Know the Core Objects of Your App---了解应用程序的内核对象
2013-07-02 18:30
369 查看
Back to App Design
You develop apps using the Cocoa application environment. Cocoa presents the app’s user interface and integrates it tightly with the other components of the operating system. It provides an integrated suite of object-oriented software components packaged in two core class libraries, the AppKit and Foundation frameworks, and a number of other frameworks providing supporting technologies. Cocoa classes are reusable and extensible—you can use them as is or extend them for your particular requirements.
你用Cocoa 应用程序开发环境开发应用程序。 Cocoa 呈现应用程序的用户界面并把它跟操作系统里的其它组件紧密结合。它提供了一整套集成的面向对象软件组件,并把它们打包进2个内核类库AppKit 和 Foundation 框架中,它还提供了一些其它提供支撑技术的各种框架。Cocoa 类都是可重用和可扩展的---你可以直接使用它们或者扩展它们以符合你的特殊需求。
Cocoa makes it easy to create apps that adopt all of the conventions and expose all of the power of OS X. In fact, you can create a new Cocoa application project in Xcode and without adding any code have a functional app. Such an app is able to display its window (or create new documents) and implements many standard system behaviors. And although the Xcode templates provide some code to make this all happen, the amount of code they provide is minimal. Most of the behavior is provided by Cocoa. The code you write for your app defines its particular appearance and behavior and differentiates it from other apps.
Cocoa 让创建应用程序变得容易, 它采用OS X的所有约定,并显露了OS X的所有力量。 事实上, 你可以在Xcode里创建一个新Cocoa 应用程序却不需要添加任何代码,它已经是有一个功能的应用程序。这样的应用程序可以显示它的窗口(或创建新文档), 并实现一些标砖系统行为。 尽管Xcode模板提供了一些代码让所有这些发生, 但是他们提供的是最少量的代码。 大多数行为是由Cocoa提供的。 你编写的代码定义了它的特殊外形和行为,使它区别于别的应用程序。
To make a great app, you should build on the foundations Cocoa lays down for you, working with the conventions and infrastructure provided for you. To do so effectively, it's important to understand how a Cocoa app fits together.
为了完成一个伟大的应用程序,你应该在基础Cocoa层的基础上建立,遵循为你提供的各种约定和基础设施。 为了有效率的完成这些, 理解如何让一个Cocoa 应用程序组合在一起很重要。
The App Style Determines the Core Architecture
一、应用程序风格决定了内核架构The style of your app defines which core objects you must use in its implementation. Cocoa supports the creation of both single-window and multiwindow apps. For multiwindow designs, it also provides a document architecture to help manage the files associated with each app window. Thus, apps can have the following forms (example apps are in parentheses):
应用程序的风格定义了你必须在实现时使用的内核对象。 Cocoa 同时支持单窗口和多窗口应用程序的创建。 对于多窗口设计, 它还提供了一个文档架构来帮助管理每个应用窗口相关联的各种文件。 因此, 应用程序包含以下形式(应用程序事例在括号内):
Single-window utility app (Calculator)
单窗口实用应用(计算器)
These types of apps typically handle ephemeral data or manage system processes and do not create or deal with any documents or persistent user data.
该类型的应用程序通常只处理瞬间的数据或管理系统进程,并不会创建或处理任何文档或长久的用户数据。
Single-window library-style app (iPhoto)
单窗口库类型应用程序(iPhoto)
These types of apps have a single window but do handle persistent user data. Sometimes single-window library-style apps are called shoebox apps. All user interaction with the app happens in a single window. Often these types of apps store user data but do not present files to users, who have no need to manually save, open, or close documents.
Library-style apps typically do not use
NSDocumentobjects to manage their data model. These objects are designed for multiwindow document-based apps.
该类型的应用程序虽然只有一个窗口,但是它们确实要处理永久用户数据。 有时单窗口库类型应用程序被称为鞋盒应用程序。所有跟应用程序有关的用户交互都发生在这个单一窗口。 常常是这些类型的应用程序存储用户数据但是却不给用户显示文件。
库类型应用程序通常不使用NSDocuments对象来管理它们的数据模型。 这些对象都是为多窗口基于文档的应用程序设计的。
Multiwindow document-based app (TextEdit)
多窗口基于文档的应用程序(文档编辑)
These types of apps enable users to create and edit multiple documents. Each document is displayed in its own window and each document is saved to a user-specified location in the file system.
