web开发中常用的上传下载代码 推荐
2014-06-06 15:34
507 查看
【web开发】之常用上传下载代码
1、常用上传代码
2、上传缩略图代码(按一定比例缩放)
4、常用下载代码(根据路劲下载)
此方法基本可以下载所有附件
5、直接下载(无路径,后台生成之后直接下载适合Excel,word,pdf等)
Excel:
上传下载说白了,其实就是输入,输出流的问题!了解输入输出流的本质这些基本上就不是问题了!
以上代码都是经本人测试之后拷贝出来直接可以使用!
有问题,请举手!
微信公众号:IT_Online
职场动态,技术咨询,招聘信息,请拿出手机扫这里!
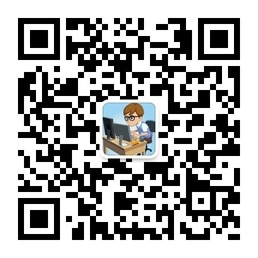
1、常用上传代码
/** * * @param upload 上传文件 * @param reaName 名称 * @param uploadType 类型 * @return * @throws IOException */ public String uploadFile(File upload, String reaName, String uploadType) throws IOException { String uploadpath="D:/csg"; String uploadpath2="/upload/image/"; String fileDir = uploadpath +uploadpath2; OutputStream os = null; InputStream is = null; File file=new File(fileDir); if(!file.exists()){ file.mkdirs(); } try { is = new FileInputStream(upload); os = new FileOutputStream(fileDir + reaName); int bytesRead = 0; byte[] buffer = new byte[8192]; while ((bytesRead = is.read(buffer, 0, 8192)) != -1) os.write(buffer, 0, bytesRead); } catch (IOException e) { e.printStackTrace(); } finally { if (os != null) { os.close(); } if (is != null) { is.close(); } } return uploadpath2+reaName; }此方法基本上可以上传所有附件
2、上传缩略图代码(按一定比例缩放)
public String uploadFile(File upload, String reaName, String uploadType) throws IOException { String uploadpath="D:/csg"; String uploadpath2="/upload/image/small/"; String fileDir = uploadpath +uploadpath2; InputStream is = null; BufferedImage bis = null; is = new FileInputStream(upload); File file=new File(fileDir); if(!file.exists()){ file.mkdirs(); } try { File reviaryFile = new File(fileDir + "small20130606.jpg"); AffineTransform transform = new AffineTransform(); bis = ImageIO.read(is); int w = bis.getWidth(); int h = bis.getHeight(); int newWidth = (int) (w * 0.1D); int newHight = (int) (h * 0.1D); transform.setToScale(0.1, 0.1); AffineTransformOp ato = new AffineTransformOp(transform, null); BufferedImage bid = new BufferedImage(newWidth, newHight, 5); ato.filter(bis, bid); ImageIO.write(bid, "jpg", reviaryFile); } catch (Exception e) { System.out.println("上传缩略图失败"); }finally{ if (is != null) { is.close(); } } return fileDir; }3、上传缩略图代码(指定比例缩放)
public String uploadFile(File upload, String reaName, String uploadType) throws IOException { String uploadpath="D:/csg"; String uploadpath2="/upload/image/small/"; String fileDir = uploadpath +uploadpath2; InputStream is = null; BufferedImage bis = null; Image srcUpload = null; is = new FileInputStream(upload); File file=new File(fileDir); if(!file.exists()){ file.mkdirs(); } try { srcUpload = ImageIO.read(is); int newWidth = 0, newHight = 0; int w = srcUpload.getWidth(null); int h = srcUpload.getHeight(null); if (w >= h) { newWidth = 90; newHight = h * 90 / w; } else { newHight = 90; newWidth = w * 90 / h; } BufferedImage image = new BufferedImage(newWidth, newHight, BufferedImage.TYPE_3BYTE_BGR); image.getGraphics().drawImage(srcUpload.getScaledInstance(newWidth, newHight, Image.SCALE_SMOOTH), 0, 0, null); FileOutputStream out = new FileOutputStream(fileDir + "/" + "small123.jpg"); JPEGImageEncoder encoder = JPEGCodec.createJPEGEncoder(out); encoder.encode(image); image.flush(); out.flush(); out.close(); System.gc(); } catch (Exception e) { System.out.println("上传缩略图失败"); }finally{ if (is != null) { is.close(); } } return fileDir; }
