在C++中应用Google Chrome脚本引擎——V8
2012-08-28 23:05
597 查看
原文地址:http://www.codeproject.com/KB/library/Using_V8_Javascript_VM.aspx
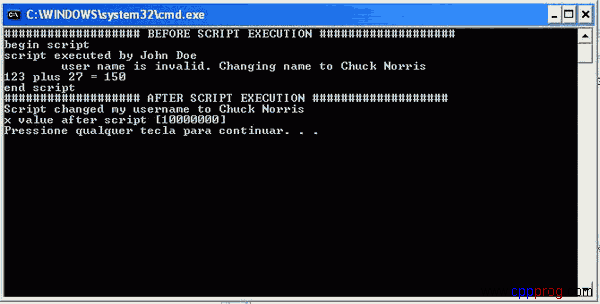
介绍
谁不想知道虚拟机是怎样工作的?不过,比起自己写一个虚拟机,更好的办法是使用大公司的产品。在这篇文章中,我将介绍如何在你的程序中使用V8——谷歌浏览器(Chrome)所使用的开源JavaScript引擎。
背景
这里的代码使用V8作为嵌入库来执行JavaScript代码。要取得库源码和其它信息,可以浏览V8开发者页面。想有效地应用V8,你需要了解C/C++和JavaScript。
使用
我们来看看演示中有哪些东西:
· 如何使用V8库API来执行JavaScript脚本。
· 如何存取脚本中的整数和字符串。
· 如何建立可被脚本调用的自定义函数。
· 如何建立可被脚本调用的自定义类。
首先,我们一起了解一下怎样初始化V8。这是嵌入V8引擎的简单例子:
1. #include <v8.h>
2. using namespace v8;
3. int main(int argc, char* argv[]) {
4. // Create a stack-allocated handle scope.
5. HandleScope handle_scope;
6. // Create a new context.
7. Handle<Context> context = Context::New();
8. // Enter the created context for compiling and
9. // running the hello world script.
10. Context::Scope context_scope(context);
11. // Create a string containing the JavaScript source code.
12. Handle<String> source = String::New("'Hello' + ', World!'");
13. // Compile the source code.
14. Handle<Script> script = Script::Compile(source);
15. // Run the script to get the result.
16. Handle<Value> result = script->Run();
17. // Convert the result to an ASCII string and print it.
18. String::AsciiValue ascii(result);
19. printf("%s ", *ascii);
20. return 0;
21. }
好了,不过这还不能说明怎样让我们控制脚本中的变量和函数。
全局模型(The Global Template)
首先,我们需要一个全局模型来掌控我们所做的修改:
1. v8::Handle<v8::ObjectTemplate> global = v8::ObjectTemplate::New();
这里建立了一个新的全局模型来管理我们的上下文(context)和定制。在V8里,每个上下文是分开的,它们有自己的全局模型。一个上下文就是一个独立的执行环境,相互之间没有关联,JavaScript运行于其中一个实例之中。
自定义函数
接下来,我们加入一个名为"plus"的自定义函数:
1. // plus function implementation - Add two numbers
2. v8::Handle<v8::Value> Plus(const v8::Arguments& args)
3. {
4. unsigned int A = args[0]->Uint32Value();
5. unsigned int B = args[1]->Uint32Value();
6. return v8_uint32(A + B);
7. }
8. //...
9. //associates plus on script to the Plus function
10. global->Set(v8::String::New("plus"), v8::FunctionTemplate::New(Plus));
自定义函数必须以const v8::Arguments&作为参数并返回v8::Handle<v8::Value>。我们把这个函数加入到模型中,关联名称"plus"到回调Plus。现在,在脚本中每次调用"plus",我们的Plus函数就会被调用。这个函数只是返回两个参数的和。
现在我们可以在JavaScript里使用这个自定义函数了:
plus(120,44);
在脚本里也可以得到函数的返回值:
x = plus(1,2);
if( x == 3){
// do something important here!
