Java 调用svnkit实现svn功能
2012-05-09 21:48
309 查看
· 显示版本树
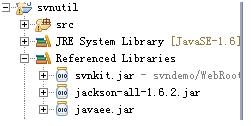
把svnkit.jar添加到项目中,用于实现svn功能。 把jackson-all-1.6.2.jar添加到项目中,用于显示树列表。把javaee.ar添加到项目中。
新建一个类(SVNUtil.class)实现svn功能
private String svnRoot;
private String userName;
private String password;
private SVNRepository repository;
/***
* 构造方法
* @param svnRoot
* svn根目录
*/
public SVNUtil(String svnRoot) {
this.svnRoot=svnRoot;
}
/***
* 构造方法
* @param svnRoot
* svn根目录
* @param userName
* 登录用户名
* @param password
* 登录密码
*/
public SVNUtil(String svnRoot, String userName, String password) {
this.svnRoot=svnRoot;
this.userName=userName;
this.password=password;
}
/***
* 通过不同的协议初始化版本库
*/
private static void setupLibrary()
{
// 对于使用http://和https://
DAVRepositoryFactory.setup();
//对于使用svn:/
/和svn+xxx:/ /
SVNRepositoryFactoryImpl.setup();
//对于使用file://
FSRepositoryFactory.setup();
}
每次连接库都进行登陆验证
/***
* 登录验证
* @return
*/
public boolean login(){
setupLibrary();
try{
//创建库连接
repository=SVNRepositoryFactory.create(SVNURL.parseURIEncoded(this.svnRoot));
//身份验证
ISVNAuthenticationManager authManager = SVNWCUtil
.createDefaultAuthenticationManager(this.userName,
this.password);
//创建身份验证管理器
repository.setAuthenticationManager(authManager);
return true;
} catch(SVNException svne){
svne.printStackTrace();
return false;
}
}
下面的方法实现查询给定路径下的条目列表功能
/***
*
* @param path
* @return 查询给定路径下的条目列表
* @throws SVNException
*/
@SuppressWarnings("rawtypes")
public List<SVN> listEntries(String path)
throws SVNException {
Collection entries = repository.getDir(path, -1, null,
(Collection) null);
Iterator iterator = entries.iterator();
List<SVN> svns = new ArrayList<SVN>();
while (iterator.hasNext()) {
SVNDirEntry entry = (SVNDirEntry) iterator.next();
SVN svn = new SVN();
svn.setCommitMessage(entry.getCommitMessage());
svn.setDate(entry.getDate());
svn.setKind(entry.getKind().toString());
svn.setName(entry.getName());
svn.setRepositoryRoot(entry.getRepositoryRoot().toString());
svn.setRevision(entry.getRevision());
svn.setSize(entry.getSize()/1024);
svn.setUrl(path.equals("") ? "/"+entry.getName() : path
+"/"+entry.getName());
svn.setAuthor(entry.getAuthor());
svn.setState(svn.getKind() == "file"?null:"closed");
svns.add(svn);
}
新建一个SVNServlet
添加一个方法用于把java对象转换为json字符串
/**
* 将java对象转换为json字符串
*
* @param obj
* :可以为map,list,javaBean等
* @return json字符串
* @createTime 2010-11-23 下午07:42:58
*/
public static String object2Json(Object
obj) {
try {
StringWriter sw = new StringWriter();
JsonGenerator gen = new JsonFactory().createJsonGenerator(sw);
mapper.writeValue(gen, obj);
gen.close();
return sw.toString();
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
protected void doGet(HttpServletRequest
request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
this.doPost(request, response);
}
protected void doPost(HttpServletRequest
request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
Object svns = null;
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String path = request.getParameter("pid");
String url = "svn://localhost/svndemo1";
String usrName = "usrName";
String password = "password";
if (path == null) {
path = "";
}
path = new String(path.getBytes("ISO-8859-1"),"UTF-8");
String type = request.getParameter("type");
PrintWriter out = response.getWriter();
try {
SVNUtil svnUtil = new SVNUtil(url, usrName, password);
if (svnUtil.login()) {
/*用于查询历史记录
if ("history".equals(type)) {
