Spring-DI-注解
2014-03-10 20:55
447 查看
注解
* 必须依赖于类中的某一个部分
* 注解应该在类的哪些部位出现
* 自定义注解
* 了解jdk内置的注解
* 解析注解
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd"> <!--
1、导入命名空间
xmlns:context="http://www.springframework.org/schema/context" http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd
2、到入依赖注入的注解解析器
<context:annotation-config></context:annotation-config>
3、把student和person导入进来
-->
<context:annotation-config></context:annotation-config>
<bean id="student" class="cn.itcast.spring0909.di.annotation.Student"></bean>
<bean id="person" class="cn.itcast.spring0909.di.annotation.Person"></bean>
</beans>
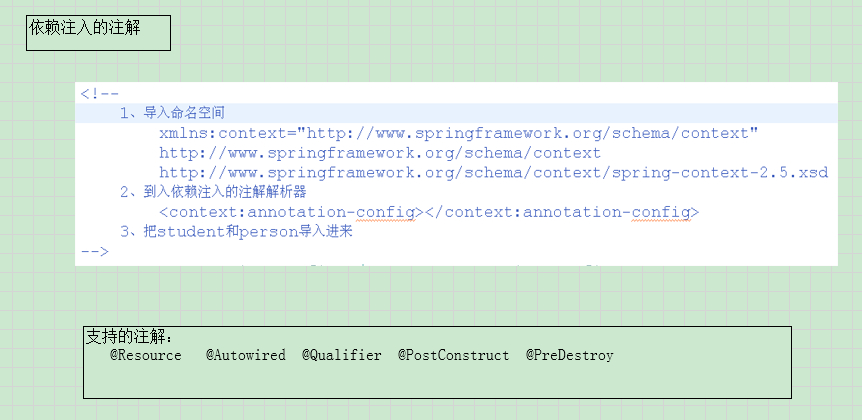
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd"> <!--
1、导入命名空间
xmlns:context="http://www.springframework.org/schema/context" http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd
2、启动类扫描的注解解析器
3、启动依赖注入的注解解析器
-->
<!--
component就是bean
base-package
会在base-package的值所在的包及子包下扫描所有的类
-->
<context:component-scan base-package="cn.itcast.spring0909.scan"></context:component-scan>
</beans>
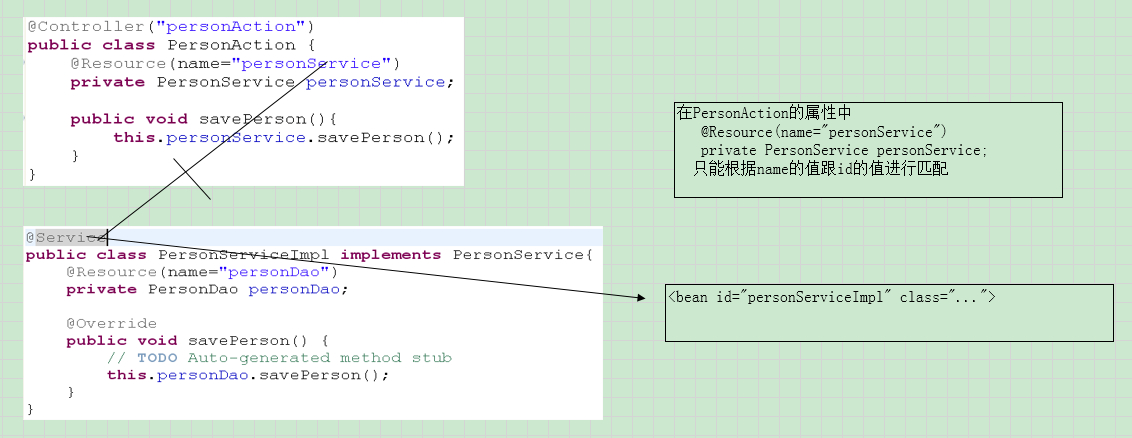
* 必须依赖于类中的某一个部分
* 注解应该在类的哪些部位出现
* 自定义注解
* 了解jdk内置的注解
* 解析注解
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd"> <!--
1、导入命名空间
xmlns:context="http://www.springframework.org/schema/context" http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd
2、到入依赖注入的注解解析器
<context:annotation-config></context:annotation-config>
3、把student和person导入进来
-->
<context:annotation-config></context:annotation-config>
<bean id="student" class="cn.itcast.spring0909.di.annotation.Student"></bean>
<bean id="person" class="cn.itcast.spring0909.di.annotation.Person"></bean>
</beans>
/** * 原理 * * 启动spring容器,并且加载配置文件 * * 会为student和person两个类创建对象 * * 当解析到<context:annotation-config></context:annotation-config> * 会启动依赖注入的注解解析器 * * 会在纳入spring管理的bean的范围内查找看哪些bean的属性上有@Resource注解 * * 如果@Resource注解的name属性的值为"",则会把注解所在的属性的名称和spring容器中bean的id进行匹配 * 如果匹配成功,则把id对应的对象赋值给该属性,如果匹配不成功,则按照类型进行匹配,如果再匹配不成功,则报错 * * 如果@Resource注解的name属性的值不为"",会把name属性的值和spring容器中bean的id做匹配,如果匹配 * 成功,则赋值,如果匹配不成功 ,则直接报错 * 说明: * 注解只能用于引用类型 * @author Administrator * */ public class PersonTest extends SpringHelper{ static{ path = "cn/itcast/spring0909/di/annotation/applicationContext.xml"; } @Test public void test(){ ClassPathXmlApplicationContext applicationContext = (ClassPathXmlApplicationContext)context; Person person = (Person)applicationContext.getBean("person"); person.say(); applicationContext.close(); } }
package cn.itcast.spring0909.di.annotation; import javax.annotation.PostConstruct; import javax.annotation.PreDestroy; import javax.annotation.Resource; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; public class Person { //@Autowired//按照类型进行匹配 //@Qualifier("student") @Resource(name="student") private Student studen; @Resource// 这行会报错(所以注解只能用于引用类型) private Long pid; public void say(){ this.studen.say(); } @PostConstruct public void init(){ System.out.println("init"); } public Person() { super(); this.pid=1L; } @PreDestroy public void destroy(){ System.out.println("destroy"); } }
package cn.itcast.spring0909.di.annotation; public class Student { public void say(){ System.out.println("student"); System.err.println("xxxxxxxx"); } }
SCAN
