C++课程设计作业,第八题
2014-06-21 20:43
447 查看
8、实现一个工资管理系统
系统的主要功能是计算职工当月工资并要求
存档案。
公司是一个不大不小公司,职工有种5类型,他们是技术人员,销售人员,文秘,技术经理,销售经理。世界从来就不是公平的,在公司内部也一样,不同职位工资不同。
技术人员工资是按小时算,薪酬为40元。销售人员工资是按销售提成,月工资为销售总额的5%。文秘为有4000元底薪,奖金要看当月的工作情况而定。技术经理和销售经理,均有每月5000元固定工资。技术经理工作业绩分为三个等级,每级有1000元奖金,销售经理资金由他管理团队销售业绩而定,为总经额的0.3%。
1、显示界面
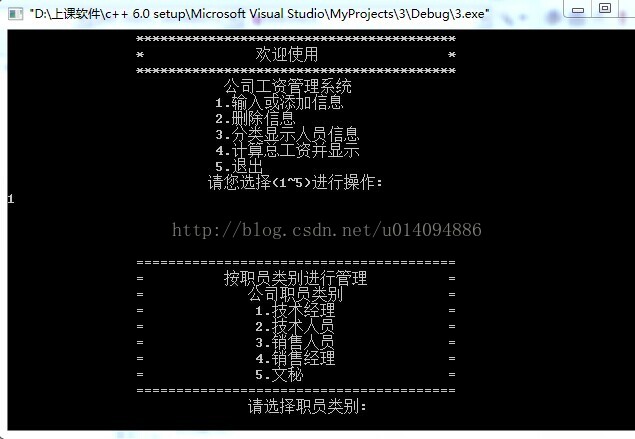
2、输入添加信息
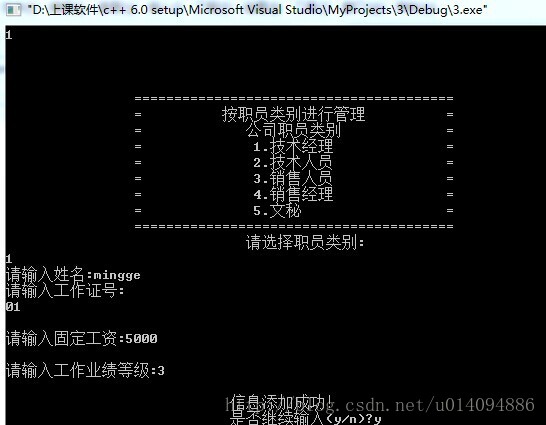
3、显示技术经理人员信息
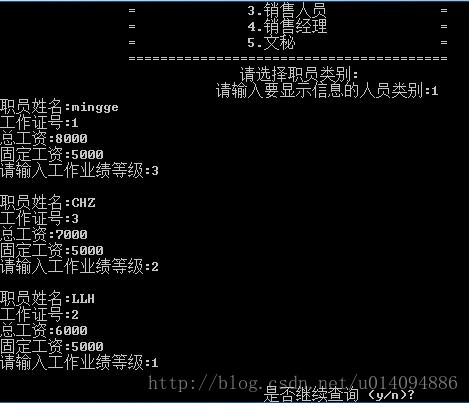
4、删除技术经理CHZ的信息
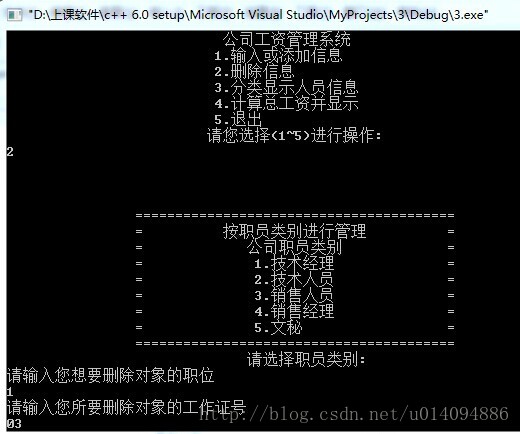
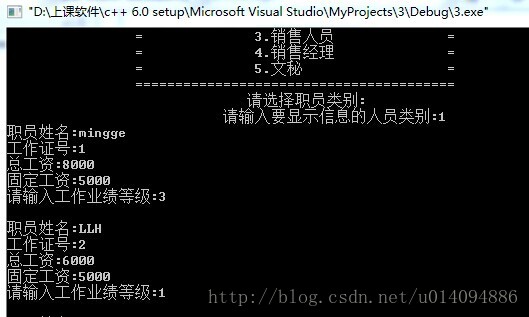
5、查询员工工资

6、添加技术人员
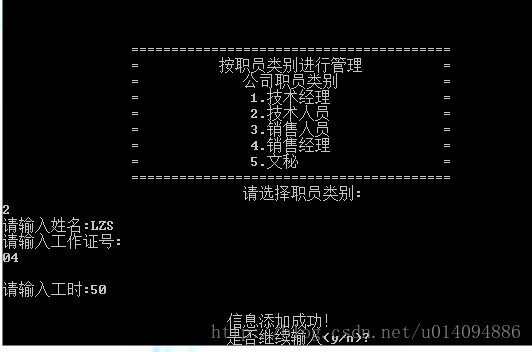
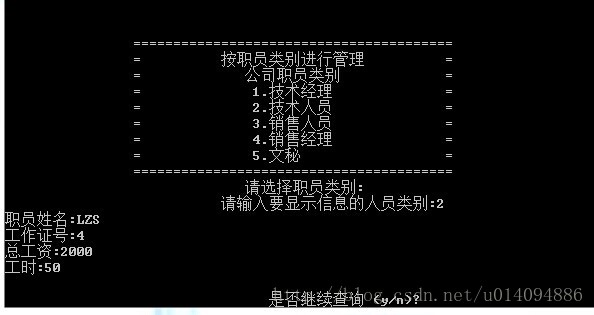
7、销售人员信息
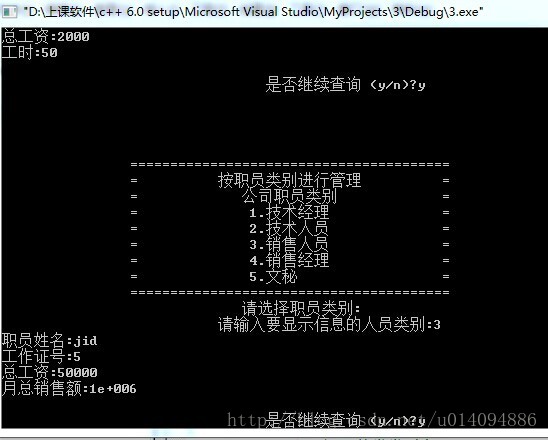
8、销售经理信息
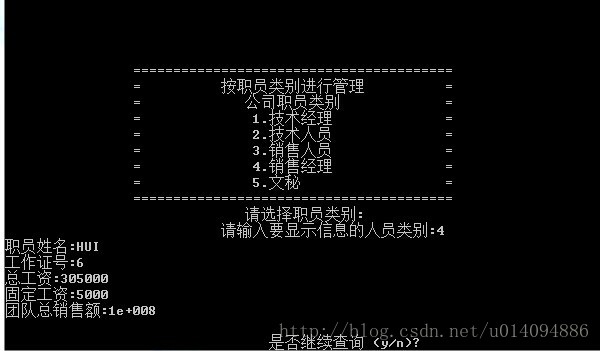
9、文秘信息
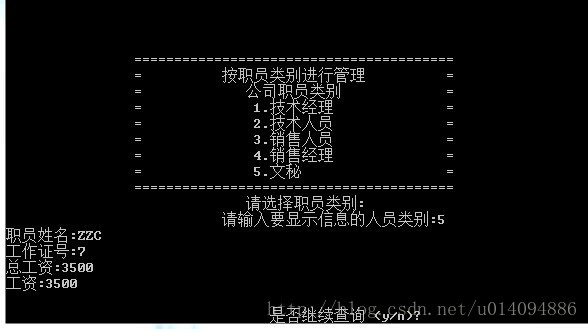
10、每次添加或删除人员之后,程序的保存功能就会自动实现,下图是我关闭程序之后再打开直接运行选择查看功能3的结果,证明系统的确有存档功能。
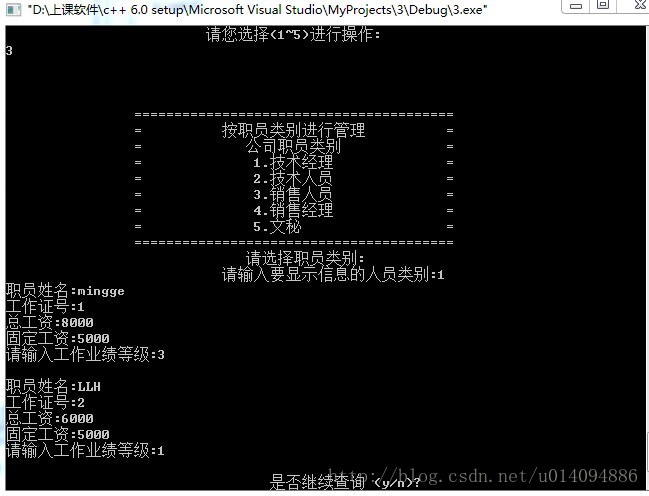
ps:谢谢明哥这学期对我们的指导。
系统的主要功能是计算职工当月工资并要求
存档案。
公司是一个不大不小公司,职工有种5类型,他们是技术人员,销售人员,文秘,技术经理,销售经理。世界从来就不是公平的,在公司内部也一样,不同职位工资不同。
技术人员工资是按小时算,薪酬为40元。销售人员工资是按销售提成,月工资为销售总额的5%。文秘为有4000元底薪,奖金要看当月的工作情况而定。技术经理和销售经理,均有每月5000元固定工资。技术经理工作业绩分为三个等级,每级有1000元奖金,销售经理资金由他管理团队销售业绩而定,为总经额的0.3%。
#include<iostream.h> class Employee//职员类 { protected: char Name[30];//姓名 int num;//工作证号 int kind;//类别 float Total_Salary;//总工资 public: char *Getname(); int Get_num(); void Input();//输入 void Output();//输出 void Compute_Total_Salary();//计算总工资 int Get_kind()//获取类别 { return kind; } float Get_Total_Salary()//获取总工资 { return Total_Salary; } void Set_kind()//设置基类类别为0 { kind=0; } void Set_kind0()//设置类别为0,(删除) { kind=0; } }; class jsmanager: public Employee//技术经理类 { private: float Salary;//固定工资 int Work_Age;//请输入工作业绩等级 public: jsmanager(){} void Set_kind()//设置技术经理类类别为1 { kind=1; } void Input(); void Compute_Total_Salary(); void Output(); ~jsmanager(){} }; class jishu:public Employee//技术人员类 { private: int Work_Hour;//工时 public: jishu(){} void Set_kind()//设置技术人员类类别为2 { kind=2; } void Input(); void Compute_Total_Salary(); void Output(); ~jishu(){} }; class xiaoshou:public Employee//销售人员类 { private: float Sale;//月总销售额 public: xiaoshou(){} void Set_kind()//设置销售人员类类别为3 { kind=3; } void Input(); void Compute_Total_Salary(); void Output(); ~xiaoshou(){} }; class xsmanager:public Employee//销售经理类 { private: float Salary;//固定工资 float Totalsale;//团队总销售业绩 public: xsmanager(){} void Set_kind()//设置销售经理类类别为4 { kind=4; } void Input(); void Compute_Total_Salary(); void Output(); ~xsmanager(){} }; class wenmi:public Employee//文秘类 { private: float Salary;//固定工资 public: wenmi(){} void Set_kind()//设置文秘类类别为5 { kind=5; } void Input(); void Compute_Total_Salary(); void Output(); ~wenmi(){} }; class System//系统类 { private: Employee A; jsmanager B[10]; jishu C[10]; xiaoshou D[10]; xsmanager E[10]; wenmi F[10]; static int j1,j2,j3,j4,j5; void infor1();//输入技术经理类对象数据 void infor2();//输入技术人员类对象数据 void infor3();//输入销售人员类对象数据 void infor4();//输入销售经理类对象数据 void infor5();//输入文秘类对象数据 void save();//将文件信息输出到内存 void Display2(int h); void Get_Total_Salary1(int h,char *name); void Get_Total_Salary2(int h,int n); void Delete_information1(int l,int g); void read(); void Interface1();//界面输出 public: System(); void In_information();//添加信息 void Delete_information();//删除信息 void Display();//查询信息 void Get_Total_Salary();//计算并显示工资 void Interface();//界面输出 }; void Employee::Input() { cout<<"请输入姓名:"; cin>>Name; cout<<"请输入工作证号:"<<endl; cin>>num; } void Employee::Output() { cout<<"职员姓名:"<<Name<<endl; cout<<"工作证号:"<<num<<endl; cout<<"总工资:"<<Total_Salary<<endl; } void jsmanager::Input() { Employee::Input(); cout<<endl<<"请输入固定工资:"; cin>>Salary; cout<<endl<<"请输入工作业绩等级:"; cin>>Work_Age; cout<<endl; } void jsmanager::Compute_Total_Salary() { Total_Salary=Salary+Work_Age*1000; } void jsmanager::Output() { Employee::Output(); cout<<"固定工资:"<<Salary<<endl; cout<<"请输入工作业绩等级:"<<Work_Age<<endl; cout<<endl; } void