C#学习之文件操作(1)
2014-05-15 23:26
281 查看
C#中对文件的操作和管理主要用到了下面几个类
System.MarshalByRefObject:这是.NET类中用于远程操作的基对象类,它允许在应用程序域之间编组数据。
FileSystemInfo:这是表示任何文件系统对象的基类
FileInfo和FIle:这些类表示文件系统上的文件
DirectoryInfo和Directory:这些类表示文件系统上的文件夹
Path:这个类包含的静态成员可以用于处理路径名
DriveInfo:它的属性和方法提供了指定驱动器的信息
表示文件和文件夹的.NET类
注意,上面的列表中有两个用于表示文件夹的类,和两个用于表示文件的类。使用哪个类主要依赖于访问该文件夹和文件的次数:
Directory类和File类只包含静态方法,不能被实例化。只要调用一个成员方法,提供合适文件系统对象的路径,就可以使用这些类。如果只对文件夹或文件执行一个操作,使用这些类比较有效,因为这样可以省去实例化.NET类的系统开销。
DirectoryInfo类和FileInfo类实现与Directory类和File类大致相同的公共方法,并拥有一些公共属性和构造函数,但他们都是有状态的,并且这些类的成员都不是静态的。需要实例化这些类,之后把每个实例与特定的文件夹或文件关联起来。如果使用同一个对象执行多个操作,使用这些类就比较有效。这是因为在构造时 它们将读取合适文件系统对象的身份验证和其他信息,无论对每个对象调用了多少方法,都不需要再次读取这些信息,相比较而言,在调用每个方法时,相应的无状态类需要再次检查文件和文件夹的详细内容。
Path类
我们不能实例化Path类,然而它提供了一种静态方法,可以更容易地对路径名执行操作。使用Path类要比手动处理各个符号容易得多。尤其因为Path类在处理不同的操作系统上的路径名时,能够识别不同的格式。经常用到的就是Path.Combine()方法。
FileProperties示例
在这里简单的写一个示例,一个简单的用户界面,可以浏览文件系统,查看文件的一些相关属性。
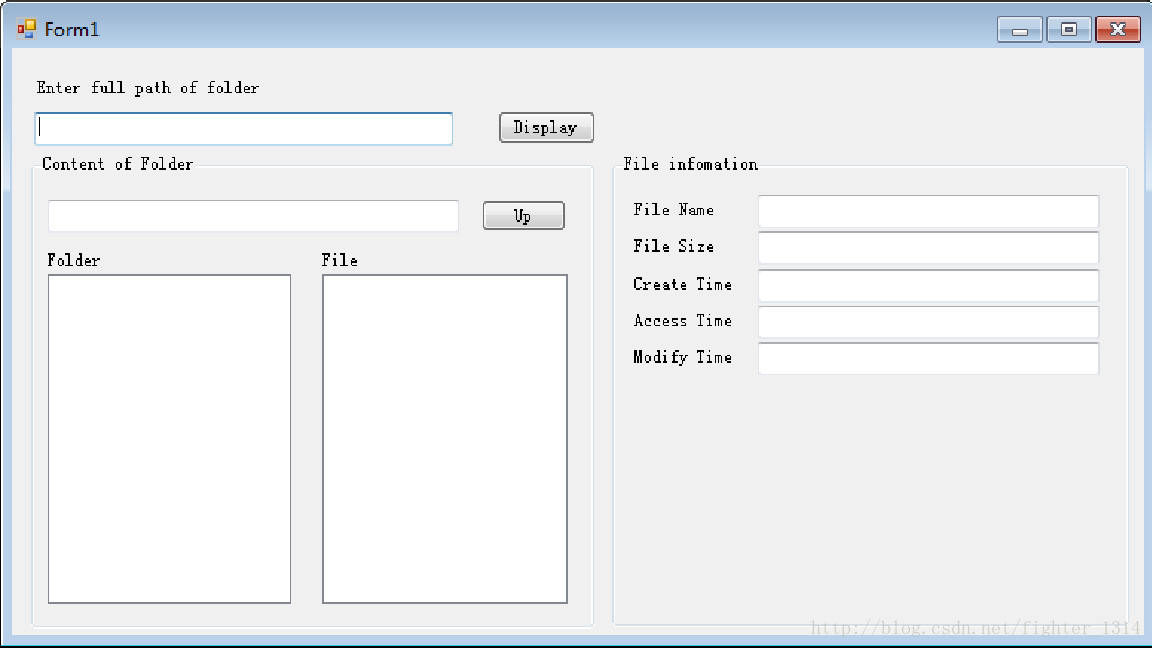
在这里,用一些直观的名字来命名这些空间。tbFolderPath,btDisplay,tbUpFolder,btUp,lbFolder,lbFile,tbFileName,tbFileSize,tbCreateTime,tbAccessTime,tbModifyTime
与此同时,需要在程序中添加一个成员变量:
private string currentFolderPath;
现在需要为用户生成的事件添加事件处理程序。
这样就实现了一个浏览文件的系统。能够大概的了解到FileInfo类DirectoryInfo类的一些用法。
System.MarshalByRefObject:这是.NET类中用于远程操作的基对象类,它允许在应用程序域之间编组数据。
FileSystemInfo:这是表示任何文件系统对象的基类
FileInfo和FIle:这些类表示文件系统上的文件
DirectoryInfo和Directory:这些类表示文件系统上的文件夹
Path:这个类包含的静态成员可以用于处理路径名
DriveInfo:它的属性和方法提供了指定驱动器的信息
表示文件和文件夹的.NET类
注意,上面的列表中有两个用于表示文件夹的类,和两个用于表示文件的类。使用哪个类主要依赖于访问该文件夹和文件的次数:
Directory类和File类只包含静态方法,不能被实例化。只要调用一个成员方法,提供合适文件系统对象的路径,就可以使用这些类。如果只对文件夹或文件执行一个操作,使用这些类比较有效,因为这样可以省去实例化.NET类的系统开销。
DirectoryInfo类和FileInfo类实现与Directory类和File类大致相同的公共方法,并拥有一些公共属性和构造函数,但他们都是有状态的,并且这些类的成员都不是静态的。需要实例化这些类,之后把每个实例与特定的文件夹或文件关联起来。如果使用同一个对象执行多个操作,使用这些类就比较有效。这是因为在构造时 它们将读取合适文件系统对象的身份验证和其他信息,无论对每个对象调用了多少方法,都不需要再次读取这些信息,相比较而言,在调用每个方法时,相应的无状态类需要再次检查文件和文件夹的详细内容。
Path类
