struts2+hibernate3+spring3(ssh2)框架下的web应用
2013-12-26 00:00
190 查看
摘要: 让我们先login吧!
是骡子是马,跑两步就知道了,所以先写个程序跑起来再说。首先建立个空的数据库在MySql里面,名字叫sbpms,然后建立一个表叫User,表有id,用户名,密码,角色,上级,邮箱。所以如下操作。
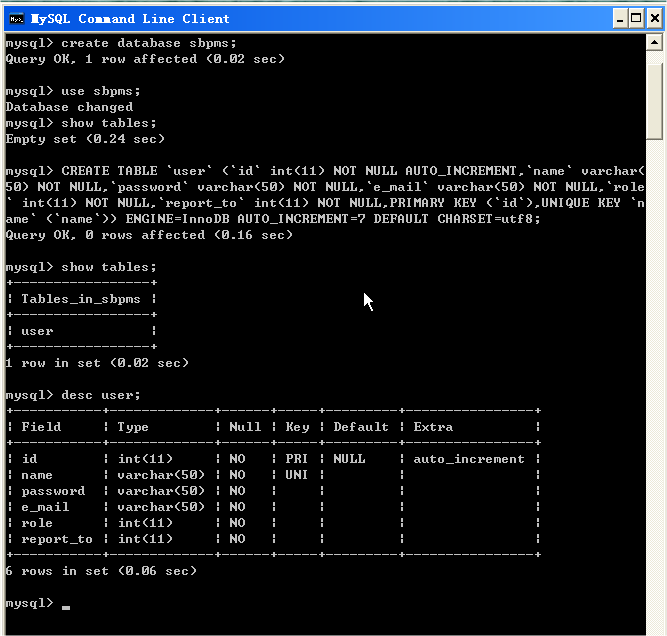
再插入一条数据INSERT INTO `user` VALUES (1,'ROOT',123','root@root.com',0,0);
然后在Eclipse的WebContent下WEB-PAGE根目录下创建两个jsp页面。Login.jsp和Test.jsp
Login.jsp内容为
Test.jsp内容为

然后在src的action中添加
.java
内容为
在com.sbpms.bean中添加User.java和User.hbm.xml文件
User.java为
User.hbm.xml为
接着开始写业务逻辑层
我将dao层和service层都用接口申明,然后在其impl子文件夹下实现。这样使得项目具有更好的扩展性。
下面是com.sbpms.dao下面建立UserDao.java,内容为
com.sbpms.dao.impl下建立UserDaoImpl.java内容为
com.sbpms.service下建立UserService.java,内容为
com.sbpms.service.impl下建立UserServiceImpl.java内容为
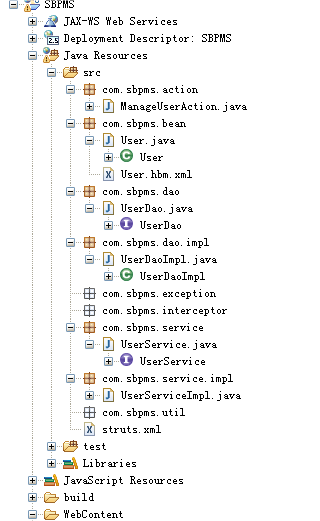
搞定,然后在Login.jsp上面点右键,run on service。得到输入界面,输入刚才插入的用户名root和密码123,得到成功的界面Test。如果输出错误,得到错误提示。
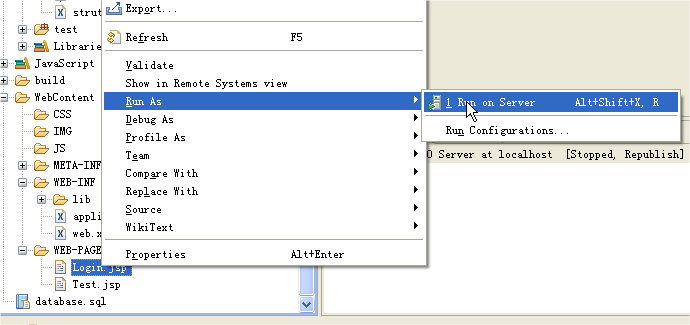
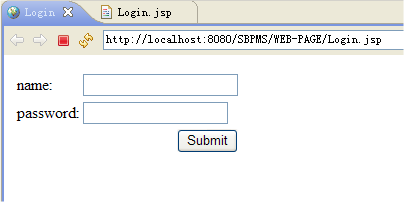
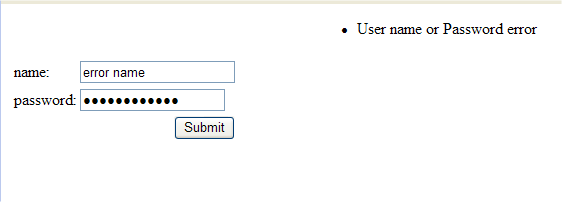
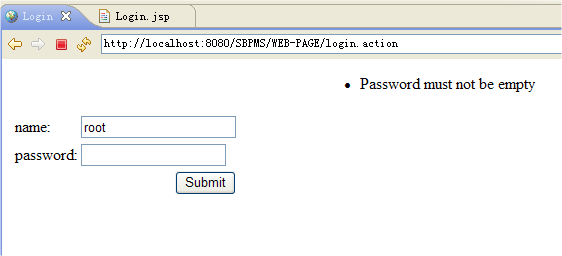
这样,第一个程序就运行起来了,成功的链接了数据库并进行了查询操作和页面错误提示。
完整的源代码我放在爱问上面,地址将在审核通过后给出。
code: http://ishare.iask.sina.com.cn/f/18694480.html
是骡子是马,跑两步就知道了,所以先写个程序跑起来再说。首先建立个空的数据库在MySql里面,名字叫sbpms,然后建立一个表叫User,表有id,用户名,密码,角色,上级,邮箱。所以如下操作。
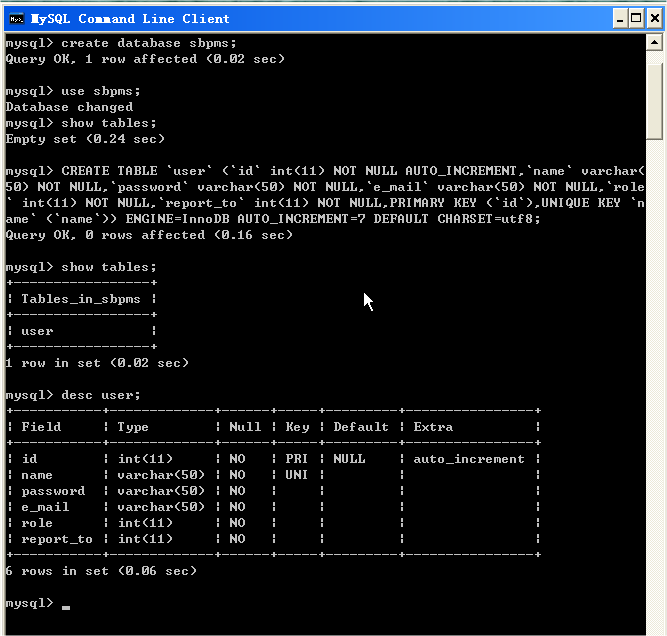
再插入一条数据INSERT INTO `user` VALUES (1,'ROOT',123','root@root.com',0,0);
然后在Eclipse的WebContent下WEB-PAGE根目录下创建两个jsp页面。Login.jsp和Test.jsp
Login.jsp内容为
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <%@ taglib prefix="s" uri="/struts-tags"%> <html> <head> <title>Login</title> </head> <body id="login"> <center> <s:actionerror id="actionError"/> </center> <s:form action="login"> <s:textfield name="user.name" label="name"></s:textfield> <s:password name="user.password" label="password"></s:password> <s:submit></s:submit> </s:form> </body> </html>
Test.jsp内容为
<%@ page language="java" import="java.util.*" pageEncoding="utf-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Test</title> </head> <body> Test </body> </html>

然后在src的action中添加
ManageUserAction
.java
内容为