该类型的应用程序允许用户创建和编辑多个文档。 每个文档都显示在它们自己的窗口并且每个文档都存储在文件系统中用户指定的位置。
You should choose a basic app style early in your design process because that choice affects everything you do later. The single-window styles are preferred in many cases, especially for developers bringing apps from iOS. The single-window style typically yields a more streamlined user experience, and it also makes it easier for your app to support a full-screen mode. However, if your app works extensively with complex documents, the multiwindow style may be preferable because it provides more document-related infrastructure to help you implement your app.
你应该在设计进程前选择一个基本的应用类型,因为该选择影响了你以后要做的全部。 单一窗口类型在很多情况下很有优势, 特别是开发者从iOS应用程序转换时。 单窗口类型通常有一个更简洁的用户体验,它还让应用程序支持全屏模式变得更简单。 然而,如果你的应用程序跟各种复杂的文档广泛工作,多窗口类型会更好,因为它提供了更多文档相关的基础设施来帮你实现你的应用程序。
Both single-window and multiwindow apps can present an effective full-screen mode, which provides an immersive experience that enables users to focus on their tasks without distractions.
单窗口和多窗口应用程序都可以实现一个有效的全屏模式, 全屏模式提供了一个不受干扰的用户体验,它能让用户专注于他们的任务而不受干扰。
The Core Objects for All Cocoa Apps
二、所有Cocoa应用程序的内核对象Regardless of whether you are using a single-window or multiwindow app style, all apps use the same core set of objects. Cocoa provides the default behavior for most of these objects. You are expected to provide a certain amount of customization of these objects to implement your app’s custom behavior.
不管你是使用单窗口风格还是多窗口风格, 所有的应用程序都使用相同的内核对象集。 Cocoa 为大多数这些对象提供了默认行为。 你需要对这些对象提供一定数量的定制才能实现你的应用程序的自定义行为。
Using the Model-View-Controller design pattern as a template, Figure 1 shows the relationships among the core objects for the single-window app styles. The objects in this figure are separated according to whether they are part of the model, view, or controller portions of the app. As you can see from the figure, the Cocoa–provided objects constitute much of the controller and view layer for your app.
作为一个模板,使用M-V-C设计模式,图 1 显示了单窗口应用风格的内核对象之间的关系。 图中的对象根据他们是否是模型,视图,控制器的一部分来划分。正如你在图中看到, Cocoa提供的对象组成了应用程序相当一部分的控制器和视图层。
Figure 1 Key objects in a single-window app
图 1 单窗口应用中的关键对象
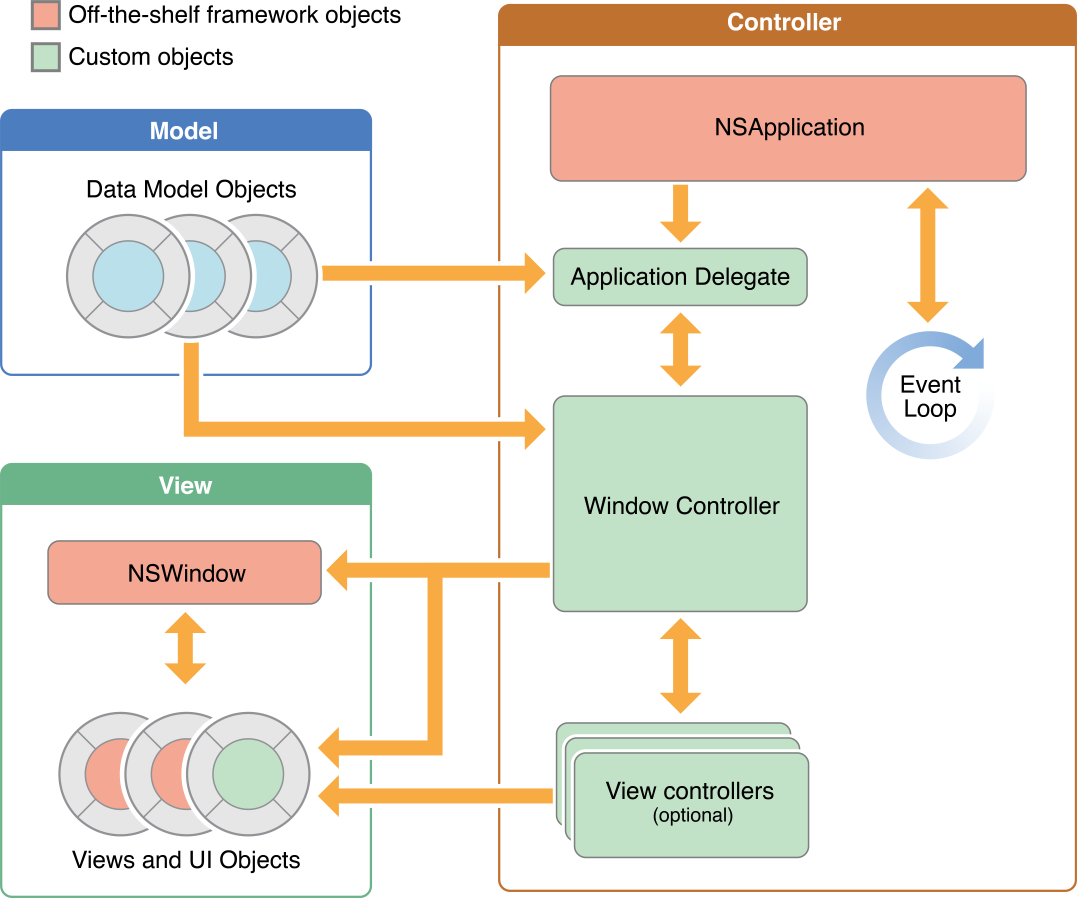
Table 1 describes the roles played by the objects in the diagram.
表一描述了在图中各对象所扮演的角色。
Object | Description(描述) |
---|---|
NSApplicationobject NSApplication对象 | (Required) Runs the event loop and manages interactions between your app and the system. You typically use the NSApplicationclass as is, putting any custom app-object-related code in your application delegate object. (必需) 运行事件循环以及管理应用程序和系统之间的交互。 你通常使用的NSApplication类把任何自定义的应用对象相关的代码放在应用程序代理对象(application delegate object)中。 |
Application delegate object 应用程序代理对象 | (Expected) A custom object that you provide which works closely with the NSApplicationobject to run the app and manage the transitions between different application states. Your application delegate object must conform to the NSApplicationDelegateprotocol. (要求) 你提供的一个自定义对象,它跟NSApplication对象紧密工作,来运行应用程序并管理不同应用状态之间的通信。 应用程序代理对象必需遵循NSApplicationDelegate 协议。 |