List<SVN> svnl = svnUtil.getHistory(path);
HashMap<String, Object> sv = new HashMap<String, Object>();
sv.put("total", svnl.size());
sv.put("rows", svnl);
svns = sv;
} else {*/
svns = svnUtil.listEntries(path);
//}
//把java对象转换成json字符串
String json = SVNServlet.object2Json(svns);
out.print(json);
} else {
System.out.println("验证失败");
}
} catch (SVNException ex) {
ex.printStackTrace();
}
out.flush();
out.close();
}
新建一个index.jsp用户显示版本数列表,页面显示我使用了jquery-easyui模板
<%@ page language="java" contentType="text/html;
charset=utf-8"
pageEncoding="utf-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN""http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<base href="<%=basePath%>">
<title>SVN</title>
<meta http-equiv="Content-Type" content="text/html;
charset=utf-8">
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This
is my page">
<link rel="stylesheet" type="text/css"
href="plugs/themes/default/easyui.css">
<link rel="stylesheet" type="text/css" href="plugs/themes/icon.css">
<script type="text/javascript" src="plugs/jquery-1.4.2.min.js"></script>
<script type="text/javascript" src="plugs/jquery.easyui.min.js"></script>
<script type="text/javascript">
$(function() {
$('#test').treegrid({
title : 'SVN列表',
nowrap : false,
rownumbers : true,
collapsible : false,
url : 'svn?pid=',
idField : 'url',
treeField : 'url',
frozenColumns : [ [ {
title : '路径',
field : 'url',
width : 350,
formatter : function(value) {
return '<span style="color:red">' +
decodeURI(value.substr(value.lastIndexOf("/"))) + '</span>';
}
} ] ],
columns : [ [ {
field : 'name',
title : '名称',
width : 120
}, {
field : 'size',
title : '文件大小(KB)',
width : 80,
rowspan : 2
}, {
field : 'revision',
title : '版本号',
width : 80,
rowspan : 2
}, {
field : 'author',
title : '作者',
width : 100,
rowspan : 2
}, {
field : 'date',
title : '修改日期',
width : 130,
rowspan : 2
}, {
field : 'commitMessage',
title : '注释',
width : 150,
rowspan : 2
}, {
field : 'kind',
title : '操作',
width : 120,
align : 'center',
rowspan : 2,
formatter : function(value) {
return value=='file' ? '<a
onclick="download()" style="cursor: pointer;color:red">下载</a><a onclick="viewHistory()" style="margin-left:5px; cursor: pointer;color:red">历史版本</a>' : '';
}
}] ],
onBeforeExpand : function(row, param) {
$(this).treegrid('options').url = 'svn?pid='+encodeURI(decodeURI(row.url));
}
});
});
function download(){
setTimeout(function(){
var node = $('#test').treegrid('getSelected');
if(node !=null)
window.open("download?url="+encodeURI(decodeURI(node.url))+"&size="+node.size+"&name="+encodeURI(decodeURI(node.name))+"&revision="+node.revision);
},200);
}
function viewHistory(){
setTimeout(function(){
var node = $('#test').treegrid('getSelected');
if(node != null) {
window.open("history.jsp?uri="+encodeURI(decodeURI(node.url)),"_blank","height=400,width=700,status=yes,toolbar=no,menubar=no,location=no");
}
}, 200);
}
</script>
</head>
<body>
<table id="test"></table>
</body>
</html>
显示效果如下
版本树列表

历史版本
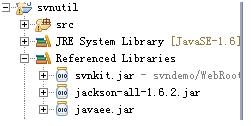
把svnkit.jar添加到项目中,用于实现svn功能。 把jackson-all-1.6.2.jar添加到项目中,用于显示树列表。把javaee.ar添加到项目中。
新建一个类(SVNUtil.class)实现svn功能
private String svnRoot;
private String userName;
private String password;
private SVNRepository repository;
/***
* 构造方法
* @param svnRoot
* svn根目录
*/
public SVNUtil(String svnRoot) {
this.svnRoot=svnRoot;
}
/***
* 构造方法
* @param svnRoot
* svn根目录