package com.sbpms.action; import com.opensymphony.xwork2.ActionSupport; import com.sbpms.bean.User; import com.sbpms.service.UserService; /** * This action is used to validate and deal with login processing * @author IanLi * */ @SuppressWarnings("serial") public class ManageUserAction extends ActionSupport { private UserService service; private User user; public UserService getService() { return service; } public void setService(UserService service) { this.service = service; } public User getUser() { return user; } public void setUser(User user) { this.user = user; } /* * This method is used to the login JSP, include the operations of call * database and do validation. */ public String login() throws Exception { switch (this.user.getRole()) { case 0: return SUCCESS; case 1: return SUCCESS; case 2: return SUCCESS; case 3: return SUCCESS; case 4: return SUCCESS; default: return INPUT; } } public void validateLogin() { this.clearActionErrors(); if (null == this.user) { this.addActionError("User name must not be empty"); return; } // Judge the the name input by whether it is empty. if (this.user.getName() == "" || null == this.user.getName()) { this.addActionError("User name must not be empty"); } else { System.out.println(this.user.toString()); // Judge the password input by whether it it empty. if (this.user.getPassword().isEmpty() ||null == this.user.getPassword()) { this.addActionError("Password must not be empty"); } else { // Judge the input name and password by whether they are accord // with the database. this.user = this.service.login(this.user); if (null == this.user) { this.addActionError("User name or Password error"); this.user = null; } else { } } } } }
在com.sbpms.bean中添加User.java和User.hbm.xml文件
User.java为
package com.sbpms.bean; /** *Bean for save the user * * @author IanLi */ public class User { private String pre_password; private Integer id; private String name; private String password; private String e_mail; private int role; private int report_to; public String getPre_password() { return pre_password; } public void setPre_password(String pre_password) { this.pre_password = pre_password; } public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getE_mail() { return e_mail; } public void setE_mail(String e_mail) { this.e_mail = e_mail; } public int getRole() { return role; } public void setRole(int role) { this.role = role; } public int getReport_to() { return report_to; } public void setReport_to(int report_to) { this.report_to = report_to; } @Override public String toString() { return name+" "+password; } }
User.hbm.xml为