jishu::Input() { Employee::Input(); cout<<endl<<"请输入工时:"; cin>>Work_Hour; cout<<endl; } void jishu::Compute_Total_Salary() { Total_Salary=40*Work_Hour; } void jishu::Output() { Employee::Output(); cout<<"工时:"<<Work_Hour<<endl; cout<<endl; } void xiaoshou::Input() { Employee::Input(); cout<<endl<<"请输入月总销售额:"; cin>>Sale; cout<<endl; } void xiaoshou::Compute_Total_Salary() { Total_Salary=Sale*0.05; } void xiaoshou::Output() { Employee::Output(); cout<<"月总销售额:"<<Sale<<endl; cout<<endl; } void xsmanager::Input() { Employee::Input(); cout<<endl<<"请输入固定工资:"; cin>>Salary; cout<<endl<<"请输入团队总销售额:"; cin>>Totalsale; cout<<endl; } void xsmanager::Compute_Total_Salary() { Total_Salary=Salary+Totalsale*0.003; } void xsmanager::Output() { Employee::Output(); cout<<"固定工资:"<<Salary<<endl; cout<<"团队总销售额:"<<Totalsale<<endl; cout<<endl; } void wenmi::Input() { Employee::Input(); cout<<endl<<"固定工资:"; cin>>Salary; cout<<endl; } void wenmi::Compute_Total_Salary() { Total_Salary=Salary; } void wenmi::Output() { Employee::Output(); cout<<"工资:"<<Salary<<endl; cout<<endl; } //.cpp #include<iostream.h> #include<string.h> #include<fstream.h> #include<stdlib.h> #define N 60//职员的总数 char fileName[]="mm.dat";//存放职员信息的数据文件 char *Employee::Getname() { return Name; } int Employee::Get_num() { return num; } int System::j1=0; int System::j2=0; int System::j3=0; int System::j4=0; int System::j5=0; System::System() { save(); } void System::Interface1() { cout<<"\n\n\n"; cout<<"\t\t========================================"<<endl; cout<<"\t\t= 按职员类别进行管理 ="<<endl; cout<<"\t\t= 公司职员类别 ="<<endl; cout<<"\t\t= 1.技术经理 ="<<endl; cout<<"\t\t= 2.技术人员 ="<<endl; cout<<"\t\t= 3.销售人员 ="<<endl; cout<<"\t\t= 4.销售经理 ="<<endl; cout<<"\t\t= 5.