CSharp设计模式读书笔记(4):单例模式(学习难度:★☆☆☆☆,使用频率:★★★★☆)
2012-11-28 15:23
323 查看
单例模式(Singleton Pattern):确保某一个类只有一个实例,而且自行实例化并向整个系统提供这个实例,这个类称为单例类,它提供全局访问的方法。
模式角色与结构:
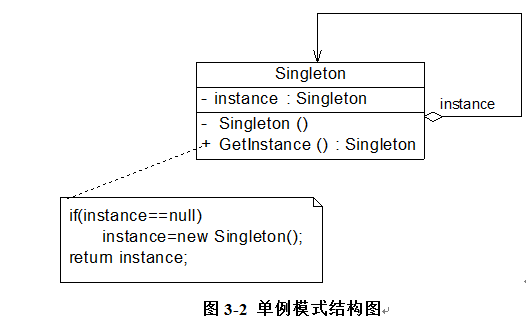
示例代码:
模式角色与结构:
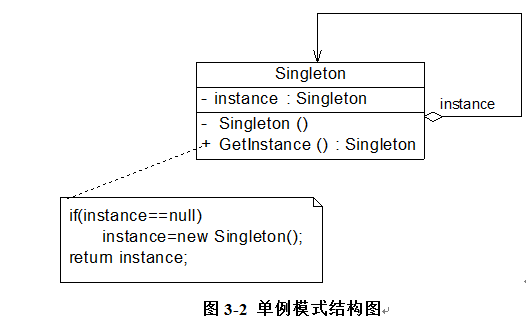
示例代码:
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace CSharp.DesignPattern.SingletonPattern { class Program { static void Main(string[] args) { Singleton singleton = Singleton.Instance; singleton.Display(); Console.ReadLine(); } } //// 简单实现。缺点:不是线程安全的实现方法。优点:实现了延迟初始化。 //// 注意:类定义为sealed. //sealed class Singleton //{ // static Singleton _instance = null; // public static Singleton Instance // { // get // { // if (_instance == null) // { // _instance = new Singleton(); // } // return _instance; // } // } // public void Display() // { // Console.WriteLine("Simple implement singleton..."); // } //} //// 安全的线程。缺点:每次加锁,增加开销,损失了性能。优点:实现了延迟初始化。 //sealed class Singleton //{ // static Singleton _instance = null; // static readonly Object padlock = new Object(); // public static Singleton Instance // { // get // { // lock (padlock) // { // if (_instance == null) // { // _instance = new Singleton(); // } // return _instance; // } // } // } // public void Display() // { // Console.WriteLine("Thread safety singleton..."); // } //} //// 判定后加锁。优点:线程安全,不是每次都加锁,实现了延迟初始化。 //sealed class Singleton //{ // static Singleton _instance = null; // static readonly Object padlock = new Object(); // public static Singleton Instance // { // get // { // if (_instance == null) // { // lock (padlock) // { // if (_instance == null) // { // _instance = new Singleton(); // } // } // } // return _instance; // } // } // public void Display() // { // Console.WriteLine("Add lock after judgement singleton..."); // } //} //// 静态初始化。缺点:不能实现延迟初始化。优点:简单常用 //sealed class Singleton //{ // static readonly Singleton _instance = new Singleton(); // public static Singleton Instance // { // get // { // return _instance; // } // } // public void Display() // { // Console.WriteLine("Static initiate singleton..."); // } //} // 延迟初始化。值得推荐。 sealed class Singleton { public static Singleton Instance { get { return Nested._instance; } } class Nested // 嵌套类或者内部类 { internal static readonly Singleton _instance = new Singleton(); } public void Display() { Console.WriteLine("Delayed initiate singleton..."); } } }
相关文章推荐
- CSharp设计模式读书笔记(22):策略模式(学习难度:★☆☆☆☆,使用频率:★★★★☆)
- CSharp设计模式读书笔记(23):模板方法模式(学习难度:★★☆☆☆,使用频率:★★★☆☆)
- CSharp设计模式读书笔记(10):装饰模式(学习难度:★★★☆☆,使用频率:★★★☆☆)
- CSharp设计模式读书笔记(5):原型模式(学习难度:★★★☆☆,使用频率:★★★☆☆)
- CSharp设计模式读书笔记(11):外观模式(学习难度:★☆☆☆☆,使用频率:★★★★★)
- CSharp设计模式读书笔记(24):访问者模式(学习难度:★★★★☆,使用频率:★☆☆☆☆)
- CSharp设计模式读书笔记(18):中介者模式(学习难度:★★★☆☆,使用频率:★★☆☆☆)
- CSharp设计模式读书笔记(13):代理模式(学习难度:★★★☆☆,使用频率:★★★★☆)
- CSharp设计模式读书笔记(8):桥接模式(学习难度:★★★☆☆,使用频率:★★★☆☆)
- CSharp设计模式读书笔记(9):组合模式(学习难度:★★★☆☆,使用频率:★★★★☆)
- CSharp设计模式读书笔记(14):职责链模式(学习难度:★★★☆☆,使用频率:★★☆☆☆)
- CSharp设计模式读书笔记(6):建造者模式(学习难度:★★★★☆,使用频率:★★☆☆☆)
- CSharp设计模式读书笔记(20):观察者模式(学习难度:★★★☆☆,使用频率:★★★★★)
- CSharp设计模式读书笔记(15):命令模式(学习难度:★★★☆☆,使用频率:★★★★☆)
- CSharp设计模式读书笔记(21):状态模式(学习难度:★★★☆☆,使用频率:★★★☆☆)
- CSharp设计模式读书笔记(16):解释器模式(学习难度:★★★★★,使用频率:★☆☆☆☆)
- 六个创建型模式2:工厂方法模式-Factory Method Pattern 【学习难度:★★☆☆☆,使用频率:★★★★★】
- 七个结构型模式7:代理模式-Proxy Pattern【学习难度:★★★☆☆,使用频率:★★★★☆】
- 十一个行为型模式6:备忘录模式-Memento Pattern【学习难度:★★☆☆☆,使用频率:★★☆☆☆
- CSharp设计模式读书笔记(1):简单工厂模式(学习难度:★★☆☆☆,使用频率:★★★☆☆)