Struts2文件上传下载
2010-09-15 02:14
387 查看
先看下文件结构
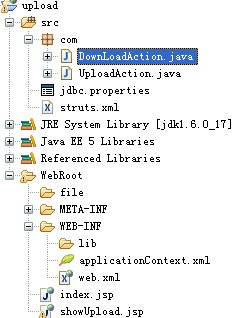
下面是 源码及其相关注释
web.xml
index.jsp
showUpload.jsp
看了上面的就可以做一个上传下载的了,这里只是简单贴下代码
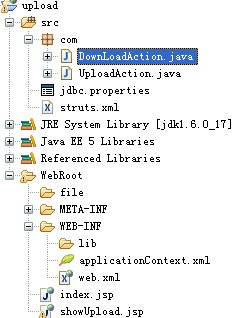
下面是 源码及其相关注释
package com; import java.io.File; import java.io.InputStream; import java.io.UnsupportedEncodingException; import org.apache.struts2.ServletActionContext; import com.opensymphony.xwork2.ActionSupport; public class DownLoadAction extends ActionSupport { private static final long serialVersionUID = 1L; private String savePath = "file//";//文件上传的路径 private String fileFileName; private File file; private String fileContentType; public String getFileName() { return fileFileName; } public void setFileName(String fileName) { this.fileFileName = fileName; } public File getFile() { return file; } public void setFile(File file) { this.file = file; } public String getFileContentType() { return fileContentType; } public void setFileContentType(String fileContentType) { this.fileContentType = fileContentType; } public String getFileFileName() { return fileFileName; } public void setFileFileName(String fileFileName) { this.fileFileName = fileFileName; } public String getSavePath() { return savePath; } public void setSavePath(String savePath) { this.savePath = savePath; } @Override public String execute() throws Exception { fileFileName = ServletActionContext.getRequest().getParameter( "fileName"); return SUCCESS; } // 从下载文件原始存放路径读取得到文件输出流 public InputStream getDownloadFile() throws UnsupportedEncodingException { fileFileName = new String(fileFileName.getBytes("ISO8859-1"), "UTF-8"); System.out.println("fileFileName:Down:"+fileFileName); return ServletActionContext.getServletContext().getResourceAsStream( savePath + fileFileName); } // 如果下载文件名为中文,进行字符编码转换 public String getDownloadChineseFileName() { String downloadChineseFileName = fileFileName; try { downloadChineseFileName = new String(downloadChineseFileName .getBytes(), "ISO8859-1"); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return downloadChineseFileName; } }
package com; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.InputStream; import java.io.OutputStream; import org.apache.struts2.ServletActionContext; import com.opensymphony.xwork2.ActionSupport; public class UploadAction extends ActionSupport { private static final long serialVersionUID = 1L; private File[] uploadFile;//上传的文件名 private String[] contentType; private String[] caption; private String[] uploadFilename; public String[] getCaption() { return caption; } public void setCaption(String[] caption) { this.caption = caption; } public String[] getContentType() { return contentType; } public void setUploadFileContentType(String[] contentType) { this.contentType = contentType; } public File[] getUploadFile() { return uploadFile; } public void setUploadFile(File[] uploadFile) { this.uploadFile = uploadFile; } public String[] getUploadFilename() { return uploadFilename; } public void setUploadFileFileName(String[] uploadFilename) { this.uploadFilename = uploadFilename; } /** * 拷贝文件到相应的目录下 * @param src * @param dst */ private static void copy(File src, File dst) { try { InputStream in = null; OutputStream out = null; try { in = new BufferedInputStream(new FileInputStream(src)); out = new BufferedOutputStream(new FileOutputStream(dst)); byte[] buffer = new byte[16 * 1024]; while (in.read(buffer) > 0) { out.write(buffer); } } finally { if (null != in) { in.close(); } if (null != out) { out.close(); } } } catch (Exception e) { e.printStackTrace(); } } @Override public String execute() { for (int i = 0; i < this.getUploadFile().length; i++) { File imageFile = null; imageFile = new File(ServletActionContext.getServletContext() .getRealPath("/") + "file/" + new String(uploadFilename[i])); copy(this.getUploadFile()[i], imageFile); } return SUCCESS; } }
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <!-- developer mode convenient for Debug --> <constant name="struts.i18n.encoding" value="GBK" /> <constant name="struts.ui.theme" value="simple" /> <constant name="struts.ui.templateDir" value="template" /> <constant name="struts.ui.templateSuffix" value="ftl" /> <package name="uploadFile" extends="struts-default"> <action name="myUpload" class="com.UploadAction"> <interceptor-ref name="fileUpload"> <param name="savePath">/file</param> <param name="maximumSize">1024000</param> </interceptor-ref> <interceptor-ref name="defaultStack" /> <result name="input">/index.jsp</result> <result name="success">/showUpload.jsp</result> </action> <action name="download" class="com.DownLoadAction"> <!-- 设置文件名参数,由页面上传入 --> <param name="fileName"></param> <result name="success" type="stream"> <!-- 下载文件类型定义 --> <param name="contentType"> application/octet-stream </param> <!-- 下载文件处理方法 --> <param name="contentDisposition"> attachment;filename="${downloadChineseFileName}" </param> <!-- 下载文件输出流定义 --> <param name="inputName">downloadFile</param> <param name="bufferSize">4096</param> </result> </action> </package> </struts>