文秘 ="<<endl; cout<<"\t\t========================================"<<endl; cout<<"\t\t 请选择职员类别: "<<endl; } void System::In_information()//输入信息 { int a; int again=1; char t; while(again) { Interface1(); cin>>a; switch(a) { case 1: infor1(); break; case 2: infor2(); break; case 3: infor3(); break; case 4: infor4(); break; case 5: infor5(); break; case 6: Interface(); break; default: cout<<"\t\t\t 没有此类职员!"<<endl; continue; } cout<<"\t\t\t 信息添加成功!"<<endl; cout<<"\t\t\t 是否继续输入(y/n)?"; cin>>t; cout<<endl; if(!(t=='Y'||t=='y')) again=0; } Interface(); } void System::infor1() { jsmanager A; // fstream datafile(fileName,ios::in|ios::out|ios::binary 4000 );//以二进制读和写方式打开文件 // datafile.seekp(0,ios::end);//写指针指到文件尾部 A.Input();//输入数据 A.Set_kind(); A.Compute_Total_Salary();//计算工资 // datafile.write((char *)&A,sizeof(class jsmanager));//将添加数据后的信息写入文件 B[j1++]=A;//将添加数据后的信息存入文件 // datafile.close();//关闭文件 } void System::infor2() { jishu A; // fstream datafile(fileName,ios::in|ios::out|ios::binary);//以二进制读和写方式打开文件 // datafile.seekp(0,ios::end);//写指针指到文件尾部 A.Input();//输入数据 A.Set_kind(); A.Compute_Total_Salary();//计算工资 //datafile.write((char *)&A,sizeof(class jishu));//将添加数据后的信息写入文件 C[j2++]=A;//将添加数据后的信息存入文件 //datafile.close();//关闭文件 } void System::infor3() { xiaoshou A; // fstream datafile(fileName,ios::in|ios::out|ios::binary);//以二进制读和写方式打开文件 // datafile.seekp(0,ios::end);//写指针指到文件尾部 A.Input();//输入数据 A.Set_kind(); A.Compute_Total_Salary();//计算工资 // datafile.write((char *)&A,sizeof(class xiaoshou));//将添加数据后的信息写入文件 D[j3++]=A;//将添加数据后的信息存入文件 // datafile.close();//关闭文件 } void System::infor4() { xsmanager A; // fstream datafile(fileName,ios::in|ios::out|ios::binary);//以二进制读和写方式打开文件 // datafile.seekp(0,ios::end);//写指针指到文件尾部 A.Input();//输入数据 A.Set_kind(); A.Compute_Total_Salary();//计算工资 // datafile.write((char *)&A,sizeof(class xsmanager));//将添加数据后的信息写入文件 E[j4++]=A;//将添加数据后的信息存入文件 // datafile.close();//关闭文件 } void System::infor5() { wenmi A; // fstream datafile(fileName,ios::in|ios::out|ios::binary);//以二进制读和写方式打开文件 // datafile.seekp(0,ios::end);//写指针指到文件尾部 