Data model objects 数据模型对象 | Hold and manipulate content specific to your app. A banking app might store a database containing financial transactions, whereas a painting app might store an image object or the sequence of drawing commands that led to the creation of that image. 保存并操作应用程序制定的内容。 银行应用程序可能存储一个包含资金交易的数据库,而一个画图应用程序可能存储一个图片对象或完成那个图片创建的绘图命令序列。 |
Window controllers 窗口控制器 | Load and manage a single window with its views and coordinate with the system to handle standard window behaviors. You subclass NSWindowControllerto manage both the window and its contents. 载入并管理单窗口及它的视图, 跟系统协调处理标准窗口行为。 你的子类NSWindowController用来管理窗口以及它的内容。 |
Window objects 窗口对象 | Represent your onscreen windows, configured in different styles depending on your app’s needs. Most windows have title bars and borders. A window object is almost always managed by a window controller. An app can also have secondary windows, also known as dialogs and panels, that support the current document window or the main window. A window is an instance of the NSWindowclass. A panel is an instance of the NSPanelclass (which is a descendant of NSWindow). 代表屏幕上的窗口,根据应用程序的需要设置不同的风格。大多数窗口都有标题栏和边框。 窗口对象大多数总是由窗口控制器管理。 应用程序也可以有副窗口, 被称为对话框(dialogs)和面板(panels), 它们支持当前的文档窗口或主窗口。 |
View controllers 视图控制器 | Coordinate the loading of a single view hierarchy into your app. View controllers work together with the window controller to present the contents of a window. In OS X (unlike in iOS), view controllers are assistants to the window controller, which is ultimately responsible for everything that goes in the window. 向你的应用程序协调载入单窗口视图层次。 视图控制器跟窗口控制器一个工作来显示窗口的内容。 在OS X(不像iOS), 视图控制器是窗口控制器的助手, 窗口控制器对在窗口中运行的所有最终负责。 |
View objects 视图对象 | Define a rectangular region in a window, draw the contents of that region, handle events in that region, and lay out and manage subviews. You can layer views on top of each other to create view hierarchies. 在窗口里定义一个矩形区域, 绘制该区域的内容, 处理该区域内的事件, 布局并管理子视图。 你可以在一个接着一个视图上创建视图,形成视图层次。 |
Control objects 控制对象 | Represent standard system controls such as buttons, text fields, and tables. Most controls work with your code to manage user interactions with your app’s content. 代表标准系统控制,比如按钮,文本框, 表格等。 大多数跟代码一个工作的控制管理跟应用程序内容的各种用户交互。 |
你可以在Mac App Programming Guide 里学习更多关于Cocoa应用程序的文档架构的内容。
Additional Core Objects for Multiwindow Apps
1、多窗口应用程序的附加内核对象Unlike a single-window app, a multiwindow app uses several windows to present its primary content. The Cocoa support for multiwindow apps is built around a document-based model implemented by a subsystem called the document architecture. In this model, each document object manages its content, coordinates the reading and writing of that content from disk, and presents the content in a window for editing. All document objects work with Cocoa to coordinate event delivery, but each document object is otherwise independent of its fellow document objects.