我们不能实例化Path类,然而它提供了一种静态方法,可以更容易地对路径名执行操作。使用Path类要比手动处理各个符号容易得多。尤其因为Path类在处理不同的操作系统上的路径名时,能够识别不同的格式。经常用到的就是Path.Combine()方法。
FileProperties示例
在这里简单的写一个示例,一个简单的用户界面,可以浏览文件系统,查看文件的一些相关属性。
在这里,用一些直观的名字来命名这些空间。tbFolderPath,btDisplay,tbUpFolder,btUp,lbFolder,lbFile,tbFileName,tbFileSize,tbCreateTime,tbAccessTime,tbModifyTime
与此同时,需要在程序中添加一个成员变量:
private string currentFolderPath;
现在需要为用户生成的事件添加事件处理程序。
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.IO; namespace FileOperation { public partial class Form1 : Form { string currentFolderPath = string.Empty; public Form1() { InitializeComponent(); } private void ClearAllFields() { this.lbFile.Items.Clear(); this.lbFolder.Items.Clear(); this.tbAccessTime.Text = ""; this.tbCreateTime.Text = ""; this.tbFileName.Text = ""; this.tbFileSize.Text = ""; this.tbFolderPath.Text = ""; this.tbModifyTime.Text = ""; this.tbUpFolder.Text = ""; } private void DisplayFileInfo(string fileFulName) { FileInfo file = new FileInfo(fileFulName); if (!file.Exists) { throw new FileNotFoundException("File not found" + fileFulName); } tbFileName.Text = file.Name; tbFileSize.Text = file.Length.ToString()+"Bytes"; tbAccessTime.Text = file.LastAccessTime.ToLongDateString(); tbCreateTime.Text = file.CreationTime.ToLongTimeString(); tbModifyTime.Text = file.LastWriteTime.ToLongDateString(); } private void DisplayFolderList(string folderFullName) { DirectoryInfo directory = new DirectoryInfo(folderFullName); if (!directory.Exists) { throw new DirectoryNotFoundException("Folder not found:" + folderFullName); } ClearAllFields(); tbUpFolder.Text = directory.FullName; currentFolderPath = directory.FullName; foreach (DirectoryInfo item in directory.GetDirectories()) { lbFolder.Items.Add(item.Name); } foreach (FileInfo item in directory.GetFiles()) { lbFile.Items.Add(item.Name); } } private void btDisplay_Click(object sender, EventArgs e) { try { string folderPath = tbFolderPath.Text; DirectoryInfo