《Delphi 算法与数据结构》学习与感悟[1]: 通过 "顺序查找" 与 "二分查找" 说明算法的重要性
2008-03-17 10:41
691 查看
测试效果图:
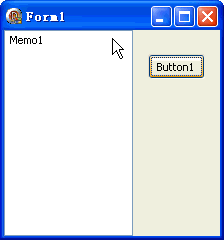
二分查找太快了, 用 GetTickCount 测试不出来, 只好使用 QueryPerformanceCounter;
另外 TStringList.Find 方法也是使用了 "二分查找" 的办法.
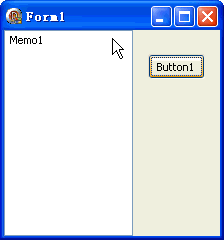
unit Unit1; interface uses Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms, Dialogs, StdCtrls; type TForm1 = class(TForm) Button1: TButton; Memo1: TMemo; procedure Button1Click(Sender: TObject); end; var Form1: TForm1; implementation {$R *.dfm} {顺序查找函数} function SeqSearch(List: TStringList; const str: string): Integer; var i: Integer; begin for i := 0 to List.Count - 1 do if CompareText(List[i], str) = 0 then begin Result := i; Exit; end; Result := -1; end; {二分查找函数; 二分查找只能针对有序列表} function BinarySearch(List: TStringList; const str: string): Integer; var L,R,M: Integer; CompareResult: Integer; begin Result := -1; L := 0; R := List.Count - 1; while L <= R do begin M := (L + R) div 2; CompareResult := CompareText(List[M], str); if CompareResult < 0 then L := M + 1 else if CompareResult > 0 then R := M - 1 else begin Result := M; Exit; end; end; end; {对比测试} procedure TForm1.Button1Click(Sender: TObject); var TestList: TStringList; i: Integer; n1,n2: Int64; Count1,Count2: Integer; s: string; const num = 1000000; {准备测试百万个数据} begin TestList := TStringList.Create; for i := 0 to num-1 do TestList.Add(IntToHex(i,8)); {准备有序的测试值列表} Memo1.Clear; Count1 := 0; Count2 := 0; {搞 10 实验} for i := 0 to 9 do begin {产生范围内的随机字串} Randomize; s := IntToHex(Random(num),8); {顺序查找} QueryPerformanceCounter(n1); SeqSearch(TestList, s); QueryPerformanceCounter(n2); Memo1.Lines.Add(IntToStr(n2-n1)+ #9); Count1 := Count1 + (n2-n1); {二分查找} QueryPerformanceCounter(n1); BinarySearch(TestList, s); QueryPerformanceCounter(n2); Memo1.Lines[i] := Memo1.Lines[i] + IntToStr(n2-n1); Count2 := Count2 + (n2-n1); end; Memo1.Lines.Add('----------------'); Memo1.Lines.Add('平均值:'); Memo1.Lines.Add(IntToStr(Count1 div 10)+ #9 + IntToStr(Count2 div 10)); Memo1.Lines.Add('----------------'); Memo1.Lines.Insert(0, '顺序'#9'二分'); TestList.Free; end; end.
二分查找太快了, 用 GetTickCount 测试不出来, 只好使用 QueryPerformanceCounter;
另外 TStringList.Find 方法也是使用了 "二分查找" 的办法.
相关文章推荐
- 《Delphi 算法与数据结构》学习与感悟[3]: 获取一个字节中非空位的个数
- 《Delphi 算法与数据结构》学习与感悟[8]: 单向链表的添加、删除与遍历
- 《Delphi 算法与数据结构》学习与感悟[5]: 定位一个字符位置时, Pos 函数为什么不是最快的?
- 《Delphi 算法与数据结构》学习与感悟[4]: 关于 const
- 《Delphi 算法与数据结构》学习与感悟[4]: 关于 const
- 《Delphi 算法与数据结构》学习与感悟[9]: 循环链表
- 《Delphi 算法与数据结构》学习与感悟[10]: 双向链表
- 《Delphi 算法与数据结构》学习与感悟[2]: 数据对齐
- 《Delphi 算法与数据结构》学习与感悟[6]: 一个简单的"单向链表"
- 《Delphi 算法与数据结构》学习与感悟[7]: 链表与数组的异同
- 《Delphi 算法与数据结构》学习与感悟[4]: 关于 const
- 算法学习之查找(顺序、二分法、排序二叉树以及 Hash 表)
- 一步一步复习数据结构和算法基础-索引顺序表查找
- 神器 VisuAlgo:通过动画学习算法和数据结构
- 算法如功夫——二分查找和顺序查找的C代码
- 算法与数据结构--在顺序线性表L中查找第1个值与e满足compare()的元素的为序--算法2.5
- 利用VisuAlgo通过动画学习算法和数据结构
- PHP数据结构——二分查找与顺序查找
- 算法学习之查找算法:静态查找表(1)顺序表查找
- 数据结构 c语言 顺序查找算法(linux下实现)