Spring MVC 3 Controller for MyBatis CRUD operation
by
Rahul Mondalon May 3rd,2012
|
Filed in: Enterprise JavaTags: CRUD,
MyBatis,
Spring,
Spring MVC
Till now we have created CRUD database service for the domain class “
User” and alsointegratedMyBatis configuration with Spring Configuration file. Next,we will create an web page using Spring MVC to use
MyBatis CRUD service to perform operations to database.
Creating
DAO class using MyBatis 3 that will help to perform CRUD operations on database.
Setting Environment for
integrating MyBatis 3 and Spring MVC 3 frameworks.
Creating
Spring MVC 3 controller for performing CRUD operation.
So,in this part we will go through following sessions that will make us able to create the user interface for the example using Spring MVC and MyBatis CRUD service:
The Spring Form Validator
The Spring MVC Controller
The jsp page andJavaScriptfiles used to perform UI creation
And the last Spring MVC configuration file
The Spring Form ValidatorFirst of all we will see the form validator used in this example. Here is the code:
view sourceprint?01 | package com.raistudies.validator; |
03 | import org.springframework.stereotype.Component; |
04 | import org.springframework.validation.Errors; |
05 | import org.springframework.validation.ValidationUtils; |
06 | import org.springframework.validation.Validator; |
08 | import com.raistudies.domain.User; |
11 | public class RegistrationValidator implements
Validator { |
13 | public
boolean supports(Class<?>c) { |
14 | return
User. class .isAssignableFrom(c); |
17 | public
void validate(Object command,Errors errors) { |
18 | ValidationUtils.rejectIfEmptyOrWhitespace(errors, "name" , "field.name.empty" ); |
19 | ValidationUtils.rejectIfEmptyOrWhitespace(errors, "standard" ,
"field.standard.empty" ); |
20 | ValidationUtils.rejectIfEmptyOrWhitespace(errors, "age" , "field.age.empty" ); |
21 | ValidationUtils.rejectIfEmptyOrWhitespace(errors, "sex" , "field.sex.empty" ); |
22 | User usrBean = (User)command; |
23 | if (!isNumber(usrBean.getAge().trim())) |
24 | errors.rejectValue( "age" , "field.age.NAN" ); |
27 | private
boolean isNumber(String str){ |
28 | for
( int i = 0 ;i <str.length();i++) { |
30 | //If we find a non-digit character we return false. |
31 | if
(!Character.isDigit(str.charAt(i))) |
As you can see,we have put some restrictions on the form values like every field should have values and the value in “
age” field should be number. You can add more restrictions on the form values as you need from the
validate() method of this class. The contain of the error message values are defined in the property file
messages.properties, which is as bellow:
view sourceprint?1 | field.name.empty=Name field is mandatory. |
2 | field.standard.empty=Standard field is mandatory. |
3 | field.age.empty=Age field is mandatory. |
4 | field.sex.empty=Sex field is mandatory. |
6 | field.age.NAN=Age should be a number. |
That is all for the form validation part,now we will see controller part.
The Spring MVC ControllerThe controller will project the request from browser to MyBatis services. Bellow is the code:
view sourceprint?01 | package com.raistudies.controllers;
|
06 | import org.springframework.beans.factory.annotation.Autowired;
|
07 | import org.springframework.stereotype.Controller;
|
08 | import org.springframework.ui.ModelMap;
|
09 | import org.springframework.validation.BindingResult;
|
10 | import org.springframework.web.bind.annotation.ModelAttribute;
|
11 | import org.springframework.web.bind.annotation.RequestMapping;
|
12 | import org.springframework.web.bind.annotation.RequestMethod;
|
13 | import org.springframework.web.servlet.ModelAndView;
|
15 | import com.raistudies.domain.User; |
16 | import com.raistudies.persistence.UserService;
|
17 | import com.raistudies.validator.RegistrationValidator;
|
20 | @RequestMapping (value= "/registration" ) |
21 | public class RegistrationController { |
23 | private