<?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping package="com.sbpms.bean"> <!-- <generator class="increment"/> --> <class name="User" table="user"> <id name="id" column="id" type="java.lang.Integer"> <generator class="increment"></generator> </id> <property name="name" column="name" type="java.lang.String"></property> <property name="password" column="password" type="java.lang.String"></property> <property name="e_mail" column="e_mail" type="java.lang.String"></property> <property name="role" column="role" type="java.lang.Integer"></property> <property name="report_to" column="report_to" type="java.lang.Integer"></property> </class> </hibernate-mapping>
接着开始写业务逻辑层
我将dao层和service层都用接口申明,然后在其impl子文件夹下实现。这样使得项目具有更好的扩展性。
下面是com.sbpms.dao下面建立UserDao.java,内容为
package com.sbpms.dao; import com.sbpms.bean.User; /** * Define the interface of User DAO * * @author IanLi */ public interface UserDao { /** * Get the information of the user * * @param user * the user with information of name and password * @return the detail information of the user ligin */ public User selectUser(User user); }
com.sbpms.dao.impl下建立UserDaoImpl.java内容为
package com.sbpms.dao.impl; import java.util.List; import org.springframework.orm.hibernate3.support.HibernateDaoSupport; import com.sbpms.bean.User; import com.sbpms.dao.UserDao; /** * The implements for User DAO interface * * @author IanLi */ public class UserDaoImpl extends HibernateDaoSupport implements UserDao { /* * Search the user from database and judge whether it is correct. * * @see * com.augmentum.ian.ihds.dao.UserDao#searchUser(com.augmentum.ian.ihds. * bean.User) */ @SuppressWarnings("unchecked") @Override public User selectUser(User user) { String sqlString = "from User user where user.name=?"; String name = user.getName(); List<User> userList = this.getHibernateTemplate().find(sqlString, name); if (userList.size() != 0) { // If the database return more than one user by the name, We only // get the first one. In fact this is a wrong database. User userR = userList.get(0); // Judge the name and the password between the input and which get // from database. if (userR.getName().equals(user.getName()) && userR.getPassword().equals(user.getPassword())) { return userR; } return null; } return null; } }
com.sbpms.service下建立UserService.java,内容为