}
访问器(Accessor)——存取脚本中的变量
现在,我们可以建立函数了...不过如果我们可以在脚本外定义一些东西岂不是更酷?Let's do it! V8里有个东东称为存取器(Accessor),使用它,我们可以关联一个名称到一对Get/Set函数上,V8会用它来存取脚本中的变量。
1. global->SetAccessor(v8::String::New("x"), XGetter, XSetter);
这行代码关联名称"x"到XGetter和XSetter函数。这样在脚本中每次读取到"x"变量时都会调用XGetter,每次更新"x"变量时会调用XSetter。下面是这两个函数的代码:
1. //the x variable!
2. int x;
3. //get the value of x variable inside javascript
4. static v8::Handle<v8::Value> XGetter( v8::Local<v8::String> name,
5. const v8::AccessorInfo& info) {
6. return v8::Number::New(x);
7. }
8. //set the value of x variable inside javascript
9. static void XSetter( v8::Local<v8::String> name,
10. v8::Local<v8::Value> value, const v8::AccessorInfo& info) {
11. x = value->Int32Value();
12. }
XGetter里我们把"x"转换成V8喜欢的数值类型。XSetter里,我们把传入的参数转换成整数,Int32Value是基本类型转换函数的一员,还有NumberValue对应double、BooleanValue对应bool,等。
现在,我们可以为字符串做相同的操作:
1. //the username accessible on c++ and inside the script
2. char username[1024];
3. //get the value of username variable inside javascript
4. v8::Handle<v8::Value> userGetter(v8::Local<v8::String> name,
5. const v8::AccessorInfo& info) {
6. return v8::String::New((char*)&username,strlen((char*)&username));
7. }
8. //set the value of username variable inside javascript
9. void userSetter(v8::Local<v8::String> name, v8::Local<v8::Value> value,
10. const v8::AccessorInfo& info) {
11. v8::Local<v8::String> s = value->ToString();
12. s->WriteAscii((char*)&username);
13. }
对于字符串,有一点点不同,"userGetter"和XGetter做的一样,不过userSetter要先用ToString方法取得内部字符串,然后用WriteAscii函数把内容写到我们指定的内存中。现在,加入存取器:
1. //create accessor for string username
2. global->SetAccessor(v8::String::New("user"),userGetter,userSetter);
打印输出
"print"函数是另一个自定义函数,它通过"printf"输出所有的参数内容。和之前的"plus"函数一样,我们要在全局模型中注册这个函数:
1. //associates print on script to the Print function
2. global->Set(v8::String::New("print"), v8::FunctionTemplate::New(Print));
实现"print"函数
1. // The callback that is invoked by v8 whenever the JavaScript 'print'
2. // function is called. Prints its arguments on stdout separated by
3. // spaces and ending with a newline.
4. v8::Handle<v8::Value> Print(const v8::Arguments& args) {
5. bool first = true;
6. for (int i = 0; i < args.Length(); i++)
7. {
8. v8::HandleScope handle_scope;
9. if (first)
10. {
11. first = false;
12. }
13. else
14. {
15. printf(" ");
16. }
17. //convert the args[i] type to normal char* string
18. v8::String::AsciiValue str(args[i]);
19. printf("%s", *str);
20. }
21. printf(" ");
22. //returning Undefined is the same as returning void...
23. return v8::Undefined();
24. }
这里,为每个参数都构建了v8::String::AsciiValue对象:数据的char*表示。通过它,我们就可以把所有类型都转换成字符串并打印出来。
JavaScript演示
在演示程序里,我们有一个简单的JavaScript脚本,调用了迄今为止我们建立的所有东西:
print("begin script");
print(script executed by + user);
if ( user == "John Doe"){
print("\tuser name is invalid. Changing name to Chuck Norris");
user = "Chuck Norris";
}
print("123 plus 27 = " + plus(123,27));
x = plus(3456789,6543211);
print("end script");
存取C++对象
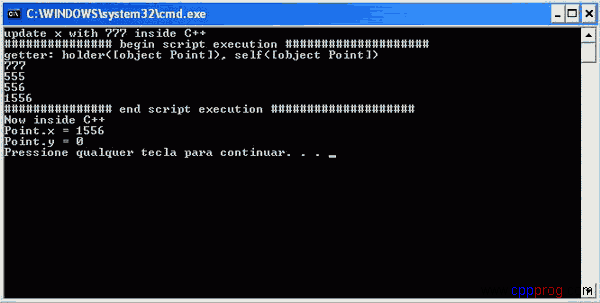
为我们的类准备环境
如果用C++把一个类映射到JavaScript中去?放一个演示用的类上来先:
1. //Sample class mapped to v8
2. class Point
3. {
4. public:
5. //constructor
6. Point(int x, int y):x_(x),y_(y){}
7.
