spring aop的使用(注解方式以及基于xml配置方式)
2012-08-06 15:28
1266 查看
注解方式
*******************
beans.xml
*******************
***************
MyInterceptor.java
***************
***************
PersonService.java
***************
***************
PersonServiceBean.java
***************
***************
SpringAOPTest.java
***************
***************
运行结果
***************

基于xml配置方式
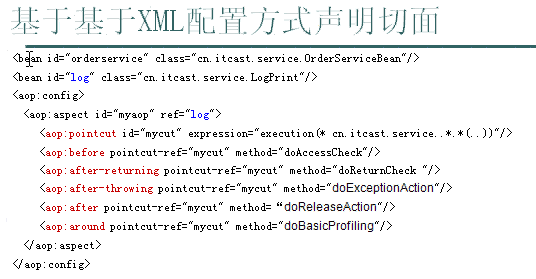
*************
beans.xml
************
*******************
MyInterceptor.java
*******************
aop表达式的使用方法
<aop:pointcut id="point" expression="execution (!void blog.service.impl.PersonServiceBean.*(..))"/>
!void blog.service.impl.PersonServiceBean.*(..)中!void 表示拦截所有返回类型为非void类型的方法
java.lang.String表示拦截所有返回类型为String类型的方法
* blog.service.impl.PersonServiceBean.*(java.lang.String,..)表示拦截所有第一个参数为String类型的方法
*******************
beans.xml
*******************
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd "> <aop:aspectj-autoproxy/> <bean id="myInterceptor" class="blog.service.MyInterceptor"/> <bean id="personService" class="blog.service.impl.PersonServiceBean"></bean> </beans>
***************
MyInterceptor.java
***************
package blog.service; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.AfterReturning; import org.aspectj.lang.annotation.AfterThrowing; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.aspectj.lang.annotation.Pointcut; /** * 切面 * */ @Aspect public class MyInterceptor { @Pointcut("execution (* blog.service.impl.PersonServiceBean.*(..))") public void anyMethod() { //表达式解释:* blog.service.impl.PersonServiceBean.*(..):第一个*号表示返回类型; //blog.service.impl.PersonServiceBean.*:表示PersonServiceBean类下的所有方法 //blog.service.impl..*.*:表示blog.service.impl包及其子包下的所有类的所有方法 //(..):表示所有的参数类型几个数都不限 }// 声明一个切入点 //会拦截参数签名位 (String , int)或(String , Integer )类型的方法, //参数顺序与args(uname,id)一致,参数类型由(int id,String uname)决定 @Before("anyMethod() && args(uname,id)") public void doAccessCheck(int id,String uname) { System.out.println("前置通知:" + uname + id); } @AfterReturning(pointcut="anyMethod()",returning="result") public void doAfterReturning(String result) { System.out.println("后置通知:" + result); } @After("anyMethod()") public void doAfter() { System.out.println("最终通知"); } @AfterThrowing(pointcut="anyMethod()",throwing="e") public void doAfterThrowing(Exception e) { System.out.println("例外通知:" + e.getMessage()); } @Around("anyMethod()") public Object doBasicProfiling(ProceedingJoinPoint pjp) throws Throwable{ //if(){//判断用户是否有权限 System.out.println("进入方法"); Object result = pjp.proceed(); System.out.println("退出方法"); //} return result; } }
***************
PersonService.java
***************
package blog.service; public interface PersonService { public String save(String name); public String update(String name,Integer userId); public String delete(int id,String dept); }
***************
PersonServiceBean.java
***************
package blog.service.impl; import blog.service.PersonService; public class PersonServiceBean implements PersonService { private String username = null; public String getUsername() { return username; } public PersonServiceBean() { } public PersonServiceBean(String username) { this.username = username; } @Override public String save(String name) { if (name.equals("") || name == null) { throw new RuntimeException("出错啦"); } System.out.println("in save method!" + name); return "in save method!" + name; } @Override public String update(String name, Integer userId) { System.out.println("in update method! name = " + name + " id = " + userId + " username = " + username); return "in update method!name = " + name + " id = " + userId; } @Override public String delete(int id, String dept) { System.out.println("id = " + id + " dept = " + dept); return "in delete method! + nameid = " + id + " dept = " + dept; } }
