原来还有这样的记词方法_Java版记不规则动词_博主推荐
2014-02-28 21:00
561 查看
昨天在看一本英语书的不规则动词的时候,突然产生的灵感:就是想把这样记单词简单方式,用程序代码实现,然后,使用户可以与之进行交互
这样,在用户背不规则动词的时候就会轻松把它给记住。基于这一点,于是我就思考了一下,画了画图,理了一下思路。然后就开始着手开干。
现在基本成型了,也可以和大家见面了。
先看看一些截图,这样比较直接一点
项目结构:
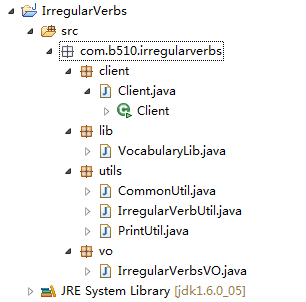
效果图:
用户可以输入命令:
"all" : 输出所有的不规则动词信息
"pronunciation" : 单词的发音,该命令时一个发音开关,如果连续输入,效果则是:开,关,开,关...
"exit" : 退出系统
其他:如果用户输入的在库中没有,系统则会给出一些联想的词汇供用户选择,如:"drea", 则供选择的是 "dream"
如果用户输入的在库中,如:"dream", 系统会输出"dream"的详细信息,这样用户就可以轻松和系统交互,并且在这一
过程中把单词给记住。
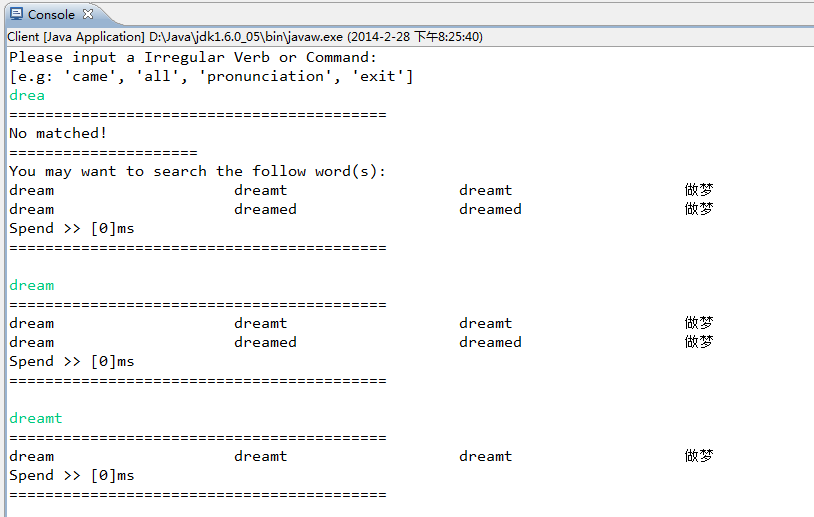
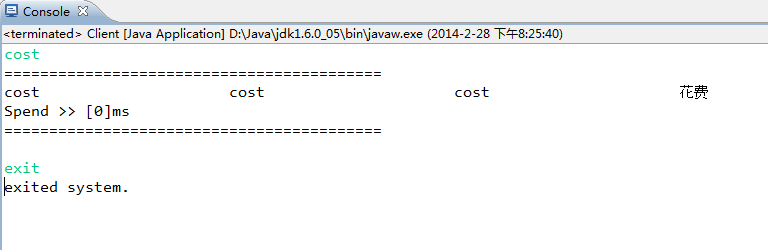
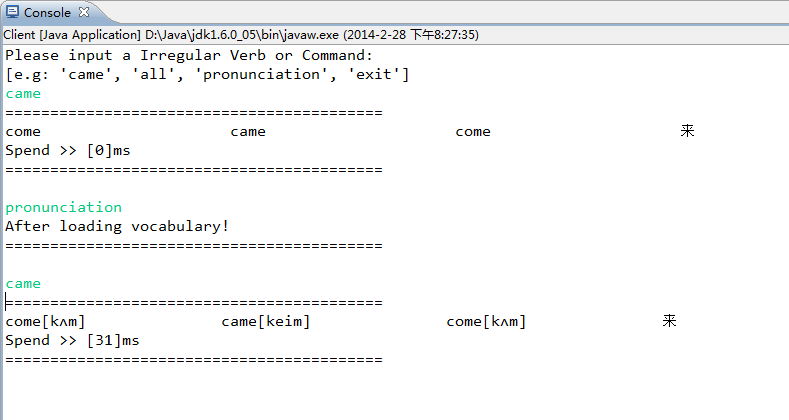
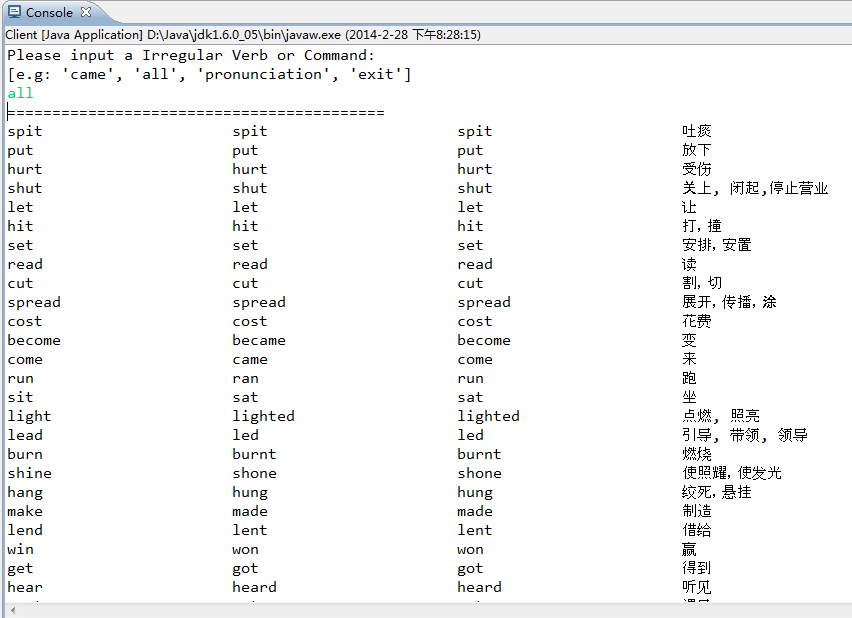
看完效果图,我想大家都基本上了解这个小程序的功能和作用吧!