8. //internal class functions
9. //just increment x_
10. void Function_A(){++x_; }
11.
12. //increment x_ by the amount
13. void Function_B(int vlr){x_+=vlr;}
14.
15. //variables
16. int x_;
17. };
为了把这个类完全嵌入脚本中,我们需要映射类成员函数和类成员变量。第一步是在我们的上下文中映射一个类模型(class template):
1. Handle<FunctionTemplate> point_templ = FunctionTemplate::New();
2. point_templ->SetClassName(String::New("Point"));
我们建立了一个"函数"模型[FunctionTemplate],但这里应该把它看成类。
然后,我们通过原型模型(Prototype Template)加入内建的类方法:
1. Handle<ObjectTemplate> point_proto = point_templ->PrototypeTemplate();
2. point_proto->Set("method_a", FunctionTemplate::New(PointMethod_A));
3. point_proto->Set("method_b", FunctionTemplate::New(PointMethod_B));
接下来,类有了两个方法和对应的回调。但它们目前只在原型中,没有类实例访问器我们还不能使用它们。
1. Handle<ObjectTemplate> point_inst = point_templ->InstanceTemplate();
2. point_inst->SetInternalFieldCount(1);
SetInternalFieldCount函数为C++类建立一个空间(后面会用到)。
现在,我们有了类实例,加入访问器以访问内部变量:
1. point_inst->SetAccessor(String::New("x"), GetPointX, SetPointX);
接着,“土壤”准备好了,开始播种:
1. Point* p = new Point(0, 0);
新对象建立好了,目前只能在C++中使用,要放到脚本里,我们还要下面的代码:
1. Handle<Function> point_ctor = point_templ->GetFunction();
2. Local<Object> obj = point_ctor->NewInstance();
3. obj->SetInternalField(0, External::New(p));
好了,GetFunction返回一个point构造器(JavaScript方面), 通过它,我们可以用NewInstance生成一个新的实例。然后,用Point对象指针设置我们的内部域(我们前面用SetInternalFieldCount建立的空间),JavaScript可以通过这个指针存取对象。
还少了一步,我们只有类模型和实例,但还缺一个名字来存取它:
1. context->Global()->Set(String::New("point"), obj);
在JavaScript里访问类方法
最后,我们还要解释一下怎样在Point类中访问Function_A...
让我们看看PointMethod_A回调:
1. Handle<Value> PointMethod_A(const Arguments& args)
2. {
3. Local<Object> self = args.Holder();
4. Local<External> wrap = Local<External>::Cast(self->GetInternalField(0));
5. void* ptr = wrap->Value();
6. static_cast<Point*>(ptr)->Function_A();
7. return Integer::New(static_cast<Point*>(ptr)->x_);
8. }
和普通访问器一样,我们必须处理参数。要访问我们的类,必须从内部域(第一个)中取得类指针。把内部域映射到"wrap"之后,我们使用它的"value"方法取得类指针。
其它
希望这篇文章对你有所帮助,如果发现文章有误,请不吝赐教。
Google的V8参考文档
许可
This article, along with any associated source code and files, is licensed under A Public Domain dedication
关于作者
GabrielWF
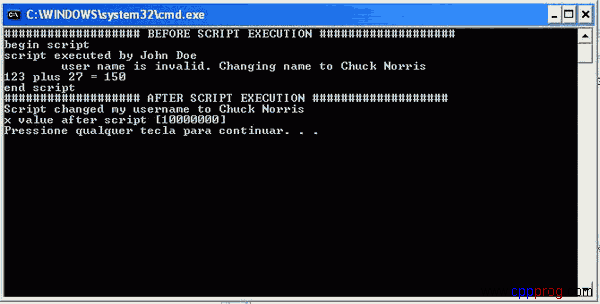
介绍
谁不想知道虚拟机是怎样工作的?不过,比起自己写一个虚拟机,更好的办法是使用大公司的产品。在这篇文章中,我将介绍如何在你的程序中使用V8——谷歌浏览器(Chrome)所使用的开源JavaScript引擎。
背景
这里的代码使用V8作为嵌入库来执行JavaScript代码。要取得库源码和其它信息,可以浏览V8开发者页面。想有效地应用V8,你需要了解C/C++和JavaScript。
使用
我们来看看演示中有哪些东西:
· 如何使用V8库API来执行JavaScript脚本。
· 如何存取脚本中的整数和字符串。
· 如何建立可被脚本调用的自定义函数。
· 如何建立可被脚本调用的自定义类。
首先,我们一起了解一下怎样初始化V8。这是嵌入V8引擎的简单例子:
1. #include <v8.h>
2. using namespace v8;
3. int main(int argc, char* argv[]) {
4. // Create a stack-allocated handle scope.