不像单窗口应用,多窗口应用程序使用几个窗口来呈现它的主要内容。 Cocoa支持的多窗口应用程序是建立在由子系统(被称为文档架构)实现的基于文档的模型基础上的。 在该模型中, 每个文档对象管理着它自己的内容, 从硬盘协调读写这些内容, 并在窗口里呈现这些内容以便编辑。所有文档对象跟Cocoa一起工作来协调事件交付,除此之外每个文档对象都跟它的兄弟文档对象相独立。
Figure 2 shows the relationships among the core objects of a multiwindow document-based app. Many of the same objects in this figure are identical to those used by a single-window app. The main difference is the insertion of the
NSDocumentControllerand
NSDocumentobjects between the application objects and the objects for managing the user interface.
图 2 显示了一个多窗口基于文档应用程序的内核对象之间的关系。 图中的许多对象跟单窗口应用程序中采用的相同。 主要区别是在应用程序对象 和 用来管理用户界面的对象 之间 插入的 NSDocumentController 和 NSDocument对像。
Figure 2 Key objects in a multiwindow document app
图 2 一个多窗口应用程序中的主要对象
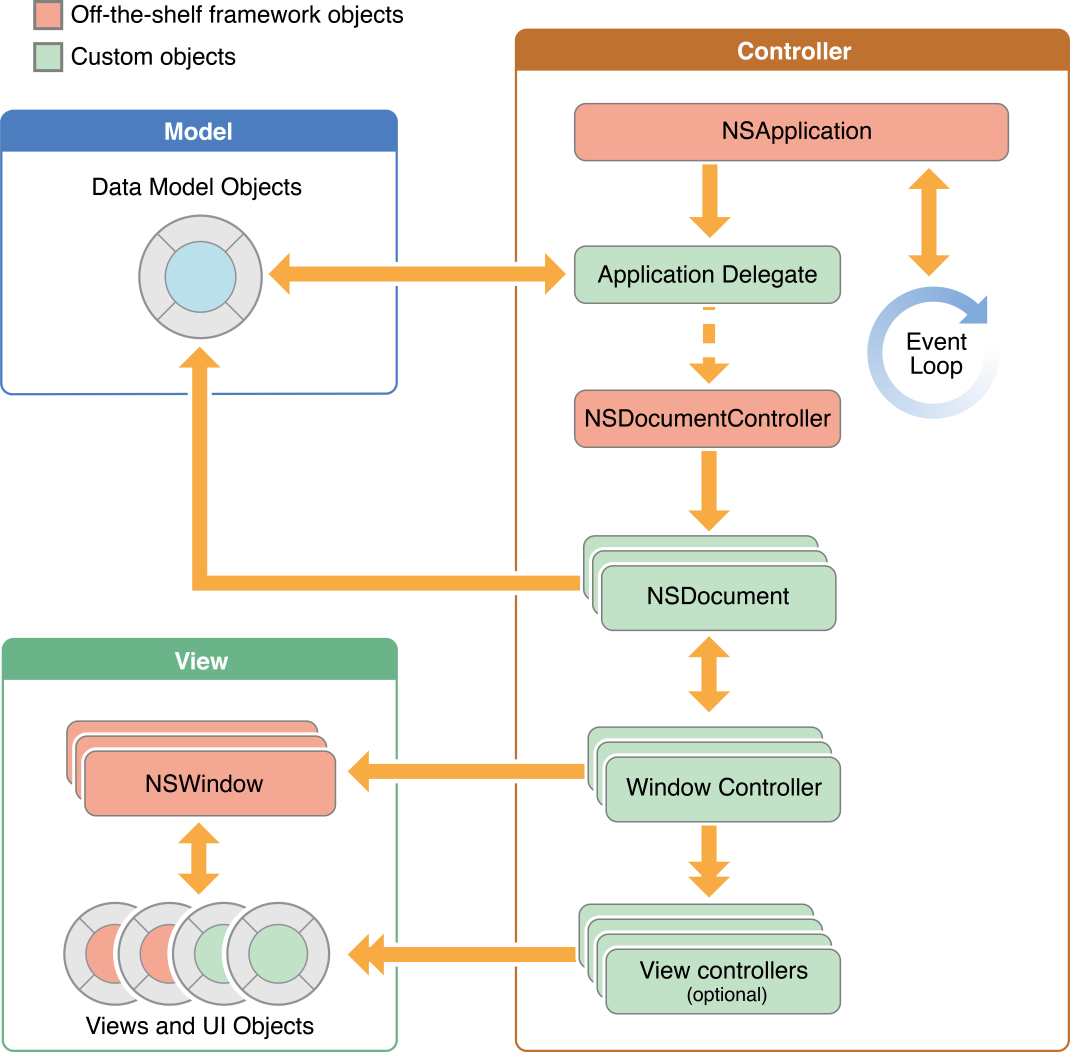
Table 2 describes the role of these inserted objects. (For information about the roles of the other objects in the diagram, see Table 1.)
表 2 描述了这些插入对象的角色(图中其它对象的角色信息,请看表一)。
Table 2 Additional objects used by multiwindow document apps表 2 多窗口文档应用程序使用的附加对象
Object | Description(描述) |
---|---|
Document controller object 文档控制器对象 | Creates and manages all document objects of an app. A document controller also manages many document-related menu items, such as the Open Recent menu and the open and save commands. It is an object of the NSDocumentControllerclass. 创建并管理应用程序中所有的文档对象。 文档对象同时管理多个文档相关的菜单项, 比如打开最近的菜单(open recent menu)以及打开并保存(open and save)命令。 它是NSDocumentController类的一个对象。 |