applicationContext.xml<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd"> <!--
1、导入命名空间
xmlns:context="http://www.springframework.org/schema/context" http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd
2、启动类扫描的注解解析器
3、启动依赖注入的注解解析器
-->
<!--
component就是bean
base-package
会在base-package的值所在的包及子包下扫描所有的类
-->
<context:component-scan base-package="cn.itcast.spring0909.scan"></context:component-scan>
</beans>
/** * 原理 * * 启动spring容器,加载配置文件 * * spring容器解析到 * <context:component-scan base-package="cn.itcast.spring0909.scan"></context:component-scan> * * spring容器会在指定的包及子包中查找类上是否有@Component * * 如果@Component注解没有写任何属性 * @Component * public class Person{ * * } * == * <bean id="person" class="..Person"> * 如果@Component("aa") * @Component * public class Person{ * * } * == * <bean id="aa" class="..Person"> * * 在纳入spring管理的bean的范围内查找@Resource注解 * * 执行@Resource注解的过程 * 说明: * xml效率比较高,但是书写比较麻烦 * 注解效率比较低,书写比较简单 * @author Administrator * */ public class PersonTest extends SpringHelper{ static{ path = "cn/itcast/spring0909/scan/applicationContext.xml"; } @Test public void test(){ Person person = (Person)context.getBean("perso"); person.say(); } }
@Component("perso")//加上这个注解 就可以让di容器自动扫描了 public class Person { @Resource(name="student") private Student studen; private Long pid; public void say(){ this.studen.say(); } @PostConstruct public void init(){ System.out.println("init"); } @PreDestroy public void destroy(){ System.out.println("destroy"); } }
@Component public class Student { public void say(){ System.out.println("student"); } }
mvc注解
applicationContext.xml<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd"> <context:component-scan base-package="cn.itcast.spring0909.mvc.spring.annotation"> </context:component-scan> </beans>
/** * 如果一个类中有基本类型,并且基本类型是用spring的形式赋值的,这个时候,该类必须用xml来完成,不能用注解 * @author Administrator * */ @Controller("personAction") public class PersonAction { @Resource private PersonService personService; public void savePerson(){ this.personService.savePerson(); } }
public interface PersonDao { public void savePerson(); }
@Repository("personDao") public class PersonDaoImpl implements PersonDao{ @Override public void savePerson() { // TODO Auto-generated method stub System.out.println("save person"); } }
public interface PersonService { public void savePerson(); }
@Service public class PersonServiceImpl implements PersonService{ @Resource(name="personDao") private PersonDao personDao; @Override public void savePerson() { // TODO Auto-generated method stub this.personDao.savePerson(); } }
public class PersonTest extends SpringHelper{ static{ path = "cn/itcast/spring0909/mvc/spring/annotation/applicationContext.xml"; } @Test public void test(){ PersonAction personAction = (PersonAction)context.getBean("personAction"); personAction.savePerson(); } }
相关文章推荐
- Java文件操作(求各专业第一名的学生)
- java读取各类型的文件
- 丁老师布置的作业,玛一段7-6的源程序(Bwriter.java)
- java正反金字塔程序
- java之线程
- Spring是什么
- Struts2配置问题
- java 第四天 面向对象(基础篇之引用传递及基本应用)
- Eclipse中使用自定义模板来弥补Myeclipse没有新建Filter的功能
- 华为上机题汇总----java
- 华为上机题汇总----java
- java字符
- java图片动态添加水印(利用Graphics2D)
- eclipse导入系统签名
- Spring 验证码(kaptcha)
- spring面试题
- c2java 第0篇
- java 调用C#的webservice
- javah 生成jni头文件。
- JAVA核心技术 第四章 对象与类 类设计技巧