5. HandleScope handle_scope;
6. // Create a new context.
7. Handle<Context> context = Context::New();
8. // Enter the created context for compiling and
9. // running the hello world script.
10. Context::Scope context_scope(context);
11. // Create a string containing the JavaScript source code.
12. Handle<String> source = String::New("'Hello' + ', World!'");
13. // Compile the source code.
14. Handle<Script> script = Script::Compile(source);
15. // Run the script to get the result.
16. Handle<Value> result = script->Run();
17. // Convert the result to an ASCII string and print it.
18. String::AsciiValue ascii(result);
19. printf("%s ", *ascii);
20. return 0;
21. }
好了,不过这还不能说明怎样让我们控制脚本中的变量和函数。
全局模型(The Global Template)
首先,我们需要一个全局模型来掌控我们所做的修改:
1. v8::Handle<v8::ObjectTemplate> global = v8::ObjectTemplate::New();
这里建立了一个新的全局模型来管理我们的上下文(context)和定制。在V8里,每个上下文是分开的,它们有自己的全局模型。一个上下文就是一个独立的执行环境,相互之间没有关联,JavaScript运行于其中一个实例之中。
自定义函数
接下来,我们加入一个名为"plus"的自定义函数:
1. // plus function implementation - Add two numbers
2. v8::Handle<v8::Value> Plus(const v8::Arguments& args)
3. {
4. unsigned int A = args[0]->Uint32Value();
5. unsigned int B = args[1]->Uint32Value();
6. return v8_uint32(A + B);
7. }
8. //...
9. //associates plus on script to the Plus function
10. global->Set(v8::String::New("plus"), v8::FunctionTemplate::New(Plus));
自定义函数必须以const v8::Arguments&作为参数并返回v8::Handle<v8::Value>。我们把这个函数加入到模型中,关联名称"plus"到回调Plus。现在,在脚本中每次调用"plus",我们的Plus函数就会被调用。这个函数只是返回两个参数的和。
现在我们可以在JavaScript里使用这个自定义函数了:
plus(120,44);
在脚本里也可以得到函数的返回值:
x = plus(1,2);
if( x == 3){
// do something important here!
}
访问器(Accessor)——存取脚本中的变量
现在,我们可以建立函数了...不过如果我们可以在脚本外定义一些东西岂不是更酷?Let's do it! V8里有个东东称为存取器(Accessor),使用它,我们可以关联一个名称到一对Get/Set函数上,V8会用它来存取脚本中的变量。
1. global->SetAccessor(v8::String::New("x"), XGetter, XSetter);
这行代码关联名称"x"到XGetter和XSetter函数。这样在脚本中每次读取到"x"变量时都会调用XGetter,每次更新"x"变量时会调用XSetter。下面是这两个函数的代码:
1. //the x variable!