=================================================
Source Code
Description:
User input some commands in the console,then the system will receive the command
and process it, and display the result to user.
e.g:
ONE : When user input the command "all", then the system will display all irregular verbs.
TWO : When user input the command "pronunciation", then the system will change the status of the pronunciation.
THREE : When user input the a irregular verb, then the system will diplay the detial of the word.
=================================================
/IrregularVerbs/src/com/b510/irregularverbs/client/Client.java
/IrregularVerbs/src/com/b510/irregularverbs/lib/VocabularyLib.java
/IrregularVerbs/src/com/b510/irregularverbs/utils/CommonUtil.java
/IrregularVerbs/src/com/b510/irregularverbs/utils/IrregularVerbUtil.java
/IrregularVerbs/src/com/b510/irregularverbs/utils/PrintUtil.java
/IrregularVerbs/src/com/b510/irregularverbs/vo/IrregularVerbsVO.java
===============================================
Download Source Code : http://files.cnblogs.com/hongten/IrregularVerbs.zip
===============================================
这样,在用户背不规则动词的时候就会轻松把它给记住。基于这一点,于是我就思考了一下,画了画图,理了一下思路。然后就开始着手开干。
现在基本成型了,也可以和大家见面了。
先看看一些截图,这样比较直接一点
项目结构:
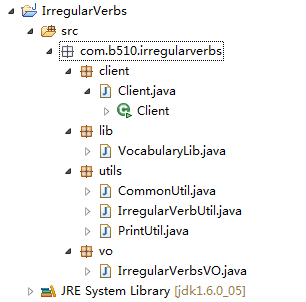
效果图:
用户可以输入命令:
"all" : 输出所有的不规则动词信息
"pronunciation" : 单词的发音,该命令时一个发音开关,如果连续输入,效果则是:开,关,开,关...
"exit" : 退出系统
其他:如果用户输入的在库中没有,系统则会给出一些联想的词汇供用户选择,如:"drea", 则供选择的是 "dream"
如果用户输入的在库中,如:"dream", 系统会输出"dream"的详细信息,这样用户就可以轻松和系统交互,并且在这一
过程中把单词给记住。
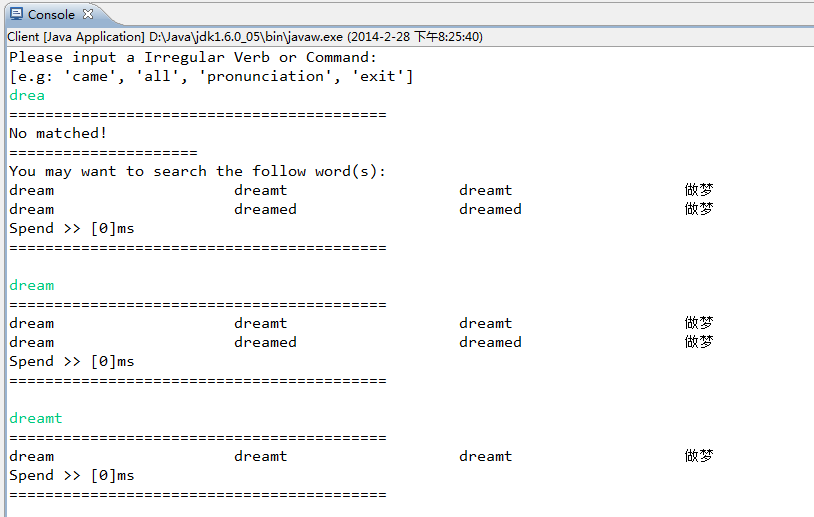
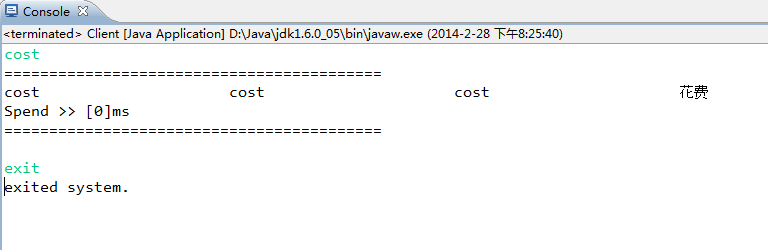
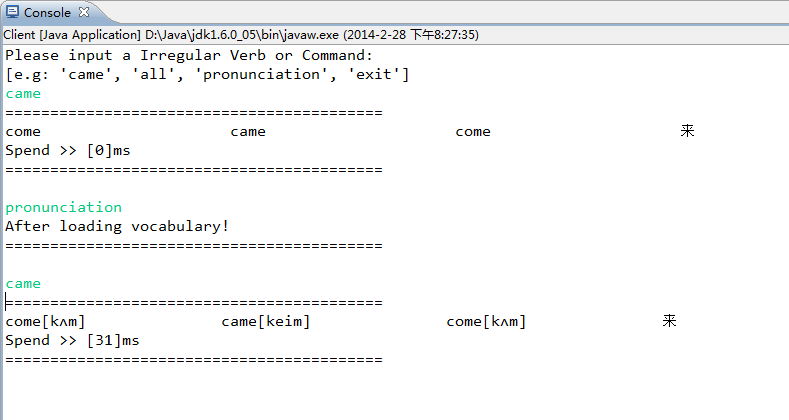
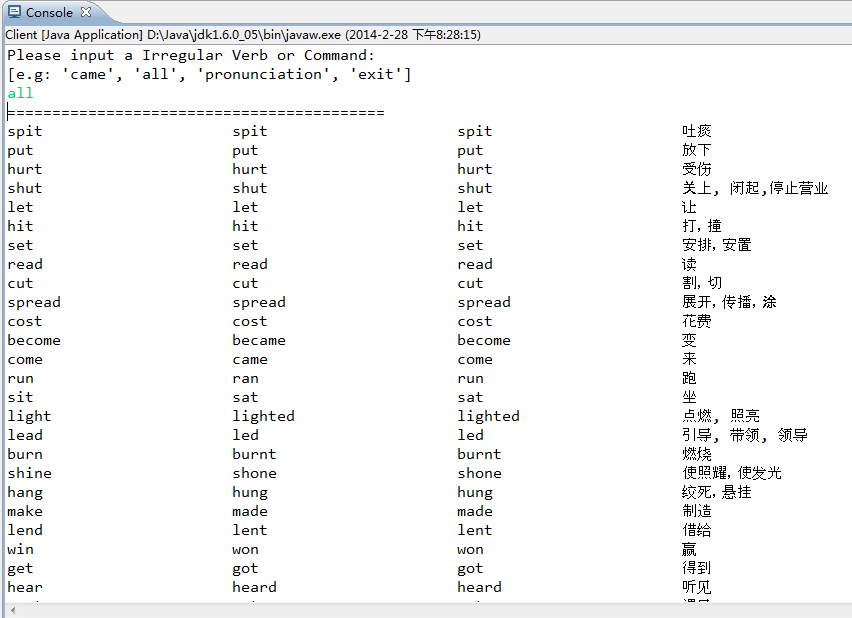
看完效果图,我想大家都基本上了解这个小程序的功能和作用吧!