***************
SpringAOPTest.java
***************
package junitTest; import org.junit.BeforeClass; import org.junit.Test; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import blog.service.PersonService; public class SpringAOPTest { @BeforeClass public static void setUpBeforeClass() throws Exception { } @Test public void AopTest(){ ApplicationContext ctx = new ClassPathXmlApplicationContext("beans.xml"); PersonService service = (PersonService)ctx.getBean("personService"); try { service.delete(12, "it"); service.update("发多个地方",324); service.save(""); } catch (Exception e) { e.printStackTrace(); } } }
***************
运行结果
***************

基于xml配置方式
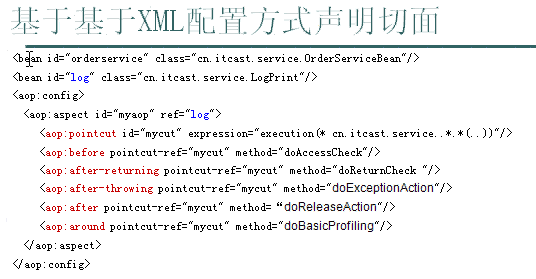
*************
beans.xml
************
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd "> <aop:aspectj-autoproxy/> <bean id="myInterceptor" class="blog.service.MyInterceptor"/> <bean id="personService" class="blog.service.impl.PersonServiceBean"></bean> <aop:config> <aop:aspect id="aspectBean" ref="myInterceptor" > <aop:pointcut id="point" expression="execution (!void blog.service.impl.PersonServiceBean.*(..))"/> <aop:before method="doAccessCheck" pointcut-ref="point" /> <aop:after-returning method="doAfterReturning" pointcut-ref="point" /> <aop:after-throwing method="doAfterThrowing" pointcut-ref="point"/> <aop:after method="doAfter" pointcut-ref="point"/> <aop:around method="doBasicProfiling" pointcut-ref="point" /> </aop:aspect> </aop:config> </beans>
*******************
MyInterceptor.java
*******************
package blog.service; import org.aspectj.lang.ProceedingJoinPoint; public class MyInterceptor { public void anyMethod() { } public void doAccessCheck() { System.out.println("前置通知" ); } public void doAfterReturning() { System.out.println("后置通知"); } public void doAfter() { System.out.println("最终通知"); } public void doAfterThrowing() { System.out.println("例外通知"); } public Object doBasicProfiling(ProceedingJoinPoint pjp) throws Throwable{ //if(){//判断用户是否有权限 System.out.println("进入方法"); Object result = pjp.proceed(); System.out.println("退出方法"); //} return result; } }
aop表达式的使用方法
<aop:pointcut id="point" expression="execution (!void blog.service.impl.PersonServiceBean.*(..))"/>
!void blog.service.impl.PersonServiceBean.*(..)中!void 表示拦截所有返回类型为非void类型的方法
java.lang.String表示拦截所有返回类型为String类型的方法
* blog.service.impl.PersonServiceBean.*(java.lang.String,..)表示拦截所有第一个参数为String类型的方法
相关文章推荐
- spring aop的使用(注解方式以及基于xml配置方式)
- spring aop的使用(注解方式以及基于xml配置方式)
- 8 -- 深入使用Spring -- 4...6 AOP代理:基于注解的XML配置文件的管理方式
- 8 -- 深入使用Spring -- 4...5 AOP代理:基于注解的“零配置”方式
- spring之aop编程——基于注解、xml配置文件方式
- Spring AOP基于注解的“零配置”方式实现以及一些其他知识点
- 基于spring注解方式配置和使用spring AOP
- Spring 3.0.5 MVC 基于注解ehcache.xml 配置方式
- Spring学习一:IOC(控制反转)和AOP(面向切面)的xml配置和注解方式
- 基于注解的Spring AOP的配置和使用--转载
- 实例说明Spring实现AOP的2种方式(注解和XML配置)
- 使用Spring的配置xml方式实现AOP
- Spring中基于配置XML与Annotation注解配置AOP
- 基于注解的Spring AOP的配置和使用
- 基于注解的Spring AOP的配置和使用--转载
- 重温Spring之旅6——基于XML配置方式进行AOP开发
- Spring 基于XML配置 基于注解配置 基于JAVA类配置比较以及适用场景
- Spring实现AOP方式之二:使用注解配置 Spring AOP
- (9) 使用Spring的注解方式实现AOP入门 以及 细节
- 基于注解的Spring AOP的配置和使用--转载