[IOS地图开发系类]5、改变大头针MKPinAnnotationView的颜色
2013-07-28 22:58
746 查看
在第四步的基础上,我们试着改变下大头针的颜色
从大头针的头文件中可以看到,它有一个颜色属性我们可以直接使用。
修改下CustomAnnotation.h和其实现文件,代码如下:
// // MKPinAnnotationView.h // MapKit // // Copyright (c) 2009-2012, Apple Inc. All rights reserved. // #import <MapKit/MKAnnotationView.h> enum { MKPinAnnotationColorRed = 0, MKPinAnnotationColorGreen, MKPinAnnotationColorPurple }; typedef NSUInteger MKPinAnnotationColor; @class MKPinAnnotationViewInternal; MK_CLASS_AVAILABLE(NA, 3_0) @interface MKPinAnnotationView : MKAnnotationView { @private MKPinAnnotationViewInternal *_pinInternal; } @property (nonatomic) MKPinAnnotationColor pinColor; @property (nonatomic) BOOL animatesDrop; @end
从大头针的头文件中可以看到,它有一个颜色属性我们可以直接使用。
修改下CustomAnnotation.h和其实现文件,代码如下:
// // CustomAnnotation.h // LBS_002_mapview // // Created by liqun on 13-7-25. // Copyright (c) 2013年 Block Cheng. All rights reserved. // #import <Foundation/Foundation.h> #import <MapKit/MapKit.h> #define PIN_RED @"Red" #define PIN_GREEN @"Green" #define PIN_PURPLE @"Purple" @interface CustomAnnotation : NSObject<MKAnnotation> @property (nonatomic, assign, readonly) CLLocationCoordinate2D coordinate; @property (nonatomic, copy, readonly) NSString *title; @property (nonatomic, copy, readonly) NSString *subtitle; @property (nonatomic, unsafe_unretained) MKPinAnnotationColor pinColor; - (id) initWithCoordinates:(CLLocationCoordinate2D)paramCoordinates title:(NSString *)paramTitle subTitle:(NSString *)paramSubTitle; + (NSString *) reusableIdentifierforPinColor :(MKPinAnnotationColor)paramColor; @end
// // CustomAnnotation.m // LBS_002_mapview // // Created by liqun on 13-7-25. // Copyright (c) 2013年 Block Cheng. All rights reserved. // #import "CustomAnnotation.h" @implementation CustomAnnotation - (void)dealloc { [_subtitle release]; [_title release]; [super dealloc]; } - (id) initWithCoordinates:(CLLocationCoordinate2D)paramCoordinates title:(NSString *)paramTitle subTitle:(NSString *)paramSubTitle { if (self = [super init]) { _coordinate = paramCoordinates; _title = [paramTitle copy]; _subtitle = [paramSubTitle copy]; } return self; } + (NSString *) reusableIdentifierforPinColor :(MKPinAnnotationColor)paramColor{ NSString *result = nil; switch (paramColor){ case MKPinAnnotationColorRed:{ result = PIN_RED; break; } case MKPinAnnotationColorGreen:{ result = PIN_GREEN; break; } case MKPinAnnotationColorPurple:{ result = PIN_PURPLE; break; } } return result; } @end最后,在ViewControlelr实现文件中,加上该注解类的颜色属性:
// // ViewController.m // LBS_002_mapview // // Created by liqun on 13-7-25. // Copyright (c) 2013年 Block Cheng. All rights reserved. // #import "ViewController.h" #import <MapKit/MapKit.h> #import "CustomAnnotation.h" @interface ViewController ()<CLLocationManagerDelegate,MKMapViewDelegate> @property (nonatomic,retain)MKMapView* mapView; @property (nonatomic,retain)CLLocationManager* locationManager; @property (nonatomic,retain)CLLocation* location; @property (nonatomic, retain) CLGeocoder *myGeocoder; @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; // Do any additional setup after loading the view, typically from a nib. MKMapView* map = [[MKMapView alloc] initWithFrame:self.view.frame]; map.mapType = MKMapTypeStandard; map.autoresizingMask = UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleHeight; [self.view addSubview:map]; [map release]; self.mapView = map; self.mapView.delegate = self; UIButton *addBt = [UIButton buttonWithType:UIButtonTypeRoundedRect]; addBt.frame = CGRectMake(0, 00, 320, 50); [addBt setTitle:@"locationMe" forState:UIControlStateNormal]; [ addBt addTarget:self action:@selector(handleLocationMe:) forControlEvents:UIControlEventTouchUpInside]; [self.view addSubview: addBt]; if ([CLLocationManager locationServicesEnabled]){ self.locationManager = [[CLLocationManager alloc] init]; self.locationManager.delegate = self; self.locationManager.purpose = @"To provide functionality based on user's current location."; [self.locationManager startUpdatingLocation]; } else { /* Location services are not enabled. Take appropriate action: for instance, prompt the user to enable location services */ NSLog(@"Location services are not enabled"); } self.myGeocoder = [[CLGeocoder alloc] init]; } - (MKAnnotationView *)mapView:(MKMapView *)mapView viewForAnnotation:(id <MKAnnotation>)annotation { MKAnnotationView *result = nil; if ([annotation isKindOfClass:[CustomAnnotation class]] == NO){ return result; } if ([mapView isEqual:self.mapView] == NO){ /* We want to