Document object 文档对象 | Manages the data objects associated with a document and works with other objects to display the document contents in a window. A document object is an instance of a custom subclass of NSDocument. 管理跟文档有关的数据对象, 并跟别的对象一起在窗口中显示文档内容。 文档对象是一个自定义NSDocumeng子类的一个实例。 |
The App Life Cycle and App Termination
三、应用程序生命周期以及应用程序终止The app life cycle is the progress of an app from its launch through its termination. Users or the operating system can launch apps. The user launches apps by double-clicking the app icon, by using Launchpad, or by opening a file whose type is currently associated with the app. The system often launches apps as part of restoring the desktop to its previous state when a user logs in.
应用程序的生命周期是一个应用程序从它启动到它终止的过程。用户或操作系统可以启动应用程序。 用户通过双击应用程序图标来启动应用,它通过使用启动面板,或通过打开一个文件,该文件类型正跟应用程序相关联。 当用户登录时,系统常常把启动应用程序作为重置桌面到前一个状态的一部分。
When an app is launched, the system creates a process and all of the normal system-related data structures for it. Inside the process, it creates a main thread, sets up the main event loop, and uses it to begin executing your app’s code. As described in Integrate Your Code with the Frameworks, an app draws its initial user interface and starts handling user events, one after another, and updating the user interface in the process.
当一个应用程序被启动时, 系统为应用程序创建一个进程以及所有跟系统相关的正常数据结构。在进程内,它创建了一个主线程, 建立了主事件循环,并使用它开始执行应用程序代码。 正如在Integrate Your Code with the Frameworks 里所描述,应用程序绘制它的初始化用户接口,并开始处理用户事件, 一个接着一个,然后在进程里更新用户接口。
Note: The main entry point for an OS X app at launch time is the
mainfunction. Mac App Programming Guidedescribes in detail what happens in this function when an app is launched.
