调用命名空间中的函数
myspace::print();
myspaceA::print();
system("pause");
}
运行结果如下:
B:无名命名空间
#include"iostream"
//没有命名的命名空间中的参数可以直接调用
namespace
{
inta(3);
voidprint()
{
std::cout
<< "gogogo";
}
}
voidmain()
{
//说明没有命名的命名空间可以直接调用a
std::cout
<< a <<std::endl;
print();
getchar();
}
输出结果是:
3
gogogo
C:命名空间中所有的数据,函数,类,对象都是共有的,结构体内部的默认是共有的。
案例如下:
#include<iostream>
//namespace所有数据,函数,类,对象都是共有
namespacerunmove
{
inty(5);
int(*padd)(int,int);//函数指针接口
inty1(5);
classmyclass
{
//类中的变量等默认是私有的
public:
inta;
};
}
//对应上面的函数指针
intadd(inta,intb)
{
returna
+b;
}
intaddp(inta,intb)
{
std::cout
<< a <<"
" <<b
<<std::endl;
returna
+b;
}
//结构体默认是透明的(public的)
structMyStruct
{
intb;
private:
inta;//是私有
};
voidmain()
{
//namespace所有数据,函数,类,对象都是共有
MyStructstruct1;//结构体内部默认公有
struct1.b
= 20;
std::cout
<< struct1.b
<< std::endl;
std::cout
<< runmove::y
<< " " <<runmove::y1
<< std::endl;
runmove::padd
= addp;
std::cout
<< runmove::padd(5,
6) << std::endl;
getchar();
}
9.使用using关键字的时候,必须在命名空间的后面
关于作用域:
A:在函数体内使用using的时候,using的作用域是从using这行代码开始到函数体结束。
B:函数体外使用using的时候,作用域是从using这行代码开始到文件本文件结尾。
案例说明:
#include<iostream>
#include<stdlib.h>
namespacemydata
{
inta(6);
}
namespaceyourdata
{
inta(8);
}
//如果想使用mydata这个明明空间,并且使用using关键字,这时候要把using放
//在mydata命名空间后面
usingnamespacemydata;
usingnamespaceyourdata;
//using如果变量重名,会出现不明确错误,加上命名空间修饰符
voidgo()
{
//命名空间如果在块语句内部,作用域是定义开始到语句结束
std::cout
<< mydata::a
<< std::endl;
}
//using namespacemydata;//作用域为从代码开始到结束
voidmain()
{
std::cout
<< mydata::a
<< std::endl;
std::cout
<< yourdata::a
<< std::endl;
system("pause");
}
10.命名空间的嵌套,为命名空间起别名,命名空间的拓展
#include<iostream>
namespacerunrunrunrun
{
inta(10);
char
*str("gogogo");
namespacerun //命名空间的嵌套
{
inta(9);
}
}
//命名空间的拓展
namespacerunrunrunrun
{
int y(5);
//int a(15);重定义错误
}
//注意这里的r就是别名,而不是后者
namespacer
=runrunrunrun;//给命名空间起一个别名
voidmain()
{
//命名空间可以嵌套
std::cout
<< r::run::a
<< std::endl;
std::cout
<< r::y
<< std::endl;
std::cout
<< r::a
<< std::endl;
system("pause");
}
11.关于默认参数问题
A:默认参数必须放在右边
B:默认参数中间不允许出现不默认的
#include<stdio.h>
#include<stdlib.h>
#include<iostream>
//默认参数必须放在右边
//默认参数中间不允许出现不默认的
voidprint(intc,inta
= 1,intd
= 2,intb
= 3)
{
std::cout
<< a <<b
<<c <<std::endl;
}
voidprintf(doublec)
{
std::cout
<< "hello,world" <<std::endl;
}
voidmain()
{
//print(1,2,3);
//函数指针没有默认参数,必须全部输入数据
void(*pt1)(intc,inta,intd,intb)
=print;
pt1(100,1,2,3);//函数指针调用,没有用默认的
print(100);
system("pause");
}
相关文章推荐
- C++中 auto自动变量,命名空间,using作用以及作用域
- C++中 auto自己主动变量,命名空间,using作用以及作用域
- 黑马程序员:命名空间以及using的作用
- c++ 命名空间 以及 作用域 函数参数 面向对象实验报告
- C++中的命名空间、using用法、区域运算符(::)详解
- c++使用命名空间的作用
- C++命名空间 namespace的作用和使用解析
- C++ using namespace std 详解 与 命名空间的使用
- C++基础积累(1)using namespace potter 自定义命名空间和使用
- C++命名空间 namespace的作用和使用解析
- XML DTD和XML SCHEMA以及命名空间的作用
- C++ using namespace std 详解 与 命名空间的使用
- c++的命名空间namespace的作用
- 【菜鸟C++学习笔记】2.命名空间的作用
- C++内存模型与名称空间(存储持续性、作用域、链接性、动态内存分配、命名空间)
- C++ 命名空间(十六)--namespace、using..
- c++高级---C++声明、定义、类的定义、头文件作用、头文件重复引用,不具名空间以及编译器编译链接过程
- 二 : using声明、using指示用于嵌套命名空间时的作用域
- C++学习笔记(九):作用域和命名空间
- Python中的命名空间、作用域以及locals() 和 globals()