process this event only for the Map View that we have created previously */ return result; } /* First, typecast the annotation for which the Map View has fired this delegate message */ CustomAnnotation *senderAnnotation = (CustomAnnotation *)annotation; /* Using the class method we have defined in our custom annotation class, we will attempt to get a reusable identifier for the pin we are about to create */ NSString *pinReusableIdentifier = [CustomAnnotation reusableIdentifierforPinColor:senderAnnotation.pinColor]; /* Using the identifier we retrieved above, we will attempt to reuse a pin in the sender Map View */ MKPinAnnotationView *annotationView = (MKPinAnnotationView *) [mapView dequeueReusableAnnotationViewWithIdentifier:pinReusableIdentifier]; if (annotationView == nil){ /* If we fail to reuse a pin, then we will create one */ annotationView = [[MKPinAnnotationView alloc] initWithAnnotation:senderAnnotation reuseIdentifier:pinReusableIdentifier]; /* Make sure we can see the callouts on top of each pin in case we have assigned title and/or subtitle to each pin */ [annotationView setCanShowCallout:YES]; annotationView.draggable = YES; annotationView.enabled = YES; } /* Now make sure, whether we have reused a pin or created a new one, that the color of the pin matches the color of the annotation */ annotationView.pinColor = senderAnnotation.pinColor; //自定义图片时,不能用drop annotationView.animatesDrop = YES; result = annotationView; return result; } - (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. } -(IBAction)handleLocationMe:(id)sender { [self.myGeocoder reverseGeocodeLocation:self.location completionHandler:^(NSArray *placemarks, NSError *error) { if (error == nil &&[placemarks count] > 0){ CLPlacemark *placemark = [placemarks objectAtIndex:0]; /* We received the results */ NSLog(@"Country = %@", placemark.country); NSLog(@"Postal Code = %@", placemark.postalCode); NSLog(@"Locality = %@", placemark.locality); NSLog(@"dic = %@", placemark.addressDictionary ); NSLog(@"dic FormattedAddressLines= %@", [placemark.addressDictionary objectForKey:@"FormattedAddressLines"]); NSLog(@"dic Name = %@", [placemark.addressDictionary objectForKey:@"Name"]); NSLog(@"dic State = %@", [placemark.addressDictionary objectForKey:@"State"]); NSLog(@"dic Street = %@", [placemark.addressDictionary objectForKey:@"Street"]); NSLog(@"dic SubLocality= %@", [placemark.addressDictionary objectForKey:@"SubLocality"]); NSLog(@"dic SubThoroughfare= %@", [placemark.addressDictionary objectForKey:@"SubThoroughfare"]); NSLog(@"dic Thoroughfare = %@", [placemark.addressDictionary objectForKey:@"Thoroughfare"]); CLLocationCoordinate2D location = CLLocationCoordinate2DMake(self.location.coordinate.latitude, self.location.coordinate.longitude); /* Create the annotation using the location */ CustomAnnotation *annotation =[[CustomAnnotation alloc] initWithCoordinates:location title:placemark.name subTitle:placemark.thoroughfare]; /* And eventually add it to the map */ annotation.pinColor = MKPinAnnotationColorPurple; [self.mapView addAnnotation:annotation]; [annotation release]; } else if (error == nil && [placemarks count] == 0){ NSLog(@"No results were returned."); } else if (error != nil){ NSLog(@"An error occurred = %@", error); } }]; } - (void)locationManager:(CLLocationManager *)manager didUpdateToLocation:(CLLocation *)newLocation fromLocation:(CLLocation *)oldLocation{ /* We received the new location */ NSLog(@"Latitude = %f", newLocation.coordinate.latitude); NSLog(@"Longitude = %f", newLocation.coordinate.longitude); self.location = newLocation; } - (void)locationManager:(CLLocationManager *)manager didFailWithError:(NSError *)error{ /* Failed to receive user's location */ } - (void)dealloc { self.mapView = nil; self.location = nil; self.locationManager = nil; [super dealloc]; } @end运行后,效果如下:
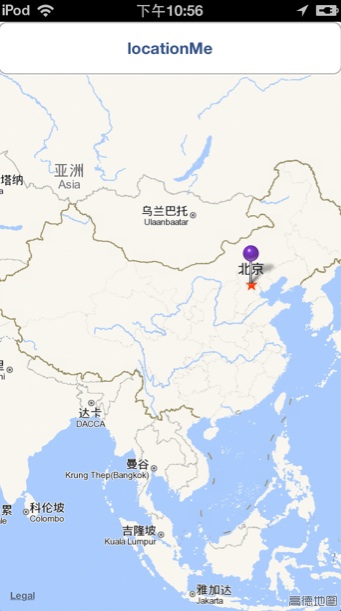
相关文章推荐
- iOS开发 UISearchController的cancel按钮自定义中文取消,改变按钮标题颜色,去掉边框线
- ios开发改变状态栏颜色
- iOS开发用Tableview实现能改变字体颜色的高仿系统的ActionSheet
- iOS开发笔记--使用blend改变图片颜色
- ios开发之--字符串局部改变颜色
- iOS开发笔记--使用blend改变图片颜色
- iOS开发之--改变系统导航的颜色,字体,还有返回样式的自定义
- iOS开发 - 改变picker选中行字体颜色
- iOS开发-改变图片的颜色
- iOS开发绘制三角形和添加文字改变文字大小颜色
- iOS 开发UidatePicker 改变字体颜色的方法
- iOS开发之全面讲解的改变系统顶部状态栏的颜色变化
- iOS开发之--最简单的导航按钮更换方法/导航颜色的改变
- iOS开发中,在label中改变数字颜色的方法(改变某个关键词的方法)
- iOS 开发之改变UITabbar顶部分割线颜色
- iOS开发——改变UITextField的样式:占位符颜色、字符起始位置等
- iOS开发笔记--使用blend改变图片颜色
- iOS开发笔记--使用blend改变图片颜色
- iOS开发笔记--使用blend改变图片颜色
- iOS 开发 解决UITableViewcell单选动态改变cell文字和背景颜色的功能,且第一次默认选择第一个cell