package com.sbpms.service; import com.sbpms.bean.User; /** * Define the interface for the User DAO * * @author IanLi */ public interface UserService { /** * Used to get message of who login * * @param user * the information of the name and password that who login * @return the detail information of user who login */ public User login(User user); }
com.sbpms.service.impl下建立UserServiceImpl.java内容为
package com.sbpms.service.impl; import com.sbpms.bean.User; import com.sbpms.dao.UserDao; import com.sbpms.service.UserService; /** *The implement of user service. Accomplish most business logic here * * @author IanLi */ public class UserServiceImpl implements UserService { private UserDao userDao; private User newUserForService; public UserDao getUserDao() { return userDao; } public void setUserDao(UserDao userDao) { this.userDao = userDao; } public User getNewUserForService() { return newUserForService; } public void setNewUserForService(User newUserForService) { this.newUserForService = newUserForService; } /* * Get message of user who login */ @Override public User login(User user) { return userDao.selectUser(user); } }
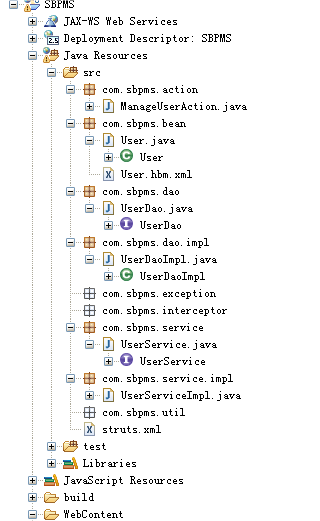
搞定,然后在Login.jsp上面点右键,run on service。得到输入界面,输入刚才插入的用户名root和密码123,得到成功的界面Test。如果输出错误,得到错误提示。
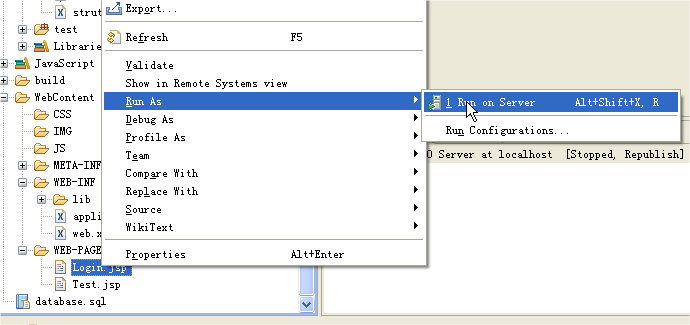
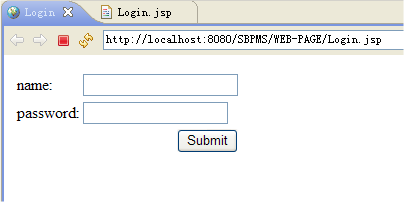
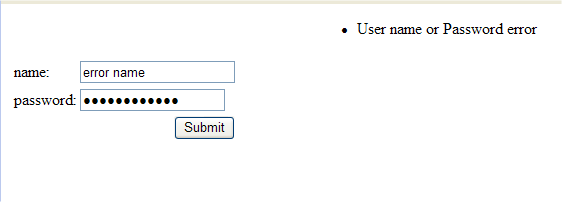
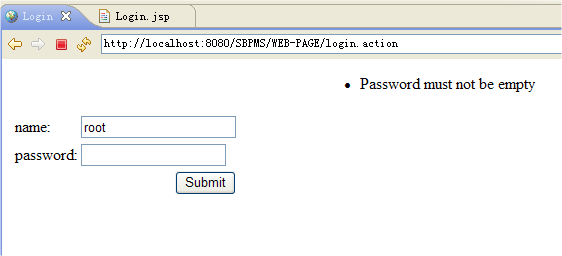
这样,第一个程序就运行起来了,成功的链接了数据库并进行了查询操作和页面错误提示。
完整的源代码我放在爱问上面,地址将在审核通过后给出。
code: http://ishare.iask.sina.com.cn/f/18694480.html
相关文章推荐
- struts2+hibernate3+spring3(ssh2)框架下的web应用
- struts2+hibernate3+spring3(ssh2)框架下的web应用(2)
- struts2+hibernate3+spring3(ssh2)框架下的web应用(1)
- struts2+hibernate3+spring3(ssh2)框架下的web应用-SBPMS系统
- struts2.1+spring2.5+hibernate3框架搭建时出现的错误及解决办法
- Struts2+Hibernate3+Spring三大框架技术实现MySQL数据分页
- 基于SSH(Struts2.0+Spring2.5+Hibernate3)的框架构建(1)
- 基于SSH(Struts2.0+Spring2.5+Hibernate3)的框架构建(2)
- java SSH2 框架搭建 (myeclipse 6.5 + jdk 1.6+ struts-2.3.1.2+hibernate 3.5+spring 3.1)
- Struts + Spring + Hibernate基础框架搭建
- Java开源框架(Struts、Hibernate、Spring)笔试面试大全
- J2EE框架(Struts&Hibernate&Spring)的理解
- 各种框架(Struts、Spring、Hibernate)及应用服务器(Tomcat、WebSphere)对应的开发环境(JDK、Servlet、JSP)
- SSM框架的基本搭建(Spring+Struts+Mybatis)
- J2EE框架(Struts,Hibernate,Spring)
- JEE5标准与Struts/Spring/Hibernate等诸多开源框架的比较分析
- 搭建ssh2(struts2.1.8,spring2.5.6,hibernate3)需要用到的所有jar包的名字
- ssh三大框架——Spring,hibernate,struts面试笔试题汇总(含答案)
- 使用struts+spring+hibernate组装web应用
- 使用struts+spring+hibernate 组装web应用