A.Input();//输入数据 A.Set_kind(); A.Compute_Total_Salary();//计算工资 // datafile.write((char *)&A,sizeof(class wenmi));//将添加数据后的信息写入文件 F[j5++]=A;//将添加数据后的信息存入文件 // datafile.close();//关闭文件 } void System::save() { int a; fstream datafile(fileName,ios::in|ios::out|ios::binary);//以二进制读和写方式打开文件 datafile.read((char *)&A,sizeof(Employee));//读取基类职员类信息的长度 while(!datafile.eof())//测试文件是否结束,是返回1,否则返回0,为了遍历整个文件,以便对它进行读取 { a=A.Get_kind();//获得信息的种类编号 switch(a)//判定人员的类别 { case 1: { datafile.seekp(-1*sizeof(class Employee),ios::cur);//将写指针移到当前位置之前1个字节处 datafile.read((char *)&B[j1],sizeof(jsmanager));//从文件中重新读取完整的职员信息,存入B[j1]中,长度为行政类的长度 B[j1].Set_kind(); j1++; break; } case 2: { datafile.seekp(-1*sizeof(class Employee),ios::cur);//将写指针移到当前位置之前1个字节处 datafile.read((char *)&C[j2],sizeof(jishu));//从文件中重新读取完整的职员信息,存入C[j2]中,长度为技术类的长度 C[j2].Set_kind(); j2++; break; } case 3: { datafile.seekp(-1*sizeof(class Employee),ios::cur);//将写指针移到当前位置之前1个字节处 datafile.read((char *)&D[j3],sizeof(xiaoshou));//从文件中重新读取完整的职员信息,存入D[j3]中,长度为销售类的长度 D[j3].Set_kind(); j3++; break; } case 4: { datafile.seekp(-1*sizeof(class Employee),ios::cur);//将写指针移到当前位置之前1个字节处 datafile.read((char *)&E[j4],sizeof(xsmanager));//从文件中重新读取完整的职员信息,存入E[j4]中,长度后勤类的长度 E[j4].Set_kind(); j4++; break; } case 5: { datafile.seekp(-1*sizeof(class Employee),ios::cur);//将写指针移到当前位置之前1个字节处 datafile.read((char *)&F[j5],sizeof(wenmi));//从文件中重新读取完整的职员信息,存入F[j5]中,长度为临聘类的长度 F[j5].Set_kind(); j5++; break; } default: break; } datafile.read((char *)&A,sizeof(Employee));//循环 } datafile.close();//关闭文件 } void System::Display2(int h) { int s=0,found=0; switch(h)//判定人员的类别 { case 1: while(s<j1) { B[s].Compute_Total_Salary(); B[s].Output(); found=1; s++; } break; case 2: while(s<j2) { C[s].Compute_Total_Salary(); C[s].Output(); found=1; s++; } break; case 3: while(s<j3) { D[s].Compute_Total_Salary(); D[s].Output(); found=1; s++; } break; case 4: while(s<j4) { E[s].Compute_Total_Salary(); E[s].Output(); found=1; s++; } break; case 5: while(s<j5) { F[s].Compute_Total_Salary(); F[s].Output(); found=1; s++; } break; } if(found==0) cout<<"\n\n\t\t 对不起,该类别中没有您所要查看信息的职员!"