* @param userName
* 登录用户名
* @param password
* 登录密码
*/
public SVNUtil(String svnRoot, String userName, String password) {
this.svnRoot=svnRoot;
this.userName=userName;
this.password=password;
}
/***
* 通过不同的协议初始化版本库
*/
private static void setupLibrary()
{
// 对于使用http://和https://
DAVRepositoryFactory.setup();
//对于使用svn:/
/和svn+xxx:/ /
SVNRepositoryFactoryImpl.setup();
//对于使用file://
FSRepositoryFactory.setup();
}
每次连接库都进行登陆验证
/***
* 登录验证
* @return
*/
public boolean login(){
setupLibrary();
try{
//创建库连接
repository=SVNRepositoryFactory.create(SVNURL.parseURIEncoded(this.svnRoot));
//身份验证
ISVNAuthenticationManager authManager = SVNWCUtil
.createDefaultAuthenticationManager(this.userName,
this.password);
//创建身份验证管理器
repository.setAuthenticationManager(authManager);
return true;
} catch(SVNException svne){
svne.printStackTrace();
return false;
}
}
下面的方法实现查询给定路径下的条目列表功能
/***
*
* @param path
* @return 查询给定路径下的条目列表
* @throws SVNException
*/
@SuppressWarnings("rawtypes")
public List<SVN> listEntries(String path)
throws SVNException {
Collection entries = repository.getDir(path, -1, null,
(Collection) null);
Iterator iterator = entries.iterator();
List<SVN> svns = new ArrayList<SVN>();
while (iterator.hasNext()) {
SVNDirEntry entry = (SVNDirEntry) iterator.next();
SVN svn = new SVN();
svn.setCommitMessage(entry.getCommitMessage());
svn.setDate(entry.getDate());
svn.setKind(entry.getKind().toString());
svn.setName(entry.getName());
svn.setRepositoryRoot(entry.getRepositoryRoot().toString());
svn.setRevision(entry.getRevision());
svn.setSize(entry.getSize()/1024);
svn.setUrl(path.equals("") ? "/"+entry.getName() : path
+"/"+entry.getName());
svn.setAuthor(entry.getAuthor());
svn.setState(svn.getKind() == "file"?null:"closed");
svns.add(svn);
}
新建一个SVNServlet
添加一个方法用于把java对象转换为json字符串
/**
* 将java对象转换为json字符串
*
* @param obj
* :可以为map,list,javaBean等
* @return json字符串
* @createTime 2010-11-23 下午07:42:58
*/
public static String object2Json(Object
obj) {
try {
StringWriter sw = new StringWriter();
JsonGenerator gen = new JsonFactory().createJsonGenerator(sw);
mapper.writeValue(gen, obj);
gen.close();
return sw.toString();
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
protected void doGet(HttpServletRequest
request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
this.doPost(request, response);
}
protected void doPost(HttpServletRequest
request,
HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
Object svns = null;
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
String path = request.getParameter("pid");
String url = "svn://localhost/svndemo1";
String usrName = "usrName";
String password = "password";
if (path == null) {
path = "";
}
path = new String(path.getBytes("ISO-8859-1"),"UTF-8");
String type = request.getParameter("type");
PrintWriter out = response.getWriter();
try {
SVNUtil svnUtil = new SVNUtil(url, usrName, password);
if (svnUtil.login()) {
/*用于查询历史记录
if ("history".equals(type)) {
List<SVN> svnl = svnUtil.getHistory(path);
HashMap<String, Object> sv = new HashMap<String, Object>();
sv.put("total", svnl.size());
sv.put("rows", svnl);
svns = sv;
} else {*/
svns = svnUtil.listEntries(path);
//}
//把java对象转换成json字符串
String json = SVNServlet.object2Json(svns);
out.print(json);
} else {
System.out.println("验证失败");
}
} catch (SVNException ex) {
ex.printStackTrace();
}
out.flush();
out.close();
}
新建一个index.jsp用户显示版本数列表,页面显示我使用了jquery-easyui模板