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"> <filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter </filter-class> </filter> <filter> <filter-name>struts-cleanup</filter-name> <filter-class> org.apache.struts2.dispatcher.ActionContextCleanUp </filter-class> </filter> <filter-mapping> <filter-name>struts-cleanup</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <!--添加一个监听器,告诉webapp启动时,就初始化化Spring容器,这要才Spring才可以管理Action 这样Struts可以向Spring索要Action的bean 这个ContextLoaderListener类,初始化时就会初始化Spring容器,加载xml的配置文件,并初始化bean 注意ContextLoaderListener默认会加载/WEB-INF/applicationContext-*.xml这个配置文件,如果你的配置文件不在这个位置 那么你需要重新指定Spring的配置。使用全局参数contextConfigLocation来指定配置文件的位置,当然可以指定多个位置的配置路径,格式如下: <context-param> <param-name>contextConfigLocation</param-name> <param-value>classpath*:beans.xml</param-value> </context-param> --> <listener> <listener-class> org.springframework.web.context.ContextLoaderListener </listener-class> </listener> <context-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/applicationContext*.xml</param-value> </context-param> <filter> <filter-name>encodingFilter</filter-name> <filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class> <init-param> <param-name>encoding</param-name> <param-value>UTF-8</param-value> </init-param> </filter> <filter-mapping> <filter-name>encodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
index.jsp
<%@ page language="java" contentType="text/html;charset=gb2312"%> <%@ taglib prefix="s" uri="/struts-tags"%> <! DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd" > <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>upload file</title> <mce:script type="text/javascript"><!-- function addMore() { var td = document.getElementById("more"); var div = document.createElement("div"); var ss = document.createElement("span"); var input = document.createElement("input"); var span = document.createElement("span"); var text = document.createElement("input"); var button = document.createElement("input"); div.style.marginTop = "4"; ss.innerHTML = "选择文件:"; input.type = "file"; input.name = "uploadFile"; span.innerHTML = "文件资源描述:"; text.type = "text"; text.size = 35; text.name = "caption"; button.type = "button"; button.value = "移 除"; button.onclick = function() { td.removeChild(div); div = null; fileInputNumber--; } div.appendChild(ss); div.appendChild(input); div.appendChild(span); div.appendChild(text); div.appendChild(button); td.appendChild(div); fileInputNumber++; } // --></mce:script> </head> <body> <s:fielderror /> <s:form method="post" name="uploadForm" action="myUpload.action" enctype="multipart/form-data" onsubmit="return check();" theme="simple"> <tr> <td id="more">选择文件:<input type="file" name="uploadFile" />文件资源描述:<input type="text" name="caption" size="35" /><input type="button" value="添 加" onclick="addMore()" /></td> </tr> <tr> <td align="center"><s:submit value="上传" /> <s:reset value="重置" /></td> </tr> </s:form> </body> </html>
showUpload.jsp
<%@ page language="java" contentType="text/html;charset=UTF-8"%> <%@ taglib prefix="s" uri="/struts-tags"%> <! DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd" > <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>查看上传文件</title> </head> <body> <center> <table border="1"> <s:iterator value="uploadFilename" status="stat"> <tr> <td> <s:property value="%{uploadFilename[#stat.index]}" /> <br /> </td> <a href="download.action?fileName=<s:property value=" mce_href="download.action?fileName=<s:property value="%{uploadFilename[#stat.index]}" ></a>">下载</a> </tr> <tr> <td> <s:property value="%{caption[#stat.index]}" /> </td> </tr> </s:iterator> </table> </center> </body> </html>
看了上面的就可以做一个上传下载的了,这里只是简单贴下代码
相关文章推荐
- Struts2的文件上传与下载
- Struts2 文件上传与下载
- Struts2之实现文件上传与下载
- Struts2 控制文件上传下载
- struts2文件的上传和下载
- struts2 文件上传与下载原理
- struts2的文件的上传与下载笔记
- Struts2 Chapter 7&8 :文件的上传和下载,图形报表的生成part1
- Struts2使用注解实现文件的上传与下载(二)
- JavaEE中struts2实现文件上传下载功能实例解析
- struts2实现文件上传与下载功能
- struts2文件上传下载
- struts2文件上传和下载
- Struts2文件上传下载
- Struts2使用注解实现文件的上传与下载(二)
- Struts2--文件上传与下载
- struts2进行文件上传下载时的格式设置(contentType类型)
- 11、Struts2 的文件上传和下载
- struts2教程--实现文件上传下载
- Struts2中文件上传下载实例