<<endl; } void System::Display()//显示信息 { int r; int again=1; char t; while(again) { Interface1(); cout<<"\t\t 请输入要显示信息的人员类别:"; cin>>r; Display2(r); cout<<"\t\t\t 是否继续查询 (y/n)?"; cin>>t; cout<<endl; if(!(t=='Y'||t=='y')) again=0; } Interface(); } void System::Get_Total_Salary2(int h,int no) { int s=0,found=0; float Total_Salary; switch(h) { case 1: while(s<j1) { if(no==B[s].Get_num()) { B[s].Compute_Total_Salary(); cout<<"\t\t 职员工作证号:"<<no<<endl; cout<<"\t\t 该职员的总工资为:"<<B[s].Get_Total_Salary()<<endl; found=1; } s++; } break; case 2: while(s<N) { if(no==C[s].Get_num()) { C[s].Compute_Total_Salary(); cout<<"\t\t 职员工作证号:"<<no<<endl; cout<<"\t\t 该职员的总工资为:"<<C[s].Get_Total_Salary()<<endl; found=1; } s++; } break; case 3: while(s<N) { if(no==D[s].Get_num()) { D[s].Compute_Total_Salary(); cout<<"\t\t 职员工作证号:"<<no<<endl; cout<<"\t\t 该职员的总工资为:"<<D[s].Get_Total_Salary()<<endl; found=1; } s++; } break; case 4: while(s<N) { if(no==E[s].Get_num()) { E[s].Compute_Total_Salary(); cout<<"\t\t 职员工作证号:"<<no<<endl; cout<<"\t\t 该职员的总工资为:"<<E[s].Get_Total_Salary()<<endl; found=1; } s++; } break; } if(found==0) cout<<"\n\n\t\t 对不起,该类别中没有您所要查看工资的职员!"<<endl; } void System::Get_Total_Salary1(int h,char *name) { int s=0,found=0; float Total_Salary; switch(h) { case 5: while(s<N) { if(strcmp(name,F[s].Getname())==0) { F[s].Compute_Total_Salary(); cout<<"\t\t 职员姓名:" <<name<<endl; cout<<"\t\t 该职员的总工资为:"<<F[s].Get_Total_Salary()<<endl; found=1; } s++; } break; } if(found==0) cout<<"\n\n\t\t 对不起,该类别中没有您所要查看工资的职员!"