RegistrationValidatorvalidator = null ; |
24 | private
UserService userService = null ; |
27 | public
void setUserService(UserService userService) { |
28 | this .userService = userService; |
31 | public
RegistrationValidatorgetValidator() { |
36 | public
void setValidator(RegistrationValidatorvalidator) {
|
37 | this .validator = validator; |
40 | @RequestMapping (method=RequestMethod.GET) |
41 | public
String showForm(ModelMap model){ |
42 | List<User>users = userService.getAllUser(); |
43 | model.addAttribute( "users" ,users); |
44 | User user = new User();
|
45 | user.setId(UUID.randomUUID().toString()); |
46 | model.addAttribute( "user" ,user); |
50 | @RequestMapping (value= "/add" ,method=RequestMethod.POST) |
51 | public
ModelAndView add( @ModelAttribute (value= "user" ) User user,BindingResult result){ |
52 | validator.validate(user,result); |
53 | ModelAndView mv = new ModelAndView( "registration" ); |
54 | if (!result.hasErrors()){ |
55 | userService.saveUser(user); |
57 | user.setId(UUID.randomUUID().toString()); |
58 | mv.addObject( "user" ,user); |
60 | mv.addObject( "users" ,userService.getAllUser()); |
64 | @RequestMapping (value= "/update" ,method=RequestMethod.POST) |
65 | public
ModelAndView update( @ModelAttribute (value= "user" ) User user,BindingResult result){ |
66 | validator.validate(user,result); |
67 | ModelAndView mv = new ModelAndView( "registration" ); |
68 | if (!result.hasErrors()){ |
69 | userService.updateUser(user); |
71 | user.setId(UUID.randomUUID().toString()); |
72 | mv.addObject( "user" ,user); |
74 | mv.addObject( "users" ,userService.getAllUser()); |
78 | @RequestMapping (value= "/delete" ,method=RequestMethod.POST) |
79 | public
ModelAndView delete( @ModelAttribute (value= "user" ) User user,BindingResult result){ |
80 | validator.validate(user,result); |
81 | ModelAndView mv = new ModelAndView( "registration" ); |
82 | if (!result.hasErrors()){ |
83 | userService.deleteUser(user.getId()); |
85 | user.setId(UUID.randomUUID().toString()); |
86 | mv.addObject( "user" ,user); |
88 | mv.addObject( "users" ,userService.getAllUser()); |
The controller is using two beans for the CRUD operations,one is
RegistrationValidator that we say above to validate the form data and other is
UserService
that we created in our previous part using MyBatis 3 to perform database operations on the form data. Both the beans will beauto wiredby spring using setter injection.
The controller have following operation methods that handles CRUD requests on form data:
showForm() :This operations will show up the form for the first time that is why we have put method type as
RequestMethod.GET
The method will also provide all available users in database using
getAllUser() method of
UserService to show up on the table bellow the form.
add() : This operation will handle the create operation. First of all it will validate the form data and if no erroroccurthen it will save form data to database to create a new user using
saveUser()method of
UserService.
It will also bind a new User object with the form.
update() : update method will update the user detail to the database using
updateUser() method of
UserService and before that it will validate the data using
RegistrationValidator.delete() :This method is used to delete the user from database and take helps from the
deleteUser() method of
UserService for this.
The jsp page and JavaScript files used to perform UI creationIn this session,we will see user interface part of the example. We are to create a web page like the following :

|
Spring MVC and MyBatis 3 Integration – User Form |
UI functionality:
The “Save Changes” button will be used to create a new user or to update an existing user.
The “New User” button will be used to set the form to create a new user.
The “Delete User” button will be used to delete a user who’s details are showing in the form
Clicking on any row will bring the corresponding row data to form fields for update or delete.