folder = new DirectoryInfo(folderPath); if (folder.Exists) { DisplayFolderList(folder.FullName); return; } FileInfo file = new FileInfo(folderPath); if (file.Exists) { DisplayFolderList(file.Directory.FullName); int index = lbFile.Items.IndexOf(file.Name); lbFile.SetSelected(index, true); return; } throw new FileNotFoundException("There is no file or folder with this name" + tbFolderPath.Text); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void lbFile_SelectedIndexChanged(object sender, EventArgs e) { try { string selectedString = lbFile.SelectedItem.ToString(); string fullFileName = Path.Combine(currentFolderPath, selectedString); DisplayFileInfo(fullFileName); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void lbFolder_SelectedIndexChanged(object sender, EventArgs e) { try { string selectedString = lbFolder.SelectedItem.ToString(); string fullPathName = Path.Combine(currentFolderPath, selectedString); DisplayFolderList(fullPathName); } catch (Exception ex) { MessageBox.Show(ex.Message); } } private void btUp_Click(object sender, EventArgs e) { try { string folderPath = new FileInfo(currentFolderPath).DirectoryName; DisplayFolderList(folderPath); } catch (Exception ex) { MessageBox.Show(ex.Message); } } } }
这样就实现了一个浏览文件的系统。能够大概的了解到FileInfo类DirectoryInfo类的一些用法。
相关文章推荐
- 黑马程序员-C#学习-文件操作
- c#文件操作的学习
- Visual Studio 2017中使用正则修改部分内容 如何使用ILAsm与ILDasm修改.Net exe(dll)文件 C#学习-图解教程(1):格式化数字字符串 小程序开发之图片转Base64(C#、.Net) jquery遍历table为每一个单元格取值及赋值 。net加密解密相关方法 .net关于坐标之间一些简单操作
- Krpano学习:在C#中修改全景场景属性(C#操作全景vtour.xml文件及相关瓦片数据/panos/*.tiles)
- C#学习笔记21——文件、目录、注册表操作
- C#源码学习之---事件驱动异步文件操作
- C#高级学习第七章-文件操作
- C#学习6,文件操作相关学习
- C#学习与上位机开发之文件操作(EXCEL保存案例)
- C#学习第十天 文件操作、文件流
- C#学习笔记之操作配置文件
- c#之文件操作(学习笔记)
- xml学习(三)---利用xml文件实现数据岛功能(网页显示xml文件中的内容免C#代码操作xml文件)
- C#学习之文件操作
- C#学习笔记:文件操作
- c#学习笔记之文件操作
- C#学习笔记 文件操作
- (C#)学习笔记1:文件和注册表操作
- C#学习——文件和数据流操作
- c#学习笔记之文件操作