java中线程的创建
2013-09-29 19:59
148 查看
一个exe是一个进程,一个exe有一个main函数表示一个主线程,一个线程可以生成多个线程。当一进程要执行,代码必须要调入内存,表示准备执行。
Java里面的线程通过java.lang.thread这个类来实现的,每一个thread对象代表一个新的线程。通过thread类的start方法来启动一个线程。
java中创建新的线程
(1)实现一个类的runnable接口。Runnable只有一个方法run,用以定义线程运行体。
Thread(Runnable target);//构造方法
(2)从thread类继承。
两种方法,最好选用接口,因为继承比较死,从一个类继承就不能从其他类继承了。
直接调用run和调用start后的区别
(1)如果不先调用start直接调用run,这是一个方法的调用,不是多线程。
(2)一个线程你把它new出来的时候,它只是一个对象,接下来你调用Start方法表示线程进入就绪状态,但是还没有分配到CPU。run()方法应该是由操作系统调度了线程,线程进入运行状态,run()方法被自动调用,run方法一结束,线程就结束。
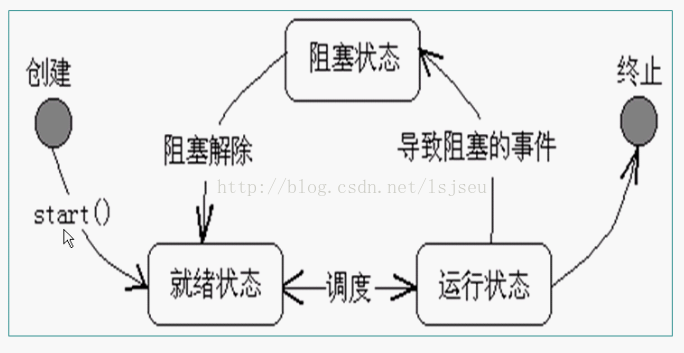
线程控制方法
(1) isAlive();//判断进程是否还活着(终止);
(2) getPriority();//获得线程的优先级数值;
(3) setPriority();//获得线程的优先级数值;
(4) Thread.sleep();//静态方法,将当前线程睡眠,指定毫秒数;睡着的时候被别人打断了就会抛出InterrupttedException
(5) join();//调用线程的该方法,将当前线程与该线程“合并”,即等待该线程结束,再恢复当前线程运行;
(6) yield();//让出cpu,当前线程进入就绪队列等待调度;
(7) wait();//当前线程进入等待池,等待被唤醒;
(8) notify()/notifyAll();//唤醒等待池中的一个/所有线程。
运行结果:
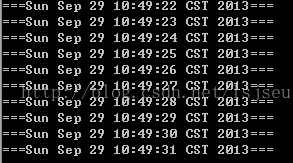
Java里面的线程通过java.lang.thread这个类来实现的,每一个thread对象代表一个新的线程。通过thread类的start方法来启动一个线程。
java中创建新的线程
(1)实现一个类的runnable接口。Runnable只有一个方法run,用以定义线程运行体。
Thread(Runnable target);//构造方法
(2)从thread类继承。
public class testThread { public static void main(String args[]){ Thread t = new Thread(new Runner()); t.start();//t1这个线程进入准备状态 for(int i=0; i<10; ++i){ System.out.println("Main" + ":" + i); } } } class Runner implements Runnable //实现方式1 { public void run(){ for(int i=0; i<10; ++i){ System.out.println("i am runner " + ":" + i); } } } class Mythread extends Thread //实现方式2 { public void run(){ for(int i=0; i<10; ++i){ System.out.println("i am runner " + ":" + i); } } }
两种方法,最好选用接口,因为继承比较死,从一个类继承就不能从其他类继承了。
直接调用run和调用start后的区别
(1)如果不先调用start直接调用run,这是一个方法的调用,不是多线程。
(2)一个线程你把它new出来的时候,它只是一个对象,接下来你调用Start方法表示线程进入就绪状态,但是还没有分配到CPU。run()方法应该是由操作系统调度了线程,线程进入运行状态,run()方法被自动调用,run方法一结束,线程就结束。
线程控制方法
(1) isAlive();//判断进程是否还活着(终止);
(2) getPriority();//获得线程的优先级数值;
(3) setPriority();//获得线程的优先级数值;
(4) Thread.sleep();//静态方法,将当前线程睡眠,指定毫秒数;睡着的时候被别人打断了就会抛出InterrupttedException
(5) join();//调用线程的该方法,将当前线程与该线程“合并”,即等待该线程结束,再恢复当前线程运行;
(6) yield();//让出cpu,当前线程进入就绪队列等待调度;
(7) wait();//当前线程进入等待池,等待被唤醒;
(8) notify()/notifyAll();//唤醒等待池中的一个/所有线程。
import java.util.*; public class testThread { public static void main(String args[]){ Mythread t = new Mythread(); t.start(); try { Thread.sleep(10000);//让主线程睡着 } catch (InterruptedException e) { } t.interrupt();//睡着的时候去打断t,这时候t抛出异常 } } class Mythread extends Thread { public void run(){ while(true){ System.out.println("===" + new Date() + "==="); try{ sleep(1000);//调用sleep的时候要去捕获异常 }catch(InterruptedException e){ return;//抛出异常我们直接让它return } } } }
运行结果:
//如何正常让一个线程停止: import java.util.*; public class testThread { public static void main(String args[]){ Mythread t = new Mythread(); t.start(); try { Thread.sleep(100);//让主线程睡着 } catch (InterruptedException e) { } t.shutDown();//睡着的时候去打断t,这时候t抛出异常 } } class Mythread extends Thread { private boolean flag = true;//设置一个标记 public void run(){ while(flag){ System.out.println("===" + new Date() + "==="); } } public void shutDown(){flag = false;} }
相关文章推荐
- Java多线程--创建线程
- Java线程学习03/【线程创建的俩种方法】/byme
- 使用Callable接口创建线程(Java5新增)
- java中创建线程主要的三种方式
- java核心知识点学习----并发和并行的区别,进程和线程的区别,如何创建线程和线程的四种状态,什么是线程计时器
- java创建线程的三种方式及其对比
- Java-线程-创建线程的方法
- Java--线程的创建方式(1)
- Java创建线程的两个方法
- 【如何创建并运行java线程】
- java线程创建的两种方式
- java多线程(二)——第二种线程创建方式
- Java创建线程的两个方法
- Java线程创建详解
- 创建Java线程的3种方式及对比
- Java创建线程的两个方法
- Java——创建线程的两种方式
- Java通过实现Runnable接口来创建线程
- Java中创建线程的两个方法____解决火车票或售票问题
- JAVA 并发编程随笔【五】Thread线程创建及运行线程任务