=================================================
Source Code
Description:
User input some commands in the console,then the system will receive the command
and process it, and display the result to user.
e.g:
ONE : When user input the command "all", then the system will display all irregular verbs.
TWO : When user input the command "pronunciation", then the system will change the status of the pronunciation.
THREE : When user input the a irregular verb, then the system will diplay the detial of the word.
=================================================
/IrregularVerbs/src/com/b510/irregularverbs/client/Client.java
/** * */ package com.b510.irregularverbs.client; import java.util.Scanner; import com.b510.irregularverbs.utils.CommonUtil; import com.b510.irregularverbs.utils.IrregularVerbUtil; import com.b510.irregularverbs.utils.PrintUtil; /** * User input some commands in the console,then the system will receive<br> * the command and process it, and display the result to user.<br> * for example: * <li>all : show the all of the Irregular Verbs</li> * <li>pronunciation : the switch of the pronunciation.and the system default status<br> * is not pronunciation.</li> * <li>The Irregular Verbs : user input the irregular verb,for example: "swim", "cost", "became".etc.</li> * @author Hongten * @created Feb 27, 2014 */ public class Client { private Scanner inputStreamScanner; private boolean controlFlag = false; public static void main(String[] args) { Client client = new Client(); client.listenConsoleAndHandleContent(); } public Client() { inputStreamScanner = new Scanner(System.in); } /** * Listen to the console and handle the content,what user inputed. */ public void listenConsoleAndHandleContent() { PrintUtil.printInfo(CommonUtil.DESCRIPTION); try { while (!controlFlag && inputStreamScanner.hasNext()) { processingConsoleInput(); } } catch (Exception e) { e.printStackTrace(); } finally { closeScanner(); } } /** * The system receive the content, and process it. */ private void processingConsoleInput() { String inputContent = inputStreamScanner.nextLine(); if (!inputContent.isEmpty()) { if (pronounce(inputContent)) { IrregularVerbUtil.reLoadVerbs(); } else { if (showAll(inputContent)) { return; } controlFlag = exit(inputContent); if (!controlFlag) { notExit(inputContent); } } } } /** * When user input the command "all"(ignore case),the system will handle the command "all",<br> * and display the all of the irregular verbs. * @param inputContent the command "all"(ignore case) * @return true, if user input the command "all"(ignore case);return false,if user input other command. */ private boolean showAll(String inputContent) { if (CommonUtil.ALL.equalsIgnoreCase(inputContent)) { IrregularVerbUtil.showAll(); return true; } else { return false; } } /** * When user input the command "pronunciation"(ignore case), the system will handle the command "pronunciation",<br> * and reload all of the irregular verbs. and display the message "After loading vocabulary!". * @param inputContent the command "pronunciation"(ignore case) * @return true, if user input the command "pronunciation"(ignore case);return false, if user input other command. */ private boolean pronounce(String inputContent) { if (CommonUtil.PRONUNCIATION.equalsIgnoreCase(inputContent)) { IrregularVerbUtil.proFlag = !IrregularVerbUtil.proFlag; return true; } else { return false; } } private void notExit(String inputContent) { IrregularVerbUtil verbsLib = IrregularVerbUtil.getInstance(); verbsLib.match(inputContent); } private boolean exit(String inputContent) { if (CommonUtil.EXIT.equalsIgnoreCase(inputContent)) { controlFlag = true; systemExit(); } return controlFlag; } private void systemExit() { System.out.println(CommonUtil.EXIT_SYSTEM); System.exit(0); } private void closeScanner() { inputStreamScanner.close(); } }
/IrregularVerbs/src/com/b510/irregularverbs/lib/VocabularyLib.java
/** * */ package com.b510.irregularverbs.lib; import java.util.HashMap; import java.util.Iterator; import java.util.List; import java.util.Map; import com.b510.irregularverbs.utils.CommonUtil; import com.b510.irregularverbs.utils.IrregularVerbUtil; import com.b510.irregularverbs.vo.IrregularVerbsVO; /** * Library for the irregular verbs. * @author Hongten * @created Feb 28, 2014 */ public class VocabularyLib { /** * Load all of the irregular verbs.<br> * There have FOUR types of the irregular verbs : "AAA", "ABA", "ABB", "ABC".