<<endl; } void System::Get_Total_Salary()//查询工资 { int r,n; char name[20]; int again=1,count; char t; cout<<"请输入要查询的职员类别: "<<endl; Interface1(); while(again) { cin>>r; switch(r) { case 1: { cout<<"\n\t\t 请输入所要查看工资的人员的工作证号"; cin>>n; Get_Total_Salary2(r,n); break; } case 2: { cout<<"\n\t\t 请输入所要查看工资的人员的工作证号"; cin>>n; Get_Total_Salary2(r,n); break; } case 3: { cout<<"\n\t\t 请输入所要查看工资的人员的工作证号"; cin>>n; Get_Total_Salary2(r,n); break; } case 4: { cout<<"\n\t\t 请输入所要查看工资的人员的工作证号"; cin>>n; Get_Total_Salary2(r,n); break; } case 5: { cout<<"\n\t\t 请输入所要查看工资的人员的姓名"; cin>>name; Get_Total_Salary1(r,name); break; } default: break; } cout<<"\n\t\t 是否继续查看职员工资(y/n)?"; cin>>t; cout<<endl; if(!(t=='Y'||t=='y')) again=0; else cout<<"请输入要查询的职员类别: "<<endl; } Interface(); } void System::read() { fstream datafile(fileName,ios::out|ios::binary);//以二进制读和写方式打开文件 for(int i1=0;i1<j1;i1++) if(B[i1].Get_kind()==1) datafile.write((char *)&B[i1],sizeof(class jsmanager));//将添加数据后的信息写入B[j1]中,长度为技术经理类的长度 for(int i2=0;i2<j2;i2++) if(C[i2].Get_kind()==2) datafile.write((char *)&C[i2],sizeof(class jishu));//将添加数据后的信息写入C[j2]中,长度为技术类的长度 for(int i3=0;i3<j3;i3++) if(D[i3].Get_kind()==3) datafile.write((char *)&D[i3],sizeof(class xiaoshou));//将添加数据后的信息写入D[j3]中,长度为销售类的长度 for(int i4=0;i4<j4;i4++) if(E[i4].Get_kind()==4) datafile.write((char *)&E[i4],sizeof(class xsmanager));//将添加数据后的信息写入E[j4]中,长度为销售经理类的长度 for(int i5=0;i5<j5;i5++) if(F[i5].Get_kind()==5) datafile.write((char *)&E[i5],sizeof(class wenmi));//将添加数据后的信息写入F[j5]中,长度为文秘类的长度 datafile.close(); } void System::Delete_information1(int l,int g) { int s=0,found=0; switch(l) { case 1: while(s<j1) { if(g==B[s].Get_num()) { int i; for(i=s;i<j1-1;i++) { B[i]=B[i+1];//把后一个工作证号的人员的信息赋给前一个 } B[i].Set_kind0();//标记类别为0 j1--;//j1=j1-1 found=1; } s++; } break; case 2: while(s<j2) { if(g==C[s].Get_num()) { int i; for(i=s;i<j2-1;i++) { C[i]=C[i+1];//把后一个工作证号的人员的信息赋给前一个 } C[i].Set_kind0();//标记类别为0 j2--; found=1; } s++; } break; case 3: while(s<j3) { if(g==D[s].Get_num()) { int i; for(i=s;i<j3-1;i++) { D[i]=D[i+1];//把后一个工作证号的人员的信息赋给前一个 } D[i].Set_kind0();//标记类别为0 j3--; found=1; } s++; } break; case 