2. int x;
3. //get the value of x variable inside javascript
4. static v8::Handle<v8::Value> XGetter( v8::Local<v8::String> name,
5. const v8::AccessorInfo& info) {
6. return v8::Number::New(x);
7. }
8. //set the value of x variable inside javascript
9. static void XSetter( v8::Local<v8::String> name,
10. v8::Local<v8::Value> value, const v8::AccessorInfo& info) {
11. x = value->Int32Value();
12. }
XGetter里我们把"x"转换成V8喜欢的数值类型。XSetter里,我们把传入的参数转换成整数,Int32Value是基本类型转换函数的一员,还有NumberValue对应double、BooleanValue对应bool,等。
现在,我们可以为字符串做相同的操作:
1. //the username accessible on c++ and inside the script
2. char username[1024];
3. //get the value of username variable inside javascript
4. v8::Handle<v8::Value> userGetter(v8::Local<v8::String> name,
5. const v8::AccessorInfo& info) {
6. return v8::String::New((char*)&username,strlen((char*)&username));
7. }
8. //set the value of username variable inside javascript
9. void userSetter(v8::Local<v8::String> name, v8::Local<v8::Value> value,
10. const v8::AccessorInfo& info) {
11. v8::Local<v8::String> s = value->ToString();
12. s->WriteAscii((char*)&username);
13. }
对于字符串,有一点点不同,"userGetter"和XGetter做的一样,不过userSetter要先用ToString方法取得内部字符串,然后用WriteAscii函数把内容写到我们指定的内存中。现在,加入存取器:
1. //create accessor for string username
2. global->SetAccessor(v8::String::New("user"),userGetter,userSetter);
打印输出
"print"函数是另一个自定义函数,它通过"printf"输出所有的参数内容。和之前的"plus"函数一样,我们要在全局模型中注册这个函数:
1. //associates print on script to the Print function
2. global->Set(v8::String::New("print"), v8::FunctionTemplate::New(Print));
实现"print"函数
1. // The callback that is invoked by v8 whenever the JavaScript 'print'
2. // function is called. Prints its arguments on stdout separated by
3. // spaces and ending with a newline.
4. v8::Handle<v8::Value> Print(const v8::Arguments& args) {
5. bool first = true;
6. for (int i = 0; i < args.Length(); i++)
7. {
8. v8::HandleScope handle_scope;
9. if (first)
10. {
11. first = false;
12. }
13. else
14. {
15. printf(" ");
16. }
17. //convert the args[i] type to normal char* string
18. v8::String::AsciiValue str(args[i]);
19. printf("%s", *str);
20. }
21. printf(" ");
22. //returning Undefined is the same as returning void...
23. return v8::Undefined();
24. }
这里,为每个参数都构建了v8::String::AsciiValue对象:数据的char*表示。通过它,我们就可以把所有类型都转换成字符串并打印出来。
JavaScript演示
在演示程序里,我们有一个简单的JavaScript脚本,调用了迄今为止我们建立的所有东西:
print("begin script");
print(script executed by + user);
if ( user == "John Doe"){
print("\tuser name is invalid. Changing name to Chuck Norris");
user = "Chuck Norris";
}
print("123 plus 27 = " + plus(123,27));
x = plus(3456789,6543211);
print("end script");
存取C++对象
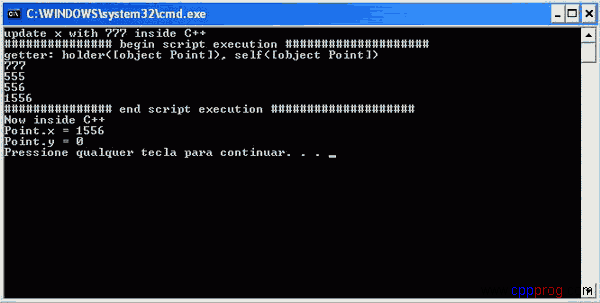
为我们的类准备环境
如果用C++把一个类映射到JavaScript中去?放一个演示用的类上来先:
1. //Sample class mapped to v8
2. class Point
3. {
4. public:
5. //constructor
6. Point(int x, int y):x_(x),y_(y){}
7.
8. //internal class functions
9. //just increment x_
10. void Function_A(){++x_; }
11.
12. //increment x_ by the amount
13. void Function_B(int vlr){x_+=vlr;}
14.