<br> * so,there are FOUR methods to load all irregular verbs. * @return all of the irregular verbs. */ public static List<IrregularVerbsVO> getVocabularyLib() { formatAAA(); formatABA(); formatABB(); formatABC(); return IrregularVerbUtil.verbs; } /** * The "AAA" format for irregular verbs. * * @return */ private static List<IrregularVerbsVO> formatAAA() { Map<String, String> aaaTypemap = libAAA(); Iterator<String> it = aaaTypemap.keySet().iterator(); while (it.hasNext()) { handleAAA(aaaTypemap, it); } return IrregularVerbUtil.verbs; } /** * @param aaaTypemap * @param it */ private static void handleAAA(Map<String, String> aaaTypemap, Iterator<String> it) { String key = it.next(); if (IrregularVerbUtil.proFlag) { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(key, key, key, aaaTypemap.get(key))); } else { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(key, key, key, aaaTypemap.get(key))); } } /** * The "ABA" format for irregular verbs * * @return */ private static List<IrregularVerbsVO> formatABA() { Map<String, String> abaTypemap = libABA(); Iterator<String> it = abaTypemap.keySet().iterator(); while (it.hasNext()) { handleABA(abaTypemap, it); } return IrregularVerbUtil.verbs; } /** * @param abaTypemap * @param it */ private static void handleABA(Map<String, String> abaTypemap, Iterator<String> it) { String key = it.next(); String[] keys = key.split(CommonUtil.COMMA); if (IrregularVerbUtil.proFlag) { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(keys[0] + CommonUtil.COMMA + keys[1], keys[2] + CommonUtil.COMMA + keys[3], keys[0] + CommonUtil.COMMA + keys[1], abaTypemap .get(key))); } else { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(keys[0], keys[1], keys[0], abaTypemap.get(key))); } } /** * The "ABB" format for irregular verbs * * @return */ private static List<IrregularVerbsVO> formatABB() { Map<String, String> abbTypemap = libABB(); Iterator<String> it = abbTypemap.keySet().iterator(); while (it.hasNext()) { handleABB(abbTypemap, it); } return IrregularVerbUtil.verbs; } /** * @param abbTypemap * @param it */ private static void handleABB(Map<String, String> abbTypemap, Iterator<String> it) { String key = it.next(); String[] keys = key.split(CommonUtil.COMMA); if (IrregularVerbUtil.proFlag) { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(keys[0] + CommonUtil.COMMA + keys[1], keys[2] + CommonUtil.COMMA + keys[3], keys[2] + CommonUtil.COMMA + keys[3], abbTypemap.get(key))); } else { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(keys[0], keys[1], keys[1], abbTypemap.get(key))); } } /** * The "ABC" format for irregular verbs * * @return */ private static List<IrregularVerbsVO> formatABC() { Map<String, String> abaTypemap = libABC(); Iterator<String> it = abaTypemap.keySet().iterator(); while (it.hasNext()) { handleABC(abaTypemap, it); } return IrregularVerbUtil.verbs; } /** * @param abaTypemap * @param it */ private static void handleABC(Map<String, String> abaTypemap, Iterator<String> it) { String key = it.next(); String[] keys = key.split(CommonUtil.COMMA); if (IrregularVerbUtil.proFlag) { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(keys[0] + CommonUtil.COMMA + keys[1], keys[2] + CommonUtil.COMMA + keys[3], keys[4] + CommonUtil.COMMA + keys[5], abaTypemap .get(key))); } else { IrregularVerbUtil.verbs.add(new IrregularVerbsVO(keys[0], keys[1], keys[2], abaTypemap.get(key))); } } //============================================ // Library for all of the irregular verbs //============================================ /** * Library for "AAA" type. * @return */ private static Map<String, String> libAAA() { Map<String, String> aaaTypemap = new HashMap<String, String>(); aaaTypemap.put(IrregularVerbUtil.proFlag ? "cost,[kɔst]" : "cost", "花费"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "cut,[kʌt]" : "cut", "割,切"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "hurt,[hə:t]" : "hurt", "受伤"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "hit,[hit]" : "hit", "打,撞"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "let,[let]" : "let", "让"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "put,[put]" : "put", "放下"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "read,[ri:d]" : "read", "读"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "set,[set]" : "set", "安排,安置"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "spread,[spred]" : "spread", "展开,传播,涂"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "spit,[spit]" : "spit", "吐痰"); aaaTypemap.put(IrregularVerbUtil.proFlag ? "shut,[ʃʌt]" : "shut", "关上, 闭起,停止营业"); return aaaTypemap; } /** * Library for "ABA" type. * @return */ private static Map<String, String> libABA() { Map<String, String> abaTypemap = new HashMap<String, String>(); abaTypemap.put(IrregularVerbUtil.proFlag ? "become,[bi'kʌm],became,[bi'keim]" : "become,became", "变"); abaTypemap.put(IrregularVerbUtil.proFlag ? "come,[kʌm],came,[keim]" : "come,came", "来"); abaTypemap.put(IrregularVerbUtil.proFlag ? "run,[rʌn],ran,[ræn]" : "run,ran", "跑"); return abaTypemap; } /** * Library for "ABB" type. * @return */ private