<%@ page language="java" contentType="text/html;
charset=utf-8"
pageEncoding="utf-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme() + "://"
+ request.getServerName() + ":" + request.getServerPort()
+ path + "/";
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN""http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<base href="<%=basePath%>">
<title>SVN</title>
<meta http-equiv="Content-Type" content="text/html;
charset=utf-8">
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This
is my page">
<link rel="stylesheet" type="text/css"
href="plugs/themes/default/easyui.css">
<link rel="stylesheet" type="text/css" href="plugs/themes/icon.css">
<script type="text/javascript" src="plugs/jquery-1.4.2.min.js"></script>
<script type="text/javascript" src="plugs/jquery.easyui.min.js"></script>
<script type="text/javascript">
$(function() {
$('#test').treegrid({
title : 'SVN列表',
nowrap : false,
rownumbers : true,
collapsible : false,
url : 'svn?pid=',
idField : 'url',
treeField : 'url',
frozenColumns : [ [ {
title : '路径',
field : 'url',
width : 350,
formatter : function(value) {
return '<span style="color:red">' +
decodeURI(value.substr(value.lastIndexOf("/"))) + '</span>';
}
} ] ],
columns : [ [ {
field : 'name',
title : '名称',
width : 120
}, {
field : 'size',
title : '文件大小(KB)',
width : 80,
rowspan : 2
}, {
field : 'revision',
title : '版本号',
width : 80,
rowspan : 2
}, {
field : 'author',
title : '作者',
width : 100,
rowspan : 2
}, {
field : 'date',
title : '修改日期',
width : 130,
rowspan : 2
}, {
field : 'commitMessage',
title : '注释',
width : 150,
rowspan : 2
}, {
field : 'kind',
title : '操作',
width : 120,
align : 'center',
rowspan : 2,
formatter : function(value) {
return value=='file' ? '<a
onclick="download()" style="cursor: pointer;color:red">下载</a><a onclick="viewHistory()" style="margin-left:5px; cursor: pointer;color:red">历史版本</a>' : '';
}
}] ],
onBeforeExpand : function(row, param) {
$(this).treegrid('options').url = 'svn?pid='+encodeURI(decodeURI(row.url));
}
});
});
function download(){
setTimeout(function(){
var node = $('#test').treegrid('getSelected');
if(node !=null)
window.open("download?url="+encodeURI(decodeURI(node.url))+"&size="+node.size+"&name="+encodeURI(decodeURI(node.name))+"&revision="+node.revision);
},200);
}
function viewHistory(){
setTimeout(function(){
var node = $('#test').treegrid('getSelected');
if(node != null) {
window.open("history.jsp?uri="+encodeURI(decodeURI(node.url)),"_blank","height=400,width=700,status=yes,toolbar=no,menubar=no,location=no");
}
}, 200);
}
</script>
</head>
<body>
<table id="test"></table>
</body>
</html>
显示效果如下
版本树列表

历史版本
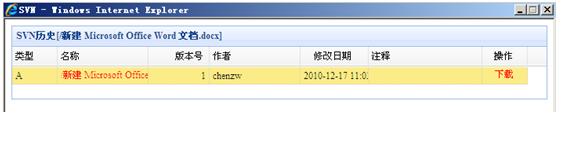
相关文章推荐
- Java 调用svnkit实现svn功能
- Java 调用svnkit实现svn功能
- Java 调用svnkit实现svn功能
- java调用svnkit实现svn功能
- Java 调用svnkit实现svn功能
- JAVA调用svnkit实现checkout
- ndk 实现 登录的功能(java 和 C 的调用)
- Java调用svnkit,检出指定版本的文件
- Java调用WebService接口实现发送手机短信验证码功能,java 手机验证码,WebService接口调用
- 通过java调用SVN API 实现代码的checkout update commit
- java调用svnkit工具类上传本地文件到svn服务器
- 1---------java调用NLPIR(ICTCLAS2016)实现分词功能
- Cocos2d-x3.3RC0通过JNI调用Android的Java层代码,实现分享功能
- java实现svn,svnkit框架的简单应用
- java调用svnkit连接svn出现Exception in thread "main"怎么解决
- java通过jna调用科大讯飞语音云实现语音识别功能
- 使用svnkit api,纯java操作svn,实现svn提交,更新等操作(修正版)
- Java 实现在线翻译功能 调用微软Bing API
- JAVA调用ImageCapOnWeb控件实现拍照功能
- Chap5:使用JNI技术实现java程序调用第三方dll(c/c++)文件的功能