链表初解(二)——双链表的创建、删除、插入
2014-03-28 23:49
387 查看
下面是基本的双链表操作,由于双链表有两个方向,所以在删除和插入节点时,可以节省一个指针,只用一个链表上的指针和一个待操作的指针即可完成插入和删除;同时也要注意在编写双链表时对情况的判断要仔细,否则很容易出错~
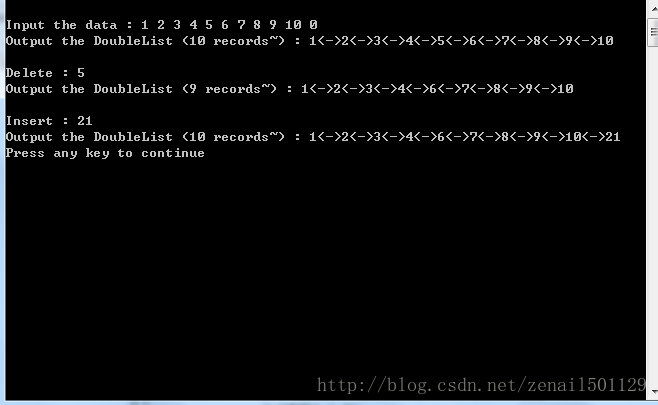
Ps:仅供参考~~
#include<iostream> using namespace std; typedef struct student { int data; struct student *next; struct student *pre; }dnode; //建立双链表 dnode *create() { dnode *head, *p, *s; int x; head = (dnode *)malloc(sizeof(dnode)); p = head; cout<<"\nInput the data : "; while(1) { if(scanf("%d", &x) != EOF && x != 0) { s = (dnode *)malloc(sizeof(dnode)); s->data = x; p->next = s; s->pre = p; p = s; } else break; } head = head->next; head->pre = NULL; p->next = NULL; return head; } //双链表删除节点 dnode *del(dnode *head, int num) { dnode *p1; p1 = head; while(num != p1->data && p1->next != NULL) p1 = p1->next; if(num == p1->data) { if(head == p1) { head = head->next; head->pre = NULL; }/*此处的处理与单链表不同,因为双链表有两个方向,所以在定义时仅定义一个指针, 这使得当出现删除链表尾部时,由于NULL没有前驱,导致无法连接,所以要分开讨论。*/ else if(p1->next == NULL) p1->pre->next = NULL; else {//p1为最后一个节点,如没有上一个分支,则第二个语句会出错。 p1->pre->next = p1->next; p1->next->pre = p1->pre; } free(p1); //释放 } else cout<<"There is no %d "<<num; return head; } //双链表插入节点 dnode *insert(dnode *head, int num) { dnode *p0, *p1; p1 = head; p0 = (dnode *)malloc(sizeof(dnode)); p0->data = num; while(num > p1->data && p1->next != NULL) p1 = p1->next; if(num <= p1->data) { if(head == p1) { p0->next = p1; p1->pre = p0; head = p0; } else { p1->pre->next = p0; p0->pre = p1->pre; p0->next = p1; p1->pre = p0; } } else {//插入尾部~ p1->next = p0; p0->next = NULL; p0->pre = p1; } return head; } //计算表长 int length(dnode *head) { int n = 0; while(head != NULL) { head = head->next; n++; } return n; } //打印双链表 void print(dnode *head) { int n = length(head); cout<<"Output the DoubleList ("<<n<<" records~) : "; while(head != NULL) { if(head->next == NULL) cout<<head->data<<endl; else cout<<head->data<<"<->"; head = head->next; } } //排序函数同单链表,此处省略了~,默认输入为递增哈~ int main() { dnode *head; //创建 head = create(); print(head); //删除 int numD; cout<<"\nDelete : "; cin>>numD; print(del(head, numD)); //插入 int numS; cout<<"\nInsert : "; cin>>numS; print(insert(head, numS)); return 0; }
Ps:仅供参考~~
相关文章推荐
- 链表的创建、插入、删除、排序和逆置
- 链表的创建、取长、输出、插入、删除、逆序
- 单向链表的基本操作-创建、插入、删除
- 链表的创建、遍历、节点的插入、节点的删除
- 链表(创建,插入,删除和打印输出)
- 数据结构学习(五)——循环双链表的操作之创建,插入、删除
- 线性表的链式存储格式基本操作:创建链表、插入、删除、查找、求表长、打印链表
- (C语言版)链表(二)——实现单向循环链表创建、插入、删除、释放内存等简单操作
- C++中链表的创建、输出、节点删除、节点插入、翻转、清空
- 链表(1)基本操作:创建,插入,删除,销毁等(模板类实现)
- 用c语言完成一个双向链表的创建,插入,删除
- 单链表中头结点的有无. 并讨论下有无头结点在单链表的创建,打印,插入,逆置,删除中的区别.
- 链表操作:创建,插入,删除,查找等功能
- 链表的创建、插入、删除等操作
- 完整的链表操作(定义-创建-插入-删除-输出)
- C语言各种链表操作(创建、打印、删除、插入、反转)
- 单向链表创建、插入、删除
- 链表的创建、插入、删除操作
- 链表的创建、插入、删除
- 对链表进行创建、结点的删除和插入操作