15. //variables
16. int x_;
17. };
为了把这个类完全嵌入脚本中,我们需要映射类成员函数和类成员变量。第一步是在我们的上下文中映射一个类模型(class template):
1. Handle<FunctionTemplate> point_templ = FunctionTemplate::New();
2. point_templ->SetClassName(String::New("Point"));
我们建立了一个"函数"模型[FunctionTemplate],但这里应该把它看成类。
然后,我们通过原型模型(Prototype Template)加入内建的类方法:
1. Handle<ObjectTemplate> point_proto = point_templ->PrototypeTemplate();
2. point_proto->Set("method_a", FunctionTemplate::New(PointMethod_A));
3. point_proto->Set("method_b", FunctionTemplate::New(PointMethod_B));
接下来,类有了两个方法和对应的回调。但它们目前只在原型中,没有类实例访问器我们还不能使用它们。
1. Handle<ObjectTemplate> point_inst = point_templ->InstanceTemplate();
2. point_inst->SetInternalFieldCount(1);
SetInternalFieldCount函数为C++类建立一个空间(后面会用到)。
现在,我们有了类实例,加入访问器以访问内部变量:
1. point_inst->SetAccessor(String::New("x"), GetPointX, SetPointX);
接着,“土壤”准备好了,开始播种:
1. Point* p = new Point(0, 0);
新对象建立好了,目前只能在C++中使用,要放到脚本里,我们还要下面的代码:
1. Handle<Function> point_ctor = point_templ->GetFunction();
2. Local<Object> obj = point_ctor->NewInstance();
3. obj->SetInternalField(0, External::New(p));
好了,GetFunction返回一个point构造器(JavaScript方面), 通过它,我们可以用NewInstance生成一个新的实例。然后,用Point对象指针设置我们的内部域(我们前面用SetInternalFieldCount建立的空间),JavaScript可以通过这个指针存取对象。
还少了一步,我们只有类模型和实例,但还缺一个名字来存取它:
1. context->Global()->Set(String::New("point"), obj);
在JavaScript里访问类方法
最后,我们还要解释一下怎样在Point类中访问Function_A...
让我们看看PointMethod_A回调:
1. Handle<Value> PointMethod_A(const Arguments& args)
2. {
3. Local<Object> self = args.Holder();
4. Local<External> wrap = Local<External>::Cast(self->GetInternalField(0));
5. void* ptr = wrap->Value();
6. static_cast<Point*>(ptr)->Function_A();
7. return Integer::New(static_cast<Point*>(ptr)->x_);
8. }
和普通访问器一样,我们必须处理参数。要访问我们的类,必须从内部域(第一个)中取得类指针。把内部域映射到"wrap"之后,我们使用它的"value"方法取得类指针。
其它
希望这篇文章对你有所帮助,如果发现文章有误,请不吝赐教。
Google的V8参考文档
许可
This article, along with any associated source code and files, is licensed under A Public Domain dedication
关于作者
GabrielWF
相关文章推荐
- 在C++中应用Google Chrome脚本引擎——V8
- [V8] 在C++中应用Google Chrome脚本引擎——V8
- 使用 SpiderMonkey 使 C++应用支持 JavaScript 脚本引擎
- 使用 SpiderMonkey 使 C++应用支持 JavaScript 脚本引擎
- 使用 SpiderMonkey 使 C++应用支持 JavaScript 脚本引擎
- 使用 SpiderMonkey 使 C++应用支持 JavaScript 脚本引擎
- 我是如何用 V8 脚本引擎替换JScript的 —— (一)前言
- Linux Ubuntu下Google Chrome V8引擎的编译实战(原创)
- 我是如何用 V8 脚本引擎替换JScript的 —— (二)准备1
- Java版AVG游戏开发入门示例[3]——脚本引擎的制作及应用
- 我是如何用 V8 脚本引擎替换JScript的 —— (二)准备1
- 我是如何用 V8 脚本引擎替换JScript的 —— (四)准备3
- Linux 下使用在C++中V8引擎的环境配置
- 我是如何用 V8 脚本引擎替换JScript的 —— (四)准备3
- V8引擎javascript与C++交互
- JavaScript V8做为脚本引擎的可行性
- Java版AVG游戏开发入门示例[3]——脚本引擎的制作及应用
- 我是如何用 V8 脚本引擎替换JScript的 —— (三)准备2
- 详解Node.js API系列C/C++ Addons(2) Google V8引擎
- JS脚本可视化调试支持——基于Google v8引擎的脚本调试