4、常用下载代码(根据路劲下载)
Dao dao = new Dao(); UploadFiles files = dao.querykById(id); String uploadpath = "D:/csg"; String path = uploadpath + files.getPath(); InputStream bis = null; OutputStream bos = null; try { File fileInstance = new File(path); if (!fileInstance.exists()) { System.out.println("---------下载文件不存在,请联系管理员-------------"); } else { HttpServletResponse response = (HttpServletResponse) ActionContext .getContext().get(ServletActionContext.HTTP_RESPONSE); bis = new BufferedInputStream(new FileInputStream(fileInstance)); bos = new BufferedOutputStream(response.getOutputStream()); response.setContentType("application/octet-stream;charset=UTF-8"); response.setHeader("Content-Disposition","attachment;filename="+ URLEncoder.encode(files.getUploadRealName(),"UTF-8")); byte[] buff = new byte[1024]; int bytesRead; while (-1 != (bytesRead = bis.read(buff, 0, buff.length))) { // 将buff中的数据写到客户端的内存 bos.write(buff, 0, bytesRead); } bos.close(); bis.close(); } } catch (IOException e) { System.out.println("下载失败"); } finally { bos.close(); bis.close(); } return null; }
此方法基本可以下载所有附件
5、直接下载(无路径,后台生成之后直接下载适合Excel,word,pdf等)
Excel:
public static void export(HttpServletResponse response,Workbook wb,String fileName)throws Exception{ response.setHeader("Content-Disposition", "attachment;filename="+new String(fileName.getBytes("utf-8"),"iso8859-1")); response.setContentType("application/ynd.ms-excel;charset=UTF-8"); OutputStream out=response.getOutputStream(); wb.write(out); out.flush(); out.close(); }PDF:
ByteArrayOutputStream ba = new ByteArrayOutputStream(); PdfWriter writer = PdfWriter.getInstance(document, ba); // 打开文档,生成页头页脚等信息在open之前 document.open(); //省略n行生成table的代码 document.add(table); document.close(); // 关闭文本,释放资源 HttpServletResponse response=super.getResponse(); response.setContentLength(ba.size()); response.setContentType("application/octet-stream;charset=UTF-8"); String str="审核表.pdf"; response.setHeader("Content-Disposition", "attachment;filename="+new String(str.getBytes("utf-8"),"iso8859-1")); ServletOutputStream out = response.getOutputStream(); ba.writeTo(out); out.flush();ps:直接下载就是在生成之后直接将文本信息传进来,就行了。而Excel这个例子是需要将生成之后的Workbook返回去,传给export方法!PDF,Excel,word直接下载方法基本类似!
上传下载说白了,其实就是输入,输出流的问题!了解输入输出流的本质这些基本上就不是问题了!
以上代码都是经本人测试之后拷贝出来直接可以使用!
有问题,请举手!
微信公众号:IT_Online
职场动态,技术咨询,招聘信息,请拿出手机扫这里!
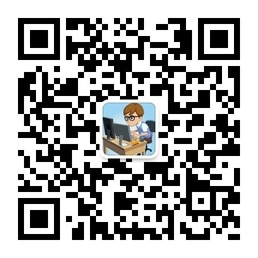
相关文章推荐
- 18常用web开发 浮动层、提示层代码下载
- WEB编程开发常用的代码
- [XNA研究强烈推荐]XNA开发雷电类游戏,源代码提供下载学习(提供代码和视频演示)
- [导入]一些web开发中常用的、做成cs文件的js代码 - 搜刮来的
- 一些web开发中常用的、做成cs文件的js代码
- 收集的WEB编程开发常用代码
- WEB编程开发常用的代码
- 一些web开发中常用的、做成cs文件的js代码 - 搜刮来的
- 论Web控件开发 - 完美上传下载控件“新”(四)
- 论Web控件开发 - 完美上传下载控件“新”(三)
- 一些web开发中常用的、做成cs文件的js代码 - 转帖来的
- WEB编程开发常用的代码
- WEB编程开发常用的代码[转载于CSDN文档中心]
- WEB编程开发常用的代码
- 论Web控件开发 - 完美上传下载控件“新”(五)
- 论Web控件开发 - 完美上传下载控件
- WEB编程开发常用的代码
- WEB编程开发常用的代码
- WEB编程开发常用的代码
- WEB编程开发常用的代码