static Map<String, String> libABB() { Map<String, String> abbTypemap = new HashMap<String, String>(); abbTypemap.put(IrregularVerbUtil.proFlag ? "burn,[bə:n],burnt,[bə:nt]" : "burn,burnt", "燃烧"); abbTypemap.put(IrregularVerbUtil.proFlag ? "deal,[di:l],dealt,[delt]" : "deal,dealt", "解决"); abbTypemap.put(IrregularVerbUtil.proFlag ? "dream,[dri:m],dreamed,[dremt]" : "dream,dreamed", "做梦"); abbTypemap.put(IrregularVerbUtil.proFlag ? "dream,[dri:m],dreamt,[dremt]" : "dream,dreamt", "做梦"); abbTypemap.put(IrregularVerbUtil.proFlag ? "hear,[hiə],heard,[hə:d]" : "hear,heard", "听见"); abbTypemap.put(IrregularVerbUtil.proFlag ? "hang,['hæŋ],hanged,[]" : "hang,hanged", "绞死,悬挂"); abbTypemap.put(IrregularVerbUtil.proFlag ? "hang,['hæŋ],hung,[hʌŋ]" : "hang,hung", "绞死,悬挂"); abbTypemap.put(IrregularVerbUtil.proFlag ? "learn,[lə:n],learned,[]" : "learn,learned", "学习"); abbTypemap.put(IrregularVerbUtil.proFlag ? "learn,[lə:n],learnt,[lə:nt]" : "learn,learnt", "学习"); abbTypemap.put(IrregularVerbUtil.proFlag ? "light,['lait],lit,[lit]" : "light,lit", "点燃, 照亮"); abbTypemap.put(IrregularVerbUtil.proFlag ? "light,['lait],lighted,[]" : "light,lighted", "点燃, 照亮"); abbTypemap.put(IrregularVerbUtil.proFlag ? "mean,[mi:n],meant,[ment]" : "mean,meant", "意思"); abbTypemap.put(IrregularVerbUtil.proFlag ? "prove,[pru:v],proved,[]" : "prove,proved", "证明, 证实,试验"); abbTypemap.put(IrregularVerbUtil.proFlag ? "shine,[ʃain],shone,[ʃəun]" : "shine,shone", "使照耀,使发光"); abbTypemap.put(IrregularVerbUtil.proFlag ? "shine,[ʃain],shined,[]" : "shine,shined", "使照耀,使发光"); abbTypemap.put(IrregularVerbUtil.proFlag ? "show,[ʃəu],showed,[]" : "show,showed", "展示, 给...看"); abbTypemap.put(IrregularVerbUtil.proFlag ? "smell,[smel],smelled,[]" : "smell,smelled", "闻, 嗅"); abbTypemap.put(IrregularVerbUtil.proFlag ? "smell,[smel],smelt,[smelt]" : "smell,smelt", "闻, 嗅"); abbTypemap.put(IrregularVerbUtil.proFlag ? "speed,[spi:d],sped,[sped]" : "speed,sped", "加速"); abbTypemap.put(IrregularVerbUtil.proFlag ? "speed,[spi:d],speeded,[]" : "speed,speeded", "加速"); abbTypemap.put(IrregularVerbUtil.proFlag ? "spell,[spel],spelled,[]" : "spell,spelled", "拼写"); abbTypemap.put(IrregularVerbUtil.proFlag ? "spell,[spel],spelt,[spelt]" : "spell,spelt", "拼写"); abbTypemap.put(IrregularVerbUtil.proFlag ? "wake,[weik],waked,[]" : "wake,waked", "醒来,叫醒, 激发"); abbTypemap.put(IrregularVerbUtil.proFlag ? "wake,[weik],woke,[wəuk]" : "wake,woken", "醒来,叫醒, 激发"); abbTypemap.put(IrregularVerbUtil.proFlag ? "build,[bild],built,[bilt]" : "build,built", "建筑"); abbTypemap.put(IrregularVerbUtil.proFlag ? "lend,[lend],lent,[lent]" : "lend,lent", "借给"); abbTypemap.put(IrregularVerbUtil.proFlag ? "rebuild,[ri:'bild],rebuilt,[ri:'bilt]" : "rebuild,rebuilt", "改建, 重建"); abbTypemap.put(IrregularVerbUtil.proFlag ? "send,[send],sent,[sent]" : "send,sent", "送"); abbTypemap.put(IrregularVerbUtil.proFlag ? "spend,[spend],spent,[spent]" : "spend,spent", "花费"); abbTypemap.put(IrregularVerbUtil.proFlag ? "bring,[briŋ],brought,[brɔ:t]" : "bring,brought", "带来"); abbTypemap.put(IrregularVerbUtil.proFlag ? "buy,[bai],bought,[bɔ:t]" : "buy,bought", "买"); abbTypemap.put(IrregularVerbUtil.proFlag ? "fight,[fait],fought,[fɔ:t]" : "fight,fought", "打架"); abbTypemap.put(IrregularVerbUtil.proFlag ? "think,[θiŋk],thought,[θɔ:t] " : "think,though", "思考,想"); abbTypemap.put(IrregularVerbUtil.proFlag ? "catch,[kætʃ],caught,[kɔ:t]" : "catch,caught", "捉,抓"); abbTypemap.put(IrregularVerbUtil.proFlag ? "teach,[ti:tʃ],taught,[tɔ:t]" : "teach,taught", "教"); abbTypemap.put(IrregularVerbUtil.proFlag ? "dig,[diɡ],dug,[dʌɡ]" : "dig,dug", "掘(土), 挖(洞、沟等)"); abbTypemap.put(IrregularVerbUtil.proFlag ? "feed,[fi:d],fed,[fed]" : "feed,fed", "喂"); abbTypemap.put(IrregularVerbUtil.proFlag ? "find,[faind],found,[]" : "find,found", "发现,找到"); abbTypemap.put(IrregularVerbUtil.proFlag ? "get,[ɡet],got,[ɡɔt]" : "get,got", "得到"); abbTypemap.put(IrregularVerbUtil.proFlag ? "hold,[həuld],held,[held]" : "hold,held", "拥有,握住,支持"); abbTypemap.put(IrregularVerbUtil.proFlag ? "lead,[li:d],led,[led]" : "lead,led", "引导, 带领, 领导"); abbTypemap.put(IrregularVerbUtil.proFlag ? "meet,[mi:t],met,[met]" : "meet,met", "遇见"); abbTypemap.put(IrregularVerbUtil.proFlag ? "sit,[sit],sat,[sæt]" : "sit,sat", "坐"); abbTypemap.put(IrregularVerbUtil.proFlag ? "shoot,[ʃu:t],shot,[ʃɔt]" : "shoot,shot", "射击"); abbTypemap.put(IrregularVerbUtil.proFlag ? "spit,[spit],spit,[]" : "spit,spit", "吐痰"); abbTypemap.put(IrregularVerbUtil.proFlag ? "spit,[spit],spat,[spæt]" : "spit,spat", "吐痰"); abbTypemap.put(IrregularVerbUtil.proFlag ? "stick,[stik],stuck,[stʌk]" : "stick,stuck", "插进, 刺入, 粘住"); abbTypemap.put(IrregularVerbUtil.proFlag ? "win,[win],won,[wʌn]" : "win,won", "赢"); abbTypemap.put(IrregularVerbUtil.proFlag ? "feel,['fi:l],felt,[felt]" : "feel,felt", "感到"); abbTypemap.put(IrregularVerbUtil.proFlag ? "keep,[ki:p],kept,[kept]" : "keep,kept", "保持"); abbTypemap.put(IrregularVerbUtil.proFlag ? "leave,[li:v],left,[left]" : "leave,left", "离开"); abbTypemap.put(IrregularVerbUtil.proFlag ? "sleep,[sli:p],slept,[slept]" : "sleep,slept", "睡觉"); abbTypemap.put(IrregularVerbUtil.proFlag ? "sweep,[swi:p],swept,[swept]" : "sweep,swept", "扫"); abbTypemap.put(IrregularVerbUtil.proFlag ? "lay,[lei],laid,[leid]" : "lay,laid", "下蛋, 放置"); abbTypemap.put(IrregularVerbUtil.proFlag ? "pay,[pei],paid,[peid]" : "pay,paid", "付"); abbTypemap.put(IrregularVerbUtil.proFlag ? "say,[sei],said,[sed]" : "say,said", "说"); abbTypemap.put(IrregularVerbUtil.proFlag ? "stand,[stænd],stood,[stud]" : "stand,stood", "站"); abbTypemap.put(IrregularVerbUtil.proFlag ? "understand,[],understood,[ʌndə'stænd]" : "understand,understood", "明白"); abbTypemap.put(IrregularVerbUtil.proFlag ? "lose,[lu:z],lost,[lɔst]" : "lose,lost", "失去"); abbTypemap.put(IrregularVerbUtil.proFlag ? "have,[həv],had,[hæd]" : "have,had", "有"); abbTypemap.put(IrregularVerbUtil.proFlag ? "make,[meik],made,[meid]" : "make,made", "制造"); abbTypemap.put(IrregularVerbUtil.proFlag ? "sell,[sel],sold,[səuld]" : "sell,sold", "卖"); abbTypemap.put(IrregularVerbUtil.proFlag ? "tell,[tel],told,[təuld]" : "tell,told", "告诉"); abbTypemap.put(IrregularVerbUtil.proFlag ? "retell,[ri:'tel],retold,[ri:'təuld]" : "retell,retold", "重讲,重复,复述"); return abbTypemap; } /** * Library for "ABC" type. * @return */ private static Map<String, String> libABC() { Map<String, String> abaTypemap = new HashMap<String, String>(); abaTypemap.put(IrregularVerbUtil.proFlag ? "blow,[bləu],blew,[blu:],blown,[]" : "blow,blew,blown", "吹"); abaTypemap.put(IrregularVerbUtil.proFlag ? "drive,[draiv],drove,[drəuv],driven,[drivən]" : "drive,drove,driven", "驾驶"); abaTypemap.put(IrregularVerbUtil.proFlag ? "draw,[drɔ:],drew,[dru:],drawn,[drɔ:n]" : "draw,drew,drawn", "画画"); abaTypemap.put(IrregularVerbUtil.proFlag ? "eat,[i:t],ate,[eit],eaten,['i:tən]" : "eat,ate,eaten", "吃"); abaTypemap.put(IrregularVerbUtil.proFlag ? "fall,[fɔ:l],Fell,[fel],fallen,['fɔ:lən]" : "fall,fell,fallen", "落下"); abaTypemap.put(IrregularVerbUtil.proFlag ? "give,[ɡiv],gave,[ɡeiv],given,['ɡivən]" : "give,gave,given", "给"); abaTypemap.put(IrregularVerbUtil.proFlag ? "get,[ɡet],got,[ɡɔt],gotten,['ɡɔtən]" : "get,got,gotten", "得到"); abaTypemap.put(IrregularVerbUtil.proFlag ? "show,[ʃəu],showed,[],shown,['ʃəun]" : "show,showed,shown", "展示, 给...看"); abaTypemap.put(IrregularVerbUtil.proFlag ? "fall,[fɔ:l],Fell,[fel],fallen,['fɔ:lən]" : "prove,proved,proven", "证明, 证实,试验"); abaTypemap.put(IrregularVerbUtil.proFlag ? "give,[ɡiv],gave,[ɡeiv],given,['ɡivən]" : "beat,beat,beaten", "打败"); abaTypemap.put(IrregularVerbUtil.proFlag ? "grow,[ɡrəu],grew,[ɡru:],grown,[ɡrəun]" : "grow,grew,grown", "生长"); abaTypemap.put(IrregularVerbUtil.proFlag ? "forgive,[fə'ɡiv],forgot,[fə'ɡɔt],forgiven,[]" : "forgive,forgot,forgiven", "原谅, 饶恕"); abaTypemap.put(IrregularVerbUtil.proFlag ? "know,[nəu],knew,[nju: nu:],known,[]" : "know,knew,known", "知道"); abaTypemap.put(IrregularVerbUtil.proFlag ? "mistake,[mi'steik],mistook,[mi'stuk],mistooken,[]" : "mistake,mistook,mistooken", "弄错; 误解,"); abaTypemap.put(IrregularVerbUtil.proFlag ? "overeat,['əuvə'i:t],overate,[əuvə'reit],overeaten,[]" : "overeat,overate,overeaten", "(使)吃过量"); abaTypemap.put(IrregularVerbUtil.proFlag ? "take,[teik],took,[tuk],taken,['teikn]" : "take,took,taken", "拿"); abaTypemap.put(IrregularVerbUtil.proFlag ? "throw,[θrəu],threw,[θru:],thrown,[θrəun]" : "throw,threw,thrown", "抛,扔"); abaTypemap.put(IrregularVerbUtil.proFlag ? "ride,[raid],rode,[rəud],ridden,['ridən]" : "ride,rode,ridden", "骑"); abaTypemap.put(IrregularVerbUtil.proFlag ? "see,[si:],saw,[sɔ:],seen,[si:n]" : "see,saw,seen", "看见"); abaTypemap.put(IrregularVerbUtil.proFlag ? "write,[rait],wrote,[rəut],written,['ritən]" : "write,wrote,writen", "写"); abaTypemap.put(IrregularVerbUtil.proFlag ? "break,[breik],broke,[brəuk],broken,['brəukən]" : "break,broke,broken", "打破"); abaTypemap.put(IrregularVerbUtil.proFlag ? "choose,[tʃu:z],chose,[tʃəuz],chosen,['tʃəuzən]" : "choose,chose,chosen", "选择"); abaTypemap.put(IrregularVerbUtil.proFlag ? "hide,[haid],hid,[hid],hidden,['hidən]" : "hide,hid,hidden", "隐藏"); abaTypemap.put(IrregularVerbUtil.proFlag ? "forget,[fə'ɡet],forgot,[fə'ɡɔt],forgotten,[fə'ɡɔtn]" : "forget,forgot,forgotten", "忘记"); abaTypemap.put(IrregularVerbUtil.proFlag ? "freeze,[fri:z],froze,[frəuz],frozen,['frəuzn]" : "freeze,froze,frozen", "冷冻,结冰,感到严寒"); abaTypemap.put(IrregularVerbUtil.proFlag ? "speak,[spi:k],spoke,[spəuk],spoken,['spəukən]" : "speak,spoke,spoken", "说"); abaTypemap.put(IrregularVerbUtil.proFlag ? "steal,[sti:l],stole,[],stolen,['stəulən]" : "steal,stole,stolen", "偷"); abaTypemap.put(IrregularVerbUtil.proFlag ? "begin,[bi'ɡin],began,[bi'ɡæn],begun,[bi'ɡʌn]" : "begin,began,gegun", "开始"); abaTypemap.put(IrregularVerbUtil.proFlag ? "drink,[driŋk],drank,[dræŋk],drunk,[drʌŋk]" : "drink,drank,drunk", "喝"); abaTypemap.put(IrregularVerbUtil.proFlag ? "sing,[siŋ],sang,[sæŋ],sung,[sʌŋ]" : "sing,sang,sung", "唱"); abaTypemap.put(IrregularVerbUtil.proFlag ? "sink,[siŋk],sank,[sæŋk],sunk,[sʌŋk]" : "sink,sank,sunk", "下沉, 沉没"); abaTypemap.put(IrregularVerbUtil.proFlag ? "swim,[swim],swam,[swæm],swum,[swʌm]" : "swim,swam,swum", "游泳"); abaTypemap.put(IrregularVerbUtil.proFlag ? "ring,[riŋ],rang,[ræŋ],rung,[rʌŋ]" : "ring,rang,rung", "打电话"); abaTypemap.put(IrregularVerbUtil.proFlag ? "be,[],was,[],been,[]" : "be,was,been", "是"); abaTypemap.put(IrregularVerbUtil.proFlag ? "be,[],was,[],been,[]" : "be,were,been", "是"); abaTypemap.put(IrregularVerbUtil.proFlag ? "bear,[bεə],bore,[bɔ:],born,[]" : "bear,bore,born", "负担, 忍受"); abaTypemap.put(IrregularVerbUtil.proFlag ? "bear,[bεə],bore,[bɔ:],born,[bɔ:n]" : "bear,bore,borne", "负担, 忍受"); abaTypemap.put(IrregularVerbUtil.proFlag ? "do,[du:],did,[did],done,[dʌn]" : "do,did,done", "做"); abaTypemap.put(IrregularVerbUtil.proFlag ? "fly,[flai],flew,[flu:],flown,[fləun]" : "fly,flew,flown", "飞"); abaTypemap.put(IrregularVerbUtil.proFlag ? "go,[ɡəu],went,[went],gone,[ɡɔn]" : "go,went,gone", "去"); abaTypemap.put(IrregularVerbUtil.proFlag ? "lie,[lai],lay,[lei],lain,[lein]" : "lie,lay,lain", "躺"); abaTypemap.put(IrregularVerbUtil.proFlag ? "wear,[wεə],wore,[wɔ:],worn,[wɔ:n]" : "wear,wore,worn", "穿"); return abaTypemap; } }
/IrregularVerbs/src/com/b510/irregularverbs/utils/CommonUtil.java
/** * */ package com.b510.irregularverbs.utils; /** * @author Hongten * @created Feb 27, 2014 */ public class CommonUtil { //Commands public static final String EXIT = "exit"; public static final String PRONUNCIATION = "pronunciation"; public static final String ALL = "all"; //Description public static final String DESCRIPTION = "Please input a Irregular Verb or Command:\n[e.g: 'came', 'all', 'pronunciation', 'exit']"; public static final String EXIT_SYSTEM = "exited system."; public static final String LOADIGN_VERBS = "After loading vocabulary!"; public static final String NO_MATCHED = "No matched!"; public static final String ADVICE = "You may want to search the follow word(s):"; public static final String SPEND = "Spend >> ["; public static final String SPEND_END = "]ms"; public static final int STR_SIZE = 25; public static final String COMMA = ","; public static final String EMPTY = ""; public static final String BLANK = " "; public static final String LINE_ONE = "====================="; public static final String LINE_TWO = LINE_ONE + LINE_ONE; public static final String LINE_THREE = LINE_TWO+ "\n"; }
/IrregularVerbs/src/com/b510/irregularverbs/utils/IrregularVerbUtil.java
/** * */ package com.b510.irregularverbs.utils; import java.util.ArrayList; import java.util.List; import com.b510.irregularverbs.lib.VocabularyLib; import com.b510.irregularverbs.vo.IrregularVerbsVO; /** * The tool for the Irregular Verbs. Providing load and reload methods to get all irregular verbs.<br> * it also provide the match method to handle the content, which user input in the console.<br> * and display the result of matching. * @author Hongten * @created Feb 27, 2014 */ public class IrregularVerbUtil { private static IrregularVerbUtil irregularVerbs = new IrregularVerbUtil(); public static List<IrregularVerbsVO> verbs = new ArrayList<IrregularVerbsVO>(); // The switch of the pronunciation public static boolean proFlag = false; /** * Load all of the irregular verbs.<br> * @return all of the irregular verbs. */ public static List<IrregularVerbsVO> loadIrregularVerbsVOs() { return VocabularyLib.getVocabularyLib(); } private IrregularVerbUtil() { } public static IrregularVerbUtil getInstance() { if (verbs.size() <= 0) { loadIrregularVerbsVOs(); } return irregularVerbs; } /** * Reload all of the irregular verbs. */ public static void reLoadVerbs() { verbs = null; verbs = new ArrayList<IrregularVerbsVO>(); loadIrregularVerbsVOs(); PrintUtil.printInfo(CommonUtil.LOADIGN_VERBS); PrintUtil.printInfo(CommonUtil.LINE_THREE); } /** * Processing the input content,and display the result for matching. * @param input input content. */ public void match(String input) { long startTime = System.currentTimeMillis(); List<IrregularVerbsVO> temp = new ArrayList<IrregularVerbsVO>(); List<IrregularVerbsVO> mayBeVOs = new ArrayList<IrregularVerbsVO>(); for (IrregularVerbsVO vo : verbs) { handleMatchWord(input, temp, vo); // match same word(s) input = input.toLowerCase(); if (vo.getPrototype().startsWith(input) || vo.getPostTense().startsWith(input) || vo.getPostParticiple().startsWith(input)) { mayBeVOs.add(vo); } } PrintUtil.printInfo(CommonUtil.LINE_TWO); matchResult(temp, mayBeVOs); long endTime = System.currentTimeMillis(); PrintUtil.printInfo(CommonUtil.SPEND + (endTime - startTime) + CommonUtil.SPEND_END); PrintUtil.printInfo(CommonUtil.LINE_THREE); } /** * There have two results for matching.