注意: 一个OS X 应用程序在启动时的主入口点(main entry point)是 main 函数。Mac App Programming Guide 详细描述了当应用程序被启动时在该函数里发生了什么。
Users can terminate an app by choosing the Quit command from the application menu, but the app and the system can also work together to manage termination of apps without a user’s involvement. Cocoa supports two techniques that make the termination of an app transparent and fast:
用户可以通过选择应用程序菜单里的退出命令来终止应用程序, 但是应用程序和系统也可以一起来管理应用程序的终止,而不用牵涉用户。Cocoa 支持2种让一个程序明确并快速终止的技术:
Automatic termination eliminates the need for users to quit an app. Instead, the system manages app termination transparently behind the scenes, killing the underlying process for apps that are not in use to reclaim needed resources such as memory.
自动终止不需要用户退出应用程序。相反,系统在屏幕后明确地管理应用程序终止,杀死已经不再使用应用程序的后台进程来回收所需的资源,比如内存 。
Sudden termination allows the system to kill an app’s process immediately without waiting for it to perform any final actions. The system uses this technique to improve the speed of operations such as logging out of, restarting, or shutting down the computer.
突然终止允许系统立刻杀死一个应用程序进程,不需要等待它执行任何最终动作。 系统系统该技术来改进操作系统的速度,比如退出登录,重启,或关闭电脑。
Although automatic termination and sudden termination are independent techniques, apps should generally support both. Apps must opt in to both automatic termination and sudden termination and implement appropriate support for them. They must support persistence and ensure that any user data and state is saved well before termination; if the underlying app process is then killed, the app can use the saved data and state to restore itself to where it was when it was terminated. And because the user does not quit an autoterminable app, such an app should also save the state of its user interface using the built-in Cocoa support. Saving and restoring the interface state provides the user with a sense of continuity between app launches.
尽管自动终止和突然终止是独立技术,应用程序通常应该都支持。 应用程序必须同时选择自动终止和突然终止并实现对它们的适当支持。 它们必须持续存在,并确保任何用户数据和状态都在终止前被完好保存;然后如果后台应用程序被杀死,当下次启动时,应用程序就能使用被保存的数据和状态来恢复到之前的状态。而且因为用户不会退出一个自动终止应用程序,所以这样的应用程序应该也通过使用内置Cocoa支持来保存它的用户界面的状态。保存和恢复接口状态在应用程序启动期间给用户一种连贯性的感觉。
OS X Apps Behave in Similar Ways
四、OS X 应用程序行为的相似性During an app’s runtime life, it behaves in ways that are similar if not identical to other OS X apps. It presents itself on the screen through its windows and views and perhaps also by drawing custom content and presenting and manipulating text. It also handles events using the same path as other apps.
在应用程序运行时生命期间,它跟别的OS X应用程序的行为方式都很相似,但不完全一样。 它在屏幕上通过它的窗口和视图来呈现它自己,可能还通过绘制自定义内容并显示和操作文本。 它也跟别的应用程序一样使用相同的路径处理事件。
An App Presents its User Interface Along with Other Apps and the System
1、一个应用程序和其它应用程序以及系统一起呈现它的用户界面Mac users often see on their screens an assortment of windows and views, some belonging to apps and others belonging to the system itself. The system displays the user’s desktop, the dock, the Finder, and in the menu bar any number of menu extras such as the audio volume control. An app’s user interface is made up of an app-specific menus in the menu bar, one or more windows, and one or more views.
Mac 用户常常在它们的屏幕上看到各式各样的窗口和视图,一些属于应用程序,其它的则属于系统本身。 系统显示用户的桌面,dock,Finder, 以及在菜单栏里任意数量的附加菜单,比如音量控制。 一个应用程序用户界面是由应用程序在菜单栏里指定的菜单,一个或多个窗口,以及一个或多个视图组成。
The menu bar is a repository for commands that the user can perform in the app. The application menu bar stretches across the top of the screen, replacing the menu bar of any other app when the app is foremost. All of an app’s menus in the menu bar are owned by one
NSMenuinstance that’s created by the app when it starts up. Menu commands (or menu items) may apply to the app as a whole, to the currently active window, or to the currently selected object. You are responsible for defining the commands that your app supports and for providing the event-handling code to respond to them. Menu items are
NSMenuItemobjects.