Let us see jsp code corresponding to the web page:
view sourceprint?04 | <%@ page session="true" %> |
09 | < title >Hello World with Spring 3 MVC</ title > |
10 | < meta
http-equiv = "Content-Type"
content = "text/html;charset=windows-1251" > |
11 | < script
type = "text/javascript"
src='<c:url value = "/resources/common.js" />'></ script > |
12 | < script
type = "text/javascript"
src='<c:url value = "/resources/registration.js" />'></ script > |
13 | < script
type = "text/javascript" > |
14 | var projectUrl = '< c:url
value = "/" />'; |
15 | if(projectUrl.indexOf(";",0) != -1){
|
16 | projectUrl = projectUrl.substring(0,projectUrl.indexOf(";",0)); |
22 | < legend >Registration Form</ legend > |
24 | < form:form
commandName = "user"
action = "/SpringMVCMyBatisCRUDExample/app/registration/add"
name = "userForm" > |
26 | < form:hidden
path = "id" /> |
28 | < tr >< td
colspan = "2"
align = "left" >< form:errors
path = "*"
cssStyle = "color : red;" /></ td ></ tr > |
29 | < tr >< td >Name : </ td >< td >< form:input
path = "name"
/></ td ></ tr > |
30 | < tr >< td >Standard : </ td >< td >< form:input
path = "standard"
/></ td ></ tr > |
31 | < tr >< td >Age : </ td >< td >< form:input
path = "age"
/></ td ></ tr > |
32 | < tr >< td >Sex : </ td >< td >< form:select
path = "sex" > |
33 | < form:option
value = "Male" /> |
34 | < form:option
value = "Female" /> |
35 | </ form:select ></ td ></ tr > |
36 | < tr >< td
colspan = "2" >< input
type = "submit"
value = "Save Changes" /> |
37 | < input
type = "reset"
name = "newUser"
value = "New User"
onclick = "setAddForm();"
disabled = "disabled" /> |
38 | < input
type = "submit"
name = "deleteUser"
value = "Delete User"
onclick = "setDeleteForm();"
disabled = "disabled" /></ td ></ tr > |
43 | < c:if
test = "${!empty users}" > |
48 | < tr
style = "background-color: gray;" > |
54 | < c:forEach
items = "${users}"
var = "user" > |
55 | < tr
style = "background-color: silver;"
id = "${user.id}"
onclick = "setUpdateForm('${user.id}');" > |
56 | < td >< c:out
value = "${user.name}" /></ td > |
57 | < td >< c:out
value = "${user.standard}" /></ td > |
58 | < td >< c:out
value = "${user.age}" /></ td > |
59 | < td >< c:out
value = "${user.sex}" /></ td > |
As you can see,the default action of the form is to add the form detail to database using add method of the controller. On click of “Delete User”,it will call a JavaScript function that will change the form url to delete the user. We have used<c:forEach/>jstl for showing all the users on the table and every row hasdeclaredon click even which will bring the table row data to form and also will change the form submit url to call update() method of controller.
Spring MVC configuration fileAt last we will see the Spring MVC configuration that has been using to configure the controller and all other things. Following is the configuration file :
view sourceprint?03 | < pre ><? xml
version = "1.0"
encoding = "UTF-8" ?> |
13 | <!-- Application Message Bundle --> |
14 | < bean
id = "messageSource"
class = "org.springframework.context.support.ReloadableResourceBundleMessageSource" > |
15 | < property
name = "basename"
value = "/WEB-INF/messages"
/> |
16 | < property
name = "cacheSeconds"
value = "3000"
/> |
19 | <!-- Scans the classpath of this application for @Components to deploy as beans --> |
20 | < context:component-scan
base-package = "com.raistudies"
/> |
22 | <!-- Configures the @Controller programming model --> |
23 | < mvc:annotation-driven
/> |
25 | <!-- Resolves view names to protected .jsp resources within the /WEB-INF/views directory --> |
26 | < bean
id = "viewResolver"
class = "org.springframework.web.servlet.view.InternalResourceViewResolver" > |
27 | < property
name = "prefix"
value = "/WEB-INF/jsp/" /> |
28 | < property
name = "suffix"
value = ".jsp" /> |
31 | < import
resource = "jdbc-context.xml" /> |
The configuration file includes themessages.properties as resource bundle and also includejdbc-context.xml configuration files which contains configuration for integration MyBatis 3 with Spring.
While running the example you will get the above screen as out put that will also show all the records present in database. Click on the “
Save Changes” button you will get following screen that will show you validation errors on the blank
form values:
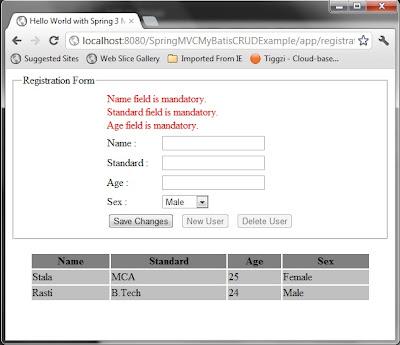
|
Spring MVC and MyBatis 3 Integration – User Form with validation errors |
Now fill the form with valid data and and click on the “Save Changes” button,it will bring the form data to bellow table.
That is all from this example. Hope that you have enjoyed the learning!!

Creating
DAO class using MyBatis 3 that will help to perform CRUD operations on database.
Setting Environment for
integrating MyBatis 3 and Spring MVC 3 frameworks.
Creating
Spring MVC 3 controller for performing CRUD operation.
You can download the source code and war file from following links:
Source (Eclipse Project without jars) :
DownloadWar (With jars) :
DownloadReference: Spring
MVC 3 controller for performing CRUD operation using MyBatis(iBatis) 3 from our
JCG partner Rahul Mondal at the
Rai Studies blog.