<br> * <li>First : matched, then display the result of matched.</li> * <li>Second : not matched, then display the related irregular verb(s)</li> * @param matchedList * @param relatedList */ private void matchResult(List<IrregularVerbsVO> matchedList, List<IrregularVerbsVO> relatedList) { if (matchedList.size() > 0) { loopMatchResult(matchedList); } else { PrintUtil.printInfo(CommonUtil.NO_MATCHED); if (relatedList.size() > 0) { PrintUtil.printInfo(CommonUtil.LINE_ONE); PrintUtil.printInfo(CommonUtil.ADVICE); loopMatchResult(relatedList); } } } /** * Display the match result * @param list */ private static void loopMatchResult(List<IrregularVerbsVO> list) { for (IrregularVerbsVO vo : list) { PrintUtil.printInfo(addBlank(vo.getPrototype().replace(CommonUtil.COMMA, CommonUtil.EMPTY)) + addBlank(vo.getPostTense().replace(CommonUtil.COMMA, CommonUtil.EMPTY)) + addBlank(vo.getPostParticiple().replace(CommonUtil.COMMA, CommonUtil.EMPTY)) + vo.getMean()); } } /** * @param input * @param temp * @param vo */ private void handleMatchWord(String input, List<IrregularVerbsVO> temp, IrregularVerbsVO vo) { if (proFlag) { if (input.equalsIgnoreCase(vo.getPrototype().split(CommonUtil.COMMA)[0]) || input.equalsIgnoreCase(vo.getPostTense().split(CommonUtil.COMMA)[0]) || input.equalsIgnoreCase(vo.getPostParticiple().split(CommonUtil.COMMA)[0])) { temp.add(vo); } } else { if (input.equalsIgnoreCase(vo.getPrototype()) || input.equalsIgnoreCase(vo.getPostTense()) || input.equalsIgnoreCase(vo.getPostParticiple())) { temp.add(vo); } } } /** * Show all of the irregular verbs. */ public static void showAll() { if (verbs.size() <= 0) { loadIrregularVerbsVOs(); } long startTime = System.currentTimeMillis(); PrintUtil.printInfo(CommonUtil.LINE_TWO); loopMatchResult(verbs); long endTime = System.currentTimeMillis(); PrintUtil.printInfo(CommonUtil.SPEND + (endTime - startTime) + CommonUtil.SPEND_END); PrintUtil.printInfo(CommonUtil.LINE_THREE); } /** * Add the blank * @param str * @return */ private static String addBlank(String str) { int blank = CommonUtil.STR_SIZE - str.getBytes().length; for (int i = 0; i < blank; i++) { str += CommonUtil.BLANK; } return str.toString(); } }
/IrregularVerbs/src/com/b510/irregularverbs/utils/PrintUtil.java
/** * */ package com.b510.irregularverbs.utils; /** * @author Hongten * @created 2014-2-27 */ public class PrintUtil { public static void printInfo(String info) { System.out.println(info); } public static void printInfo(StringBuffer info) { System.out.println(info); } public static void printInfo(char info) { System.out.println(info); } }
/IrregularVerbs/src/com/b510/irregularverbs/vo/IrregularVerbsVO.java
/** * */ package com.b510.irregularverbs.vo; /** * @author Hongten * @created Feb 27, 2014 */ public class IrregularVerbsVO { private String prototype; private String postTense; private String postParticiple; private String mean; public IrregularVerbsVO(String prototype, String postTense, String postParticiple, String mean) { super(); this.prototype = prototype; this.postTense = postTense; this.postParticiple = postParticiple; this.mean = mean; } public String getPrototype() { return prototype; } public void setPrototype(String prototype) { this.prototype = prototype; } public String getPostTense() { return postTense; } public void setPostTense(String postTense) { this.postTense = postTense; } public String getPostParticiple() { return postParticiple; } public void setPostParticiple(String postParticiple) { this.postParticiple = postParticiple; } public String getMean() { return mean; } public void setMean(String mean) { this.mean = mean; } }
===============================================
Download Source Code : http://files.cnblogs.com/hongten/IrregularVerbs.zip
===============================================
相关文章推荐
- Java创建对象的方法清单 —— 原来还可以这样创建对象
- 汉字方法名和变量名!---- 原来JAVA还可以这样用啊!
- java中的继承问题,类B继承A,那么构造方法需要这样写吗?这不是重复吗?还有接口与抽象类怎么实现?
- 原来高效学习java的方法是这样的……
- Java多态和实现接口的类的对象赋值给接口引用的方法(推荐)
- Java重载重写与实现方法的规则
- java定时器无法自动注入的问题解析(原来Spring定时器可以这样注入service)
- Java中的类、方法、属性的命名规则
- 登陆注册-java三层架构需要导包/命名规则/敲代码方法
- [改善Java代码]不推荐覆写start方法
- java中equals与hashCode还有tostring方法学习记录
- Java反射的两种使用方法(推荐)
- java中:包、类、字段、方法命名规则
- Java 关于eclipse导入项目发生的问题及解决方法(推荐)
- (Template Method)模板方法模式的Java实现 推荐
- Java小记之方法重写与继承中的构造方法的规则
- 再探Java子类方法重写父类方法遵循“两同两小一大”规则
- 转:java中包、类、方法、属性、常量的命名规则
- 重载Throwable.fillInStackTrace方法已提高Java性能这样的做法对法?
- 原来手机还有这样的用途!(尤其是第三种)