菜单栏是一个命令仓库,这些命令能让用户在应用程序里使用。 应用程序菜单栏横跨屏幕的顶部, 当应用程序在最前面时,菜单栏能替换任何其它应用程序的菜单栏。 NSMenu实例拥有所有在菜单栏里的应用程序菜单,该实例由应用程序在启动时创建。 菜单命令(或菜单项)可能应用于整个应用程序,当前活动窗口,或当前被选择对象。 你负责定义应用程序支持的各种命令,事件处理代码来对这些命令做出响应。菜单项都是NSMenuItem对象。
You use windows and views to present your app’s visual content on the screen and to manage the immediate interactions with that content. Single-window apps have one main window and may have one or more secondary windows or panels. Multiwindow apps have multiple windows for displaying their primary content and may have one or more secondary windows or panels too. The style of a window determines its appearance on the screen. The windows of the system and of apps are interleaved according to specific levels and Z-order. If multiple apps currently are launched, a window of the currently active app is foremost and is shown with its controls enabled. Figure 3 shows the menu bar, along with some standard system and app windows and panels.
你使用窗口和视图在屏幕上呈现应用程序的可是内容,并管理与那内容的及时交互。 单窗口应用程序有一个主窗口,以及含有一个或多个副窗口或面板。多窗口应用程序有多个用来显示它们的主要内容的窗口,并可能也有一个或多个副窗口。 窗口的风格决定了它在屏幕上的外形。系统以及应用程序的窗口根据特定的层次(specific levels)和Z-order互相交错排列。如果多个应用程序正在启动,那么正活跃的应用程序窗口在最前面,并显示了它的各种控制。图3显示了一些标准系统和应用程序的窗口和面板,以及它们的菜单栏。
Figure 3 Windows and menus displayed by apps and the system
图 3 应用程序和系统的窗口和菜单
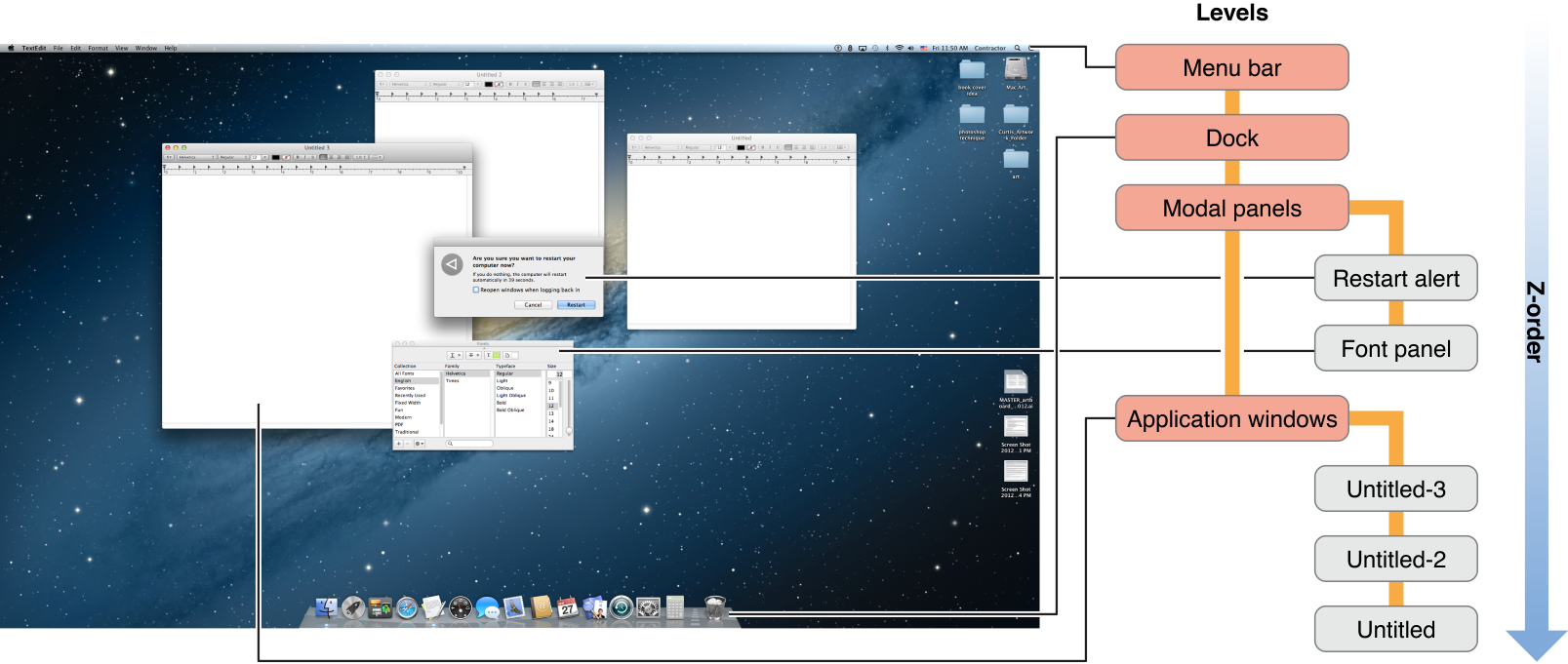
Window Programming Guide contains information about creating and configuring windows. View Programming Guidepresents information about using and creating view hierarchies.