4: while(s<j4) { if(g==E[s].Get_num()) { int i; for(i=s;i<j4-1;i++) { E[i]=E[i+1];//把后一个工作证号的人员的信息赋给前一个 } E[i].Set_kind0();//标记类别为0 j4--; found=1; } s++; } break; case 5: while(s<j5) { if(g==F[s].Get_num()) { int i; for(i=s;i<j5-1;i++) { F[i]=F[i+1];//把后一个工作证号的人员的信息赋给前一个 } F[i].Set_kind0();//标记类别为0 j5--; found=1; } s++; } break; } if(found==0) cout<<"\n\n\t\t 对不起,该类别中没有您所要查询的职员!"<<endl; } void System::Delete_information() { int a,h; char t; int again=1; while (again) { Interface1(); cout<<"请输入您想要删除对象的职位"<<endl; cin>>a; cout<<"请输入您所要删除对象的工作证号"<<endl; cin>>h; Delete_information1(a,h); cout<<"\t\t\t是否继续删除(y/n)?"; cin>>t; cout<<endl; if(!(t=='Y'||t=='y')) again=0; } Interface(); } void System::Interface()//界面 { int r; cout<<"\n\n\n\n\n\n\n"; cout<<"\t\t****************************************"<<endl; cout<<"\t\t* 欢迎使用 *"<<endl; cout<<"\t\t****************************************"<<endl; cout<<"\t\t 公司工资管理系统 "<<endl; cout<<"\t\t 1.输入或添加信息 "<<endl; cout<<"\t\t 2.删除信息 "<<endl; cout<<"\t\t 3.分类显示人员信息 "<<endl; cout<<"\t\t 4.计算总工资并显示 "<<endl; cout<<"\t\t 5.退出 "<<endl; cout<<"\t\t 请您选择(1~5)进行操作: "<<endl; cin>>r; switch(r) { case 1: In_information(); break; case 2: Delete_information(); break; case 3: Display(); break; case 4: Get_Total_Salary(); break; case 5: read(); exit(0); } } void main(void) { System s; s.Interface(); }
1、显示界面
2、输入添加信息
3、显示技术经理人员信息
4、删除技术经理CHZ的信息
5、查询员工工资
6、添加技术人员
7、销售人员信息
8、销售经理信息
9、文秘信息
10、每次添加或删除人员之后,程序的保存功能就会自动实现,下图是我关闭程序之后再打开直接运行选择查看功能3的结果,证明系统的确有存档功能。
ps:谢谢明哥这学期对我们的指导。
相关文章推荐
- 代写编程的作业、笔试题、课程设计,包括但不限于C/C++/Python
- C++课程设计类作业3
- C++课程设计(作业)
- C++课程设计类作业2
- C++课程设计类作业4
- 第九周C++作业报告六 (第八周四)
- c++课程设计
- C++面向对象课程设计 通信录
- C++课程设计 面积运算
- 课程设计C++
- [设计] C++课程设计-ATM (纪念大一)
- 课程设计大作业参考题目
- C++课程设计指导书
- [C++]数据结构课程设计:迷宫老鼠1.0
- C++课程设计 之 俄罗斯方块
- C++课程设计( 南阳康佳公司的设备管理系统 )
- c++课程设计 对日期的基本运算 使用重载
- Linux下写的一个C++课程设计
- c++ 数据结构课程设计 魔王语言解释
- C/C++语言课程设计任务书