Android开发使用Dom从网络端解析xml文件
2013-05-06 23:17
453 查看
本程序实现了从网络端解析xml文件,展示在列表,并实现点击进入相关页面。
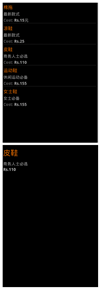

首先我们创建一个类,用来实现http请求和xml文件节点的获取
然后我们创建一个Activity继承与ListActivity,在这个Activity中定义一些节点。
最后实现点击进入一个新的页面的Activity。
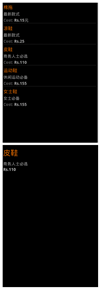

首先我们创建一个类,用来实现http请求和xml文件节点的获取
public class XMLParser { // constructor public XMLParser() { } /** * Getting XML from URL making HTTP request * @param url string * */ public String getXmlFromUrl(String url) { String xml = null; try { // defaultHttpClient DefaultHttpClient httpClient = new DefaultHttpClient(); HttpPost httpPost = new HttpPost(url); HttpResponse httpResponse = httpClient.execute(httpPost); HttpEntity httpEntity = httpResponse.getEntity(); xml = EntityUtils.toString(httpEntity,"utf-8"); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } catch (ClientProtocolException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } // return XML return xml; } /** * Getting XML DOM element * @param XML string * */ public Document getDomElement(String xml){ Document doc = null; DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance(); try { DocumentBuilder db = dbf.newDocumentBuilder(); InputSource is = new InputSource(); is.setCharacterStream(new StringReader(xml)); doc = db.parse(is); } catch (ParserConfigurationException e) { Log.e("Error: ", e.getMessage()); return null; } catch (SAXException e) { Log.e("Error: ", e.getMessage()); return null; } catch (IOException e) { Log.e("Error: ", e.getMessage()); return null; } return doc; } /** Getting node value * @param elem element */ public final String getElementValue( Node elem ) { Node child; if( elem != null){ if (elem.hasChildNodes()){ for( child = elem.getFirstChild(); child != null; child = child.getNextSibling() ){ if( child.getNodeType() == Node.TEXT_NODE ){ return child.getNodeValue(); } } } } return ""; } /** * Getting node value * @param Element node * @param key string * */ public String getValue(Element item, String str) { NodeList n = item.getElementsByTagName(str); return this.getElementValue(n.item(0)); } }
然后我们创建一个Activity继承与ListActivity,在这个Activity中定义一些节点。
public class AndroidXMLParsingActivity extends ListActivity { // All static variables static final String URL = "http://10.0.2.2/biyeshejidata/menu.xml"; // XML node keys static final String KEY_ITEM = "item"; // parent node static final String KEY_ID = "id"; static final String KEY_NAME = "name"; static final String KEY_COST = "cost"; static final String KEY_DESC = "description"; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); ArrayList<HashMap> menuItems = new ArrayList<HashMap>(); XMLParser parser = new XMLParser(); String xml = parser.getXmlFromUrl(URL); // getting XML Document doc = parser.getDomElement(xml); // getting DOM element NodeList nl = doc.getElementsByTagName(KEY_ITEM); // looping through all item nodes for (int i = 0; i < nl.getLength(); i++) { // creating new HashMap HashMap map = new HashMap(); Element e = (Element) nl.item(i); // adding each child node to HashMap key => value map.put(KEY_ID, parser.getValue(e, KEY_ID)); map.put(KEY_NAME, parser.getValue(e, KEY_NAME)); map.put(KEY_COST, "Rs." + parser.getValue(e, KEY_COST)); map.put(KEY_DESC, parser.getValue(e, KEY_DESC)); // adding HashList to ArrayList menuItems.add(map); } // Adding menuItems to ListView ListAdapter adapter = new SimpleAdapter(this, menuItems, R.layout.list_item, new String[] { KEY_NAME, KEY_DESC, KEY_COST }, new int[] { R.id.name, R.id.desciption, R.id.cost }); setListAdapter(adapter); // selecting single ListView item ListView lv = getListView(); lv.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { // getting values from selected ListItem String name = ((TextView) view.findViewById(R.id.name)).getText().toString(); String cost = ((TextView) view.findViewById(R.id.cost)).getText().toString(); String description = ((TextView) view.findViewById(R.id.desciption)).getText().toString(); // Starting new intent Intent in = new Intent(getApplicationContext(), SingleMenuItemActivity.class); in.putExtra(KEY_NAME, name); in.putExtra(KEY_COST, cost); in.putExtra(KEY_DESC, description); startActivity(in); } }); } }
最后实现点击进入一个新的页面的Activity。
public class SingleMenuItemActivity extends Activity { // XML node keys static final String KEY_NAME = "name"; static final String KEY_COST = "cost"; static final String KEY_DESC = "description"; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.single_list_item); // getting intent data Intent in = getIntent(); // Get XML values from previous intent String name = in.getStringExtra(KEY_NAME); String cost = in.getStringExtra(KEY_COST); String description = in.getStringExtra(KEY_DESC); // Displaying all values on the screen TextView lblName = (TextView) findViewById(R.id.name_label); TextView lblCost = (TextView) findViewById(R.id.cost_label); TextView lblDesc = (TextView) findViewById(R.id.description_label); lblName.setText(name); lblCost.setText(cost); lblDesc.setText(description); } }
相关文章推荐
- 【Android网络开发の1】XML之DOM方式 解析和生成XML文件 推荐
- Android开发本地及网络Mp3音乐播放器(十一)使用Jsoup组件请求网络,并解析音乐数据
- android应用开发之利用SAX、DOM和Pull实现对XML文件的解析并进行单元测试
- Android开发学习---使用XmlPullParser解析xml文件
- RSS阅读器(使用android解析技术解析网络中的xml文件并以列表的形式显示出来)
- Android网络开发中如何使用JSON进行网络通信---Android_JSON数据通讯方法解析
- Android网络开发中如何使用JSON进行网络通信---Android JSON数据通讯方法解析
- Android异步下载网络图片&android解析xml文件的方式&使用Adapter为ListView提供数据
- 【Android网络开发の2】XML之SAX方式 解析和生成XML文件
- android如何使用DOM和SAXParserFactory来解析XML文件
- Android中的XML解析-DOM的使用与开发技巧(转)
- 在android开发中,经常用到去解析xml文件,常见的解析xml的方式有一下三种:SAX、Pull、Dom解析方式。最近做了一个android版的CSDN阅读器,用到了其中的两种(sax,pull)
- Android网络编程之二:DOM解析XML文件
- Android开发之DOM解析xml文件的方法
- Android演示使用DOM解析xml文件
- android学习——使用SAX、DOM 和 PULL 解析xml文件,及使用pull生成xml文件
- Android开发进阶(六)--原始XML文件的使用以及PULL解析小例
- Android异步下载网络图片&android解析xml文件的方式&使用Adapter为ListView提供数据
- Android学习笔记---Android平台1.使用dom解析xml文件
- Android中的XML解析-DOM的使用与开发技巧