Window Programming Guide --- 窗口编程指南-- 包含了关于创建和设置窗口的各种信息。 View Programming Guide--视图编程指南--描述了关于使用和创建视图层次的信息。
An App Handles Events Originating in User Actions
2、应用程序处理事件起源于用户操作As the window server delivers events to an app, it queues those events and then processes them sequentially in its main run loop. Processing an event involves dispatching the event to the object best suited to handle it.
当窗口服务器给应用程序传送事件时,它给这些事件排队,然后再它的主运行循环里按顺序的处理它们。
Distributing and handling events is the job of responder objects, which are objects inheriting from the
NSResponderclass. An application, its windows and views, window and view controllers, and other objects are all responder objects. An app dispatches an event to the window where it occurred. The window object, in turn, forwards the event to what is called the first responder. In the case of mouse events, the first responder is typically the view object in which the touch or click took place.
发布和处理事件是响应者对象(responder objects)的工作, 响应者对象继承自NSResponder类。 应用程序的窗口和视图,窗口和视图控制器,以及其他对象都是响应者对象。 应用程序发配事件到它发生的那个窗口,而该窗口对象被称为第一相应者(first responder)。 在鼠标事件中,第一响应者通常是触摸或点击发生的那个视图对象。
If the first responder is unable to handle an event, it forwards the event to its next responder, which is typically a parent view, view controller, or window. If that object is unable to handle the event, it forwards it to its next responder, and so on, until the event is handled. This series of linked responder objects is known as the responder chain. Messages continue traveling up the responder chain—toward higher-level responder objects, such as a window controller or the application object—until the event is handled. If the event isn't handled, it is discarded. The responder object that handles an event often sets in motion a series of programmatic actions by the app.
如果第一响应者不能处理事件,它把事件转送给它的下一个响应者,该响应者通常是一个父视图,视图控制器,或窗口。 如果那个对象还是不能处理事件,它继续转送,一个接着一个,直到事件被处理。 这一系列被连接的响应者对象被称为响应者链(responder chain)。 消息继续沿着响应者链向着高级别的响应者对象被传送,比如一个窗口控制器或应用程序对象---直到事件被处理。 如果事件还是没被处理,它就被丢弃。 处理事件的响应者对象往往由应用程序设置了一些列程序操作(programmatic actions)。
Cocoa Event Handling Guide provides more information about responders, the responder chain, and handling events.
Cocoa Event Handling Guide--Cocoa 事件处理指南---提供了更多有关响应者,响应者链,以及处理事件的信息。
Back to App Design
相关文章推荐
- Know the Core Objects of Your App
- The Core Objects of Your App
- [Yii Framework] Yii::app() 包含的对象列表 -- The list objects of Yii::app()
- Please change the value of 'Security.salt' in app/config/core.php to a salt value specific to your a
- Getting the most out of your pixels - adapting to view state changes(WIN8下设计适应多种分辨率的APP)[转.原]
- Update your app to take advantage of the larger aspect ratio on new Android flagship devices
- The value of CFBundleShortVersionString in your WatchKit app's Info.plist (1.0) does not match
- Android应用程序上传错误The package name of your apk may not begin with any of the following values:[com.android, com.google, android, co
- 苹果审核:2.12 We found that the usefulness of your app is limited by the minimal amount of content it in
- 访问字符串对象的内容(Accessing the Content of String Objects)CFString
- Keep the number of files in your /app_code directory small
- We are unable to complete the review of your app since one or more of your In App Purchases have not
- Choosing App Localization For Your App Based On the Target Audience Of the Project
- How to Have Two Versions of the Same App on Your Device
- [笔记] How to get the versionCode and versionName of your app
- 面向对象:With the wonder of your love, the sun above always shines
- Looks like the Spring listener was not configured for your web app!
- [Android]Error running app: This version of Android Studio is incompatible with the Gradle Plugin us
- The Frightening Science of Prediction: How Target & 10 Others Make Money Predicting Your Next Life Event(转摘)
- This version of the rendering